What is Function Declaration and Function Expression in JavaScript. How to use them.
- The Tech Platform
- Oct 13, 2021
- 4 min read
Updated: Jun 2, 2023
When working with JavaScript functions, you have two primary ways to define them: Function Declaration and Function Expression. While both methods allow you to create functions, they have distinct differences in their syntax, behavior, and scope.
Understanding the distinctions between Function Declarations and Function Expressions is crucial for writing clean and maintainable JavaScript code. In this article, we will explore the characteristics of each approach and discuss when and how to use them effectively.
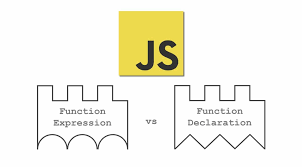
Function Declaration
A Function Declaration, also known as a Function Statement, allows you to define a function with specified parameters without the need for a variable assignment. It stands on its own as a separate construct and cannot be nested within a non-function block. To declare a function, you use the function keyword followed by the function name and parameter list, enclosed in parentheses. The function body is enclosed in curly braces.
Syntax:
function tech(parA, parB)
{
//statement
}
Function Expression
A Function Expression operates similarly to a Function Declaration or Function Statement, but with one key distinction - it does not require a function name. Instead, function expressions often create anonymous functions. Function expressions can be executed immediately after they are defined, which is known as an immediately-invoked function expression (IIFE).
Syntax:
var tech = function(parA, parB)
{
//statement
}
In a function expression, you assign the function to a variable (in this case, tech) using the assignment operator (=). The function itself is defined using the function keyword, followed by the parameter list and the function body enclosed in curly braces.
Both function declarations and function expressions allow you to define reusable blocks of code, but the distinction lies in their syntax and usage. Function declarations are hoisted to the top of their scope, meaning they can be called before they are defined. Function expressions, on the other hand, are not hoisted and must be defined before they are called.
Difference: Function Declaration vs Function Expression in JavaScript
FUNCTION DECLARATION | FUNCTION EXPRESSION |
A function declaration must have a function name. | A function Expression is similar to a function declaration without the function name. |
Function declaration does not require a variable assignment. | Function expressions can be stored in a variable assignment. |
These are executed before any other code. | Function expressions load and execute only when the program interpreter reaches the line of code. |
The function in function declaration can be accessed before and after the function definition. | The function in function declaration can be accessed only after the function definition. |
Function declarations are hoisted | Function expressions are not hoisted |
How to use Function Declaration and Function Expression
Difference 1: Function name
The first difference between Function Declaration and Function Expression lies in the presence of a function name. Function Declaration requires a name, while Function Expression allows for anonymous functions.
Function declaration:
function doStuff() {};
Function expression:
const doStuff = function() {}
We often see anonymous functions used with ES6 syntax like so:
const doStuff = () => {}
In Function Declaration, the function name is explicitly provided, followed by the function body enclosed in curly braces.
On the other hand, Function Expression assigns the function to a variable using the const keyword (or let/var), making the function anonymous. The function body is defined in the same way as in Function Declaration.
Difference 2: Hoisting
Hoisting is another important aspect to consider. Function Declarations are hoisted to the top of their scope, meaning they can be called before they are defined in the code. Function Expression, however, is not hoisted, and thus, it must be defined before it is called.
Function declarations are hoisted but function expressions are not. It’s easy to understand with an example:
doStuff();
function doStuff() {
// function body
};
The above does not throw an error, but this would:
doStuff(); // Error: doStuff is not a function
const doStuff = () => {
// function body
};
Difference 3: Function Expressions
Function expressions are often preferred when you want to avoid polluting the global scope. By keeping the function anonymous, it can be used and forgotten immediately after invocation.
Immediately Invoked Function Expressions (IIFE) take advantage of function expressions to create a function that is invoked immediately after its creation. Example of an IIFE:
(function() {
// function body
})();
// or using arrow function syntax:
(() => {
// function body
})();
IIFEs are useful in scenarios where you want to encapsulate code and prevent it from affecting the global scope.
Difference 4: Callbacks
Callbacks in JavaScript often involve passing a function as an argument to another function. Function expressions are commonly used in callbacks to limit the scope of the function.
Example using Function Expression as a Callback:
function mapAction(item)
{
// do something with the item
}
array.map(mapAction)
In the above example, the mapAction function is only accessible within the scope where it is defined, providing better encapsulation and reducing potential naming conflicts.
The issue arises when a callback function like mapAction is declared as a Function Declaration. In such cases, the function becomes available globally and can be accessed throughout the entire application, even if it is only needed within a specific scope.
To address this problem, you can use a Function Expression instead, which limits the visibility of the callback function to the scope where it is defined. By using an anonymous function or assigning the function expression to a variable, you can ensure that the callback function is only accessible within the desired context.
Example using an anonymous function as a callback:
array.map(item => {
// do stuff to an item
});
In this example, the callback function is defined inline using an arrow function (ES6 syntax). Since the function is anonymous, it is not accessible outside of the scope where it is used, thus preventing unnecessary exposure to the entire application.
Alternatively, you can define the callback function as a named function expression and pass it as an argument to the map function:
const mapAction = function(item) {
// do stuff to an item
};
array.map(mapAction);
In this case, the mapAction function is declared as a Function Expression and assigned to the mapAction variable. It can then be passed as a callback to the map function. The advantage here is that mapAction will only be available to the code below its initialization, preventing unintended access from other parts of the application.
By using Function Expressions for callbacks, you can effectively limit the visibility and scope of the functions, improving encapsulation and reducing the risk of naming conflicts or unintended use throughout your application.
Summary
Overall, the choice between Function Declaration and Function Expression depends on your specific use case and the scope in which the function is needed. Function Declarations are useful when you want the function to be available throughout the code, while Function Expressions, including anonymous functions and IIFEs, are handy for limiting scope and encapsulating functionality.
Comments