Enhance Your File System Workflows: New Functionalities in Python Pathlib Module
- The Tech Platform
- Mar 15, 2024
- 7 min read
The pathlib module, introduced in Python 3.4, has become a cornerstone for working with file systems in Python. It offers a robust and cross-platform way to handle file paths. Unlike, using raw strings, pathlib provides a class-based approach with objects representing file system paths. These objects come equipped with a rich set of methods for manipulating and interacting with paths, making common file system tasks more streamlined and efficient.
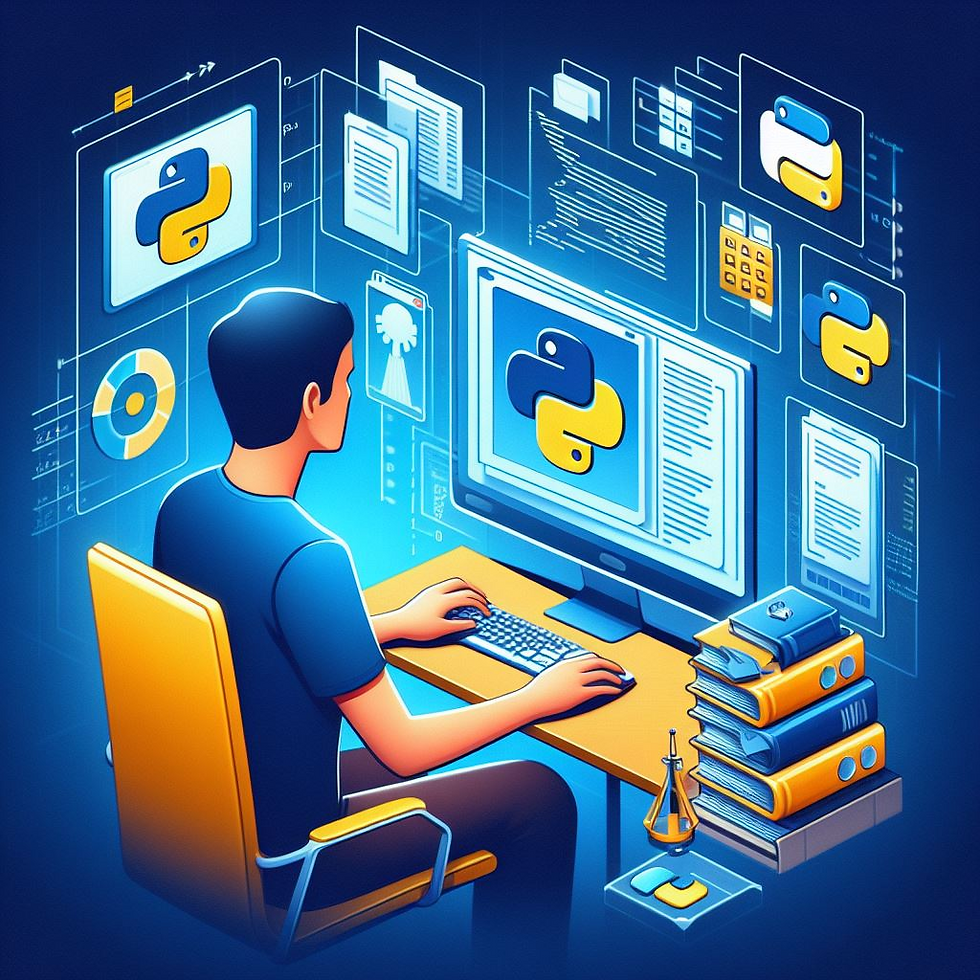
Recently, pathlib has seen exciting new functionalities added to enhance its capabilities. These features provide more control over path matching, improve directory tree navigation, and offer platform-independent ways to check for special file system objects. Let's delve into these advancements and explore how they elevate pathlib as a powerful tool for file system operations in Python.
New Functionalities and Methods in the Pathlib module:
The pathlib module in Python has recently gained four new functionalities:
pathlib.Path.walk(): This method simplifies directory tree traversal by yielding information about each directory and its contents.
walk_up parameter: Added to pathlib.PurePath.relative_to(), allows for including .. (parent directory) entries in the generated relative path.
pathlib.Path.is_junction(): This method provides a platform-independent way to check if a path represents a junction point (a special file system object).
Case-sensitive matching: The addition of the case_sensitive parameter to pathlib.Path.glob(), pathlib.Path.rglob(), and pathlib.PurePath.match() allows for more precise control over file system searches based on letter case.
Let's explore each functionality in detail.
1. Directory Tree Navigation
Traversing directory trees, also known as walking directories, is a fundamental task in Python for various file system operations. It involves iterating through a directory, accessing its subdirectories, and potentially processing the files within those subdirectories. Traditionally, this has been accomplished using the os.walk() function from the OS module. However, the pathlib module offers a more efficient approach ie., the new pathlib.Path.walk() method.
Traversing with os.walk()
The os.walk() function takes a directory path as input and returns a generator that yields a tuple for each directory in the tree. This tuple contains three elements:
root: The absolute path of the current directory being processed.
dirs: A list of subdirectory names within the current directory.
files: A list of filenames within the current directory.
Here's how you would use os.walk() to iterate through a directory tree:
import os
# Directory path to walk
root_dir = "/home/user/project"
for root, dirs, files in os.walk(root_dir):
# Process the current directory
print(f"Current directory: {root}")
# Process subdirectories (optional)
# ...
# Process files
for filename in files:
print(f"File: {filename}")
While os.walk() is effective, it has some drawbacks:
Mixing Modules: It requires importing both os and pathlib if you're using pathlib for other file system operations.
String Paths: It works with string paths, which can be less convenient than using Path objects.
Introducing pathlib.Path.walk()
pathlib.Path.walk() addresses these limitations by offering a specific way to traverse directory trees. This new method directly works with Path objects, providing a more integrated experience.
Here's its functionality:
Input: Takes a Path object representing the root directory of the tree.
Output: Yields a tuple for each directory encountered during the walk, similar to os.walk():
The first element is the Path object of the current directory.
The second element is a list of subdirectory names (also as Path objects).
The third element is a list of filenames within that directory (strings).
This approach offers several benefits:
Seamless Integration: It works directly with Path objects, promoting consistency and leveraging the existing functionalities of pathlib.
Concise Code: It simplifies directory traversal compared to manual loops and conditional statements used with os.walk().
Cleaner Syntax: The code becomes more readable and avoids the need for string path conversions.
Here's an example of using pathlib.Path.walk():
from pathlib import Path
# Directory path to walk
root_dir = Path("/home/user/project")
# Walk the directory tree
for current_dir, subdirs, files in root_dir.walk():
print(f"Current directory: {current_dir}")
# Process subdirectories and files as needed
# ...
In this example, pathlib.Path.walk() iterates through the directory tree starting from root_dir, yielding information about each directory and its contents using Path objects.
2. More Precise Relatives Path:
Before the introduction of the walk_up parameter, pathlib.PurePath.relative_to() had a limitation when generating relative paths.
pathlib.PurePath.relative_to():
This method calculates the relative path from a starting path (base path) to a target path.
It's crucial for tasks like creating relative links or referencing files within a project structure.
Previous Behavior (Without walk_up):
The core assumption was that the base and the target path resided under the same root directory.
Consequently, the generated relative path only included directory names within that shared root.
It wouldn't include any .. (parent directory) entries, even if navigating the directory tree was necessary to reach the target.
Why walk_up was Needed:
Inaccurate Paths for Upward Navigation: This behavior could lead to inaccurate relative paths when the target path is located outside the immediate subdirectory structure of the base path. It wouldn't include the necessary .. entries to navigate upwards.
Inconsistency with os.path.relpath(): The default behavior of pathlib.PurePath.relative_to() differed from the popular os.path.relpath() function in the os module, navigating upwards when generating relative paths. This inconsistency could confuse and require additional logic when working with both modules.
Less Intuitive Results: Omitting .. entries sometimes made the relative path less readable and harder to understand, especially for developers navigating a project structure that might involve moving up and down directories.
The Need for walk_up:
The walk_up parameter addresses these limitations by allowing you to explicitly control whether the relative path can include .. entries.
Setting walk_up=True enables pathlib.PurePath.relative_to() to consider navigating upwards in the directory tree if necessary, resulting in a more accurate and intuitive relative path.
Example:
from pathlib import Path
base_path = Path("/home/user/project")
file_path = Path("/home/user/project/data/file.txt")
# Relative path without walk_up (default)
relative_path = file_path.relative_to(base_path)
print(relative_path) # Output: data/file.txt (no '..' entries)
# Relative path with walk_up (including '..')
relative_path = file_path.relative_to(base_path, walk_up=True)
print(relative_path) # Output: ../data/file.txt (includes '..' to navigate up)
In this example, without walk_up, the relative path omits the .. because it assumes both paths share the same root (/home/user/project). With walk_up=True, the relative path accurately reflects the need to navigate up one level to reach the data directory from the project directory.
Benefits of walk_up:
Consistency with os.path.relpath(): Setting walk_up=True aligns the behavior of pathlib.PurePath.relative_to() with the os.path.relpath() function from the os module. This consistency can be helpful when working with both modules or migrating code.
Intuitive Relative Paths: Including .. entries often makes the relative path more readable and easier to understand, especially when navigating up the directory tree. It reflects the actual path you would take to reach the target from the starting point.
3. Junction Detection
The introduction of pathlib.Path.is_junction() brings a convenient way to check for junctions within your Python code.
Junctions (symbolic links or shortcuts on some platforms) are special file system objects that act as pointers to another location. They create an alias for an existing directory or file elsewhere in the file system.
Previous Method: os.path.isjunction():
Previously, checking for junctions in Python involved using the os.path.isjunction() function. However, the new pathlib.Path.is_junction() method offers a more streamlined and integrated approach within the pathlib ecosystem.
Code Example:
import os
# Assuming a path that might be a junction (replace with your actual path)
potential_junction = "your/potential/junction/path"
# Check if it's a junction (might have platform-specific behavior)
is_junction = os.path.isjunction(potential_junction)
if is_junction:
print(f"{potential_junction} is a junction.")
else:
print(f"{potential_junction} is not a junction.")
Drawbacks of using os.path.isjunction():
Platform-Specific: The implementation of os.path.isjunction() might differ across operating systems. This can lead to inconsistencies in your code if you need to handle junctions on different platforms.
Mixing Modules: You need to import both os and pathlib modules, potentially cluttering your code if you primarily use pathlib for file system operations.
Current Method: pathlib.Path.is_junction():
This new method determines if a Path object represents a junction point. It offers a pathlib-specific way to check for junctions, integrating seamlessly with the existing Path objects.
The new pathlib.Path.is_junction() method provides a convenient way to check for junctions (symbolic links or shortcuts) directly within pathlib workflows. Here's how to use it:
from pathlib import Path
# Assuming a path that might be a junction (replace with your actual path)
potential_junction = Path("your/potential/junction/path")
# Check if it's a junction
is_junction = potential_junction.is_junction()
if is_junction:
print(f"{potential_junction} is a junction.")
# Handle the junction as needed (e.g., follow the link)
else:
print(f"{potential_junction} is not a junction.")
Explanation:
We import Path from pathlib.
We define a potential_junction variable with a path that might be a junction. Replace this with the actual path you want to check.
We use the is_junction() method on the potential_junction object.
An if statement checks the result of is_junction().
If True, the path is a junction, and a message is printed. You can then follow the link or perform any necessary actions based on the junction.
If False, the path is not a junction, and another message is printed.
Benefits of using pathlib.path.is_junction():
Platform-Independent: Unlike the existing os.path.isjunction() function, which might have platform-specific implementations, pathlib.Path.is_junction() provides a consistent approach across different operating systems. This simplifies your code and avoids the need for platform-specific checks.
pathlib Integration: Using pathlib.Path.is_junction() leverages the familiar syntax and functionality of pathlib, making it easier to integrate junction detection into your existing code that uses Path objects.
By using pathlib.Path.is_junction(), you can efficiently detect junctions within your file system operations using pathlib. This streamlines your code and ensures consistent behavior across platforms.
4. Case-Sensitive Matching
The case_sensitive parameter performs case-specific searches within your file system operations. This offers more control and ensures you find the right files or directories based on their case.
The addition of the case_sensitive parameter to pathlib.Path.glob(), pathlib.Path.rglob(), and pathlib.PurePath.match() brings more control to file system operations in Python
Before this addition, the pathlib.Path.glob(), pathlib.Path.rglob(), and pathlib.PurePath.match() methods in Python performed case-insensitive matching by default. This means searching for files or directories with a specific pattern wouldn't differentiate between uppercase and lowercase letters.
The introduction of the case_sensitive parameter changes this behavior and brings more control to your file system operations:
Optional Parameter: You can now choose whether you want the search to be case-sensitive.
Default Behavior: If you don't specify the case_sensitive parameter, the search remains case-insensitive, with the previous default behavior.
Case-Sensitive Matching: Setting case_sensitive=True allows you to perform searches that are sensitive to the case of letters in filenames and directory names. This is particularly important on operating systems where filenames are case-sensitive (e.g., some Unix-like systems).
Benefits:
Fine-Grained Control: This parameter empowers you to tailor your searches based on the specific case requirements. You can be flexible with a case or ensure exact matches based on the desired case sensitivity.
Accurate Search Results: By explicitly setting case sensitivity, you can avoid unintended matches caused by case differences. This leads to more precise and reliable results when searching for files or directories using these pathlib methods.
Example:
Imagine you have a directory containing files named "textFile.txt", "TextFile.TXT", and "anotherFile.txt". Here's how the case_sensitive parameter affects matching:
from pathlib import Path
# Directory path
dir_path = Path("your/directory/path")
# Case-insensitive search (default behavior)
matches = dir_path.glob("*.txt") # Matches all three files
for match in matches:
print(match)
# Case-sensitive search (only matches exact case)
matches = dir_path.glob("*.txt", case_sensitive=True) # Matches only "textFile.txt"
for match in matches:
print(match)
In the first case, the default behavior is case-insensitive, so all three files are found. However, when you set case_sensitive=True, only "textFile.txt" is matched because it exactly matches the search pattern.
Conclusion
Pathlib gains significant power with these additions. They simplify directory navigation, provide more control over relative paths and matching, and offer a platform-independent way to detect junctions. These enhancements make pathlib an even more versatile and robust tool for file system operations in Python.
Comments