Python Format String: A Beginner's Guide
- The Tech Platform
- Jul 19, 2023
- 6 min read
Python's format string is a powerful feature that allows developers to format and manipulate output strings in a concise and efficient manner. Whether you're working on data visualization, generating reports, or simply printing formatted messages, understanding and utilizing Python format strings can significantly enhance your coding experience.
In this beginner's guide, we will explore the fundamentals of Python format string, provide examples, and different formatting options provided by Python along with their use case and applications.
Table of content:
2. Basic Syntax
7. Conclusion
What is Python Format String?
A format string is a string that contains placeholders, known as conversion specifiers, along with optional formatting instructions. These placeholders are replaced with values during runtime, allowing for dynamic and customizable output formatting. Python's format string syntax is based on the C programming language's printf-style formatting.
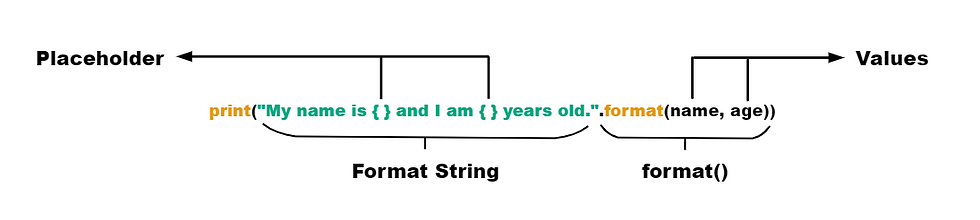
Basic Syntax
The general structure of a format string consists of a string literal containing placeholders, which are substituted with values during runtime using the format() method.
Let's take a closer look at the syntax:
formatted_string = "Text and {} placeholders".format(value1, value2, ...)
In this example,
The curly braces {} act as placeholders within the string.
The format(value1, value2, ....) method is then used to insert values into these placeholders. The values can be variables, literals, or even expressions, and they are mapped to the corresponding placeholders based on their order.
Examples of Format String Usage
Here we have some basic examples which will help you to understand how Python Format String work and how placeholders can be inserted and values are ordered.
1. Basic Placeholder Insertion:
Placeholder insertion is a process of replacing placeholders within a format string with corresponding values during runtime.
name = "John"
age = 25
print("My name is {} and I am {} years old.".format(name, age))
Output:
My name is John and I am 25 years old.
2. Specifying Value Order:
Specifying value order allows us to control the order in which values are inserted into placeholders within a format string by using index numbers.
By explicitly stating the index numbers within the curly braces {}, we can override the default order of value insertion based on their positions in the format() method arguments.
This is particularly useful when we want to rearrange the values or reference them in a specific order within the output string.
name = "Lisa"
age = 30
print("My name is {1} and I am {0} years old.".format(age, name))
Output:
My name is Lisa and I am 30 years old.
Formatting Options in Python Format Strings
Python format strings offer a variety of formatting options that allow you to customize the appearance of your output:
Field Width and Alignment
Numeric Formatting
String Truncation
1. Field Width and Alignment:
Field width refers to the width of the output field, i.e., the number of characters reserved for the formatted value. Alignment determines how the value will be aligned within the field. To specify field width and alignment, you can use format specifiers within the curly braces {}.
The general syntax is
{:[width][alignment]}
[width]: Specifies the desired width of the field. If the formatted value is shorter than the width, additional spaces will be added to reach the specified width.
[alignment]: Determines the alignment of the value within the field. There are three common alignment options:
<: Left-aligns the value within the field.
>: Right-aligns the value within the field.
^: Centers the value within the field.
value = "Hello"
print("'{:10}'".format(value))
print("'{:<10}'".format(value))
print("'{:>10}'".format(value))
Output:

2. Numeric Formatting:
Python format strings provide various options to format numeric values, such as integers and floating-point numbers. These options allow you to control padding, precision, and comma separation.
Padding: Padding allows you to specify the minimum width of the output, filling any remaining space with specified characters. This is useful for aligning columns of numeric values.
Precision: Precision refers to the number of digits after the decimal point for floating-point numbers. It allows you to control the level of detail in the output.
Comma Separation: Comma separation adds thousands of separators to large numbers for improved readability.
number = 43287
print("Default: {:d}".format(number))
print("Padded: {:08d}".format(number))
print("With commas: {:,}".format(number))
Output:

3. String Truncation:
String truncation allows you to limit the displayed length of a string to a desired number of characters. It can be useful when dealing with long strings or when you only need to display a portion of the text.
Truncation: Truncation is achieved by specifying the maximum number of characters you want to display within the format specifier.
Truncated strings will end with an ellipsis (...) if the original string exceeds the specified length.
message = "This is a very long message."
print("{:.10}".format(message))
Output:

Advanced Formatting Techniques
Beyond the basics, Python format strings offer advanced techniques for formatting specific data types.
1. Formatting Dates and Times:
When working with dates and times, it's often necessary to format them in a specific way. Python's datetime module provides functionalities to work with dates and times. By utilizing format specifiers, you can customize the output format of date and time values.
The %Y specifier represents the four-digit year.
The %m specifier represents the two-digit month.
The %d specifier represents the two-digit day.
The %H specifier represents the hour in 24-hour format.
The %M specifier represents the minute.
The %S specifier represents the second.
Example:
import datetime
current_datetime = datetime.datetime.now()
print("Current date and time: {:%Y-%m-%d %H:%M:%S}".format(current_datetime))
Output:

2. Formatting Currency and Percentages:
Formatting currency and percentages is often required when dealing with financial data or presenting statistical information. Python format strings provide format specifiers to control the display of currency symbols, decimal places, and percentage values.
The :.2f format specifier is used to format floating-point numbers as currency with two decimal places.
The :.2% format specifier is used to format decimal numbers as percentages with two decimal places.
Example:
price = 19.99
discount = 0.25
print("Price: ${:.2f}".format(price))
print("Discount: {:.2%}".format(discount))
Output:

In the first example, "{:.2f}" is used to format the price value as a floating-point number with two decimal places, preceded by the dollar sign. The :.2f format specifier indicates that the value should be formatted as a floating-point number with two decimal places.
In the second example, "{:.2%}" is used to format the discount value as a percentage with two decimal places. The :.2% format specifier indicates that the value should be formatted as a percentage with two decimal places.
3. Handling Precision and Rounding:
Python format strings provide options to handle precision and rounding when working with floating-point numbers. You can specify the desired number of decimal places to display or round the value to a specific precision.
The :.2f format specifier limits the number of decimal places to two, effectively rounding the value to the specified precision.
value = 3.14159265359
print("Value: {:.2f}".format(value))
Output:

In this example, "{:.2f}" is used to format the value as a floating-point number with two decimal places. The :.2f format specifier limits the number of decimal places to two, effectively rounding the value to the specified precision.
Use Cases and Applications
Formatting options in Python format strings can be used in various scenarios where you need to present data in a structured and visually appealing manner. Here are some common use cases:
Generating Formatted Reports and Tables:
Format strings are widely used in generating reports and tables with aligned columns, consistent spacing, and properly formatted data. By utilizing field width and alignment options, you can ensure that your output is neatly organized and easy to read.
Data Visualization with Formatted Labels:
When visualizing data, format strings are valuable for customizing labels and annotations. You can apply formatting options to numeric labels, such as adding commas for large numbers or specifying precision for decimal values, to enhance clarity and comprehension.
Logging and Error Message Formatting:
Format strings are useful for formatting log messages, error messages, and debugging output. You can utilize formatting options to structure the messages, include relevant data, and align the output for easier readability.
Data Export and File Generation:
When exporting data to files, format strings allow you to format the output in a specific manner. For example, when exporting data as CSV (Comma-Separated Values) or other delimited formats, you can use format strings to control the appearance of each field or column.
User Interface Display:
Format strings can be employed in user interfaces to present data to users in a formatted and user-friendly way. Whether it's displaying formatted timestamps, currency values, or progress indicators, format strings enable consistent and appealing data presentation.
Data Analysis and Reporting:
In data analysis and reporting tasks, format strings can be used to format statistical measures, percentages, and other numerical data. This ensures that the data is displayed appropriately and in a standardized format across different reports and visualizations.
Localization and Internationalization:
Format strings play a crucial role in localization and internationalization efforts. They allow for the adaptation of output formats to different languages, regions, and cultural conventions. By using format strings with localization libraries, you can dynamically format dates, times, numbers, and currencies according to specific locales.
Conclusion
Python format strings are features for output formatting in Python. By understanding their syntax, utilizing formatting options, and exploring advanced techniques, you can improve the readability and beauty of your code. This beginner's guide has provided you with the fundamental knowledge to use Python format strings effectively.
Comments