When developing web applications, managing time zones becomes an essential aspect to consider. In most cases, we rely on the server time as the reference for date and time tracking, which is relatively straightforward. However, there are scenarios where we need to track the date and time from the end user's perspective. This requires capturing the time directly from the user's browser using JavaScript. Additionally, considering that users can be located in various time zones, handling time zones correctly can be a challenging task.
This article aims to explore the concepts related to time zones and provide effective methods for handling them using JavaScript. We will delve into the intricacies of time zone management and discuss techniques to ensure accurate time representation for users across different geographical locations. By understanding the complexities of time zones and leveraging JavaScript's capabilities, you can build robust applications that accurately handle and display time information.
Join us on this journey as we uncover the world of time zones and discover how JavaScript can help us tackle its complexities effectively.
How to handle Time Zones in JavaScript
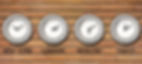
Before we delve into the details, let's familiarize ourselves with some important terminology related to time zones.
1. GMT - Universal Reference:
The world is divided into different time zones, each with its own offset. This offset represents the number of minutes added or subtracted from the reference time to obtain the local time in each time zone.
The reference time used globally is known as Greenwich Mean Time (GMT). It is based on the line of longitude passing through the Greenwich district of London's Old Royal Observatory, extending from the North Pole to the South Pole. If a location is east of the Greenwich Meridian, its local time is typically ahead of GMT. Conversely, locations west of the Greenwich Meridian have local times that are behind GMT.
2. Offset - Difference from the Reference:
The offset of a time zone refers to the number of hours or minutes by which it is ahead of or behind GMT. This offset can vary throughout the year due to factors such as Daylight Saving Time. Countries may also modify their time zone offsets or daylight-saving schedules through legislation. For instance, when Indiana switched from Central to Eastern Time, the time zone's offset changed accordingly.
Countries often name their time zones after themselves. For example, Korea Standard Time (KST) represents the time zone used in Korea, and it has a specific offset value of UTC+09:00.
3. UTC — A better alternative to GMT
Many people mistakenly consider GMT and Coordinated Universal Time (UTC) to be the same and use the terms interchangeably. However, they are not identical.
UTC was established in 1972 to address the issue of Earth's rotational slowing. It is based on International Atomic Time and utilizes the caesium atomic frequency to set the time standard. UTC is considered a more precise and reliable time standard compared to GMT. Although there are subtle differences between GMT and UTC, they are hardly noticeable in everyday development.
var d = new Date();
var n = d.getTimezoneOffset(); // Returns UTC Offset in Minutes
To retrieve the UTC offset in minutes for the current date and time using JavaScript, you can use the getTimezoneOffset() method on a Date object. This method returns the time zone offset relative to UTC.
Using Date Time Processing with JavaScript in Practice
When working with web applications, it is often necessary to handle time zones, especially when dealing with end user interactions. JavaScript provides built-in features and libraries to process date and time, allowing us to effectively manage time zone-related tasks. In this article, we will explore the practical aspects of using date and time processing in JavaScript.
Date Time Operations — Using Pure JavaScript
JavaScript's Date object comes with various methods for performing date and time calculations. It internally manages time data using absolute numbers, such as Unix time, and provides constructors and methods like parse(), getHour(), and setHour(). However, it's important to note that the Date object is influenced by the client's local time zone, which is determined by the operating system running the browser. When generating a Date object from user input, it represents the client's local time zone. Although the Date object internally keeps track of time in UTC, it usually accepts and outputs data in the local time of the computer it's running on. When working with different time zones, JavaScript has limited native methods available.
ISOString - One operation that the Date object can perform with non-local time zones is parsing a text containing any time zone's numeric UTC offset. The output Date object does not retain the original local time and offset.
var d = new Date();
d.toISOString() //=> "2021-09-06T13:52:23.770Z"
d.valueOf() //=> 1630936367439 (this is what is actually stored in the object)
Date Time Operations — Using Third-Party Libraries
To overcome the limitations of native JavaScript methods and handle time zones more effectively, there are several third-party libraries available. These libraries offer a wide range of date formatting and manipulation functions. They can be divided into two types based on whether they support the ECMAScript Internationalization API or not.
Intl-based Libraries: These libraries utilize the Intl API for their data and are recommended for new development. Examples include Luxon (successor to Moment.js) and date-fns-tz (an extension for date-fns).
Non-Intl Libraries: These libraries package their time zone data and are also frequently updated. Examples include js-joda/timezone (extension for js-joda), date-fns-timezone (extension for older versions of date-fns), BigEasy/TimeZone, and tz.js. It is important to avoid using discontinued libraries such as MomentJS, WallTime-js, and TimeZoneJS for new projects.
Date-fns
Among these libraries, Date-fns is a popular choice. It is a small library with a simple API that offers a wide range of functions for date manipulation. With nearly 140 functions, Date-fns is often referred to as "Lodash for dates." It has advantages such as immutability, native date support, modularity, speed, comprehensive documentation, internationalization (I18n) support, and compatibility with TypeScript and Flow.
To use Date-fns, you can install the library via npm using the command npm install date-fns. Date-fns is compatible with both CommonJS and ES modules. Instead of importing the entire API, you can selectively import the specific functions you need. For example, to display the current date, you can import the format function and use it as follows:
//Import the function initially
const {format} = require('date-fns');
//today's date
const today =format(new Date(),'dd.MM.yyyy');
console.log(today);
In this example, we import the format function from the date-fns library and use it to format the current date in the desired format.
Working with Time Zones
When working with time zones in JavaScript, the Date-fns library provides support for handling UTC or ISO date strings and displaying them in the local time of your users. However, managing multiple time zones can be challenging. Let's explore how we can tackle these situations using Date-fns with an example.
Before proceeding, make sure to install the date-fns-tz library using the command npm install date-fns-tz.
1. Zoned Time to UTC:
To convert zoned time to UTC, you can use the zonedTimeToUtc function provided by Date-fns. This function takes a zoned time and converts it to the corresponding UTC time. Here's an example:
const { format, utcToZonedTime } = require("date-fns-tz");
const today = new Date();
const timeZone = 'Europe/Paris';
const timeInParis = utcToZonedTime(today, timeZone);
console.log(`Default time zone: ${format(today, 'yyyy-MM-dd HH:mm:ss')} Time in Paris: ${format(timeInParis, 'yyyy-MM-dd HH:mm:ss')}`);
You need to make sure that you have the 'date-fnz-tz' package installed.
The output will be like this:
Default time zone: 2021-09-10 12:34:56 Time in Paris: 2021-09-10 18:34:56
2. UTC to Zoned Time:
Conversely, if you have UTC time and need to convert it to a specific time zone, you can use the utcToZonedTime function. This function takes a UTC time and converts it to the corresponding time in the specified time zone. Here's an example:
const { format, zonedTimeToUtc } = require("date-fns-tz");
const today = newDate();
const timeZone = 'Europe/Paris';
const timeInParis = zonedTimeToUtc(today, timeZone);
console.log(`Default time zone: ${format(today, 'yyyy-MM-dd HH:mm:ss')} Time in Paris: ${format(timeInParis, 'yyyy-MM-dd HH:mm:ss')}`);
Conclusion
There are several libraries available for working with time zones in JavaScript. These libraries typically implement the standard IANA time zone database and provide functions to handle time zone-related operations. While they can't change the behavior of the native Date object, they offer convenient methods for working with different time zones.
Modern libraries, such as those using the Intl API's time zone data, are recommended for new projects as they are more likely to be future-proof. Older libraries may have additional overhead, especially in web browsers, due to the potentially large size of the time zone database.