Throttling and Debouncing in JavaScript
- The Tech Platform
- Jul 29, 2021
- 2 min read
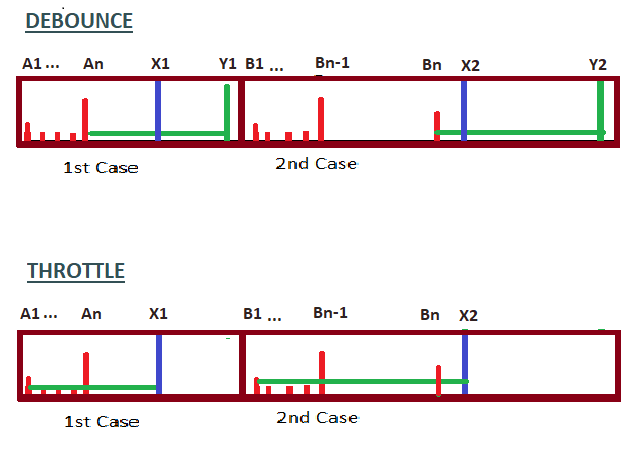
Throttling
Throttling is also called throttle function which is used in websites. Throttling is used to call a function. Then the number of times a button is clicked the function will be called the same number of times.
To throttle a function means to ensure that the function is called at most once in a specified time period (for instance, once every 10 seconds). This means throttling will prevent a function from running if it has run “recently”. Throttling also ensures a function is run regularly at a fixed rate.
Other use cases :
Throttling a button click so we can’t spam click
Throttling an API call
Throttling a mousemove/touchmove event handler.
Advantages:
It prevent frequent calling of the function.
It makes the website faster and controls the rate at which a particular function is called.
Example:
Without Throttling Function:
In this code suppose if the button is clicked 500 times then the function will be clicked 500 times this is controlled by a throttling function.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport"
content="width=device-width,
initial-scale=1.0" />
<title>
JavaScript | Without Throttling
</title>
</head>
<body>
<button id="nothrottle">Click Me</button>
<script>
// Selected button with th egiven id
const btn = document.querySelector("#nothrottle");
// Add event listener to the button
// to listen the click event
btn.addEventListener("click", () => {
console.log("button is clicked");
});
</script>
</body>
</html>
With Throttling Function:
In this code if a user continues to click the button every click is executed after 1500ms except the first one which is executed as soon as the user clicks on the button.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<title>
JavaScript | With Throttling
</title>
</head>
<body>
<button id="throttle">Click Me</button>
<script>
const btn=document.querySelector("#throttle");
// Throttling Function
const throttleFunction=(func, delay)=>{
// Previously called time of the function
let prev = 0;
return (...args) => {
// Current called time of the function
let now = new Date().getTime();
// Logging the difference between previously
// called and current called timings
console.log(now-prev, delay);
// If difference is greater than delay call
// the function again.
if(now - prev> delay){
prev = now;
// "..." is the spread operator here
// returning the function with the
// array of arguments
return func(...args);
}
}
}
btn.addEventListener("click", throttleFunction(()=>{
console.log("button is clicked")
}, 1500));
</script>
</body>
</html>
Debouncing
Debouncing is a programming practice used to ensure that time-consuming tasks do not fire so often, that it stalls the performance of the web page. The debounced function will ignore all calls to it until the calls have stopped for a specified time period. Only then will it call the original function.
Debouncing forces a function to wait a certain amount of time before running again. In other words, it limits the rate at which a function gets invoked.
Other use cases:
Debouncing a resize event handler.
Debouncing a save function in an autosave feature.
Don’t do anything while the user drags and drops.
Don’t make any Axios requests until the user stops typing.
Example:
const debounce = function(func,delay){
let timer;
return function()
{
//anonymous function
const context = this;
const args = arguments;
clearTimeout(timer);
timer = setTimeout(()=>
{
func.apply(context,args)
},
delay);
}
}
The Tech Platform
Comments