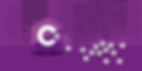
In ASP.NET Core, the IDisposable interface provides a mechanism for releasing unmanaged resources used by a class. It allows developers to clean up any unmanaged resources that an object has acquired during its lifetime. This article will provide a detailed explanation of how to use IDisposable in ASP.NET Core.
Understanding IDisposable The IDisposable interface is part of the System namespace in .NET. It contains only one method, Dispose(), which is called to release unmanaged resources. Classes that implement IDisposable should call the Dispose() method when they are no longer needed to release any resources they hold.
Implementing IDisposable In ASP.NET Core, classes that use unmanaged resources should implement the IDisposable interface. To implement IDisposable, you need to follow these steps:
Step 1: Declare the IDisposable interface in the class definition.
public class MyService : IDisposable
{
// Class definition goes here
}
Step 2: Implement the Dispose() method in the class definition.
public void Dispose()
{
// Release any unmanaged resources here
}
Step 3: Use the using statement to create an instance of the class.
using (var myService = new MyService())
{
// Code that uses myService goes here
}
The using statement automatically calls the Dispose() method when the block of code is finished executing, ensuring that any unmanaged resources are properly cleaned up.
Using IDisposable in Dependency Injection In ASP.NET Core, the dependency injection (DI) system provides a way to manage the lifetime of objects that implement IDisposable. When a service is registered with the DI container, it can be configured to dispose of the object automatically when it is no longer needed. To use IDisposable in DI, you need to follow these steps:
Step 1: Register the service with the DI container.
services.AddScoped<IMyService, MyService>();
Step 2: Implement the IDisposable interface in the service class.
public class MyService : IMyService, IDisposable
{
// Class definition goes herepublic void Dispose()
{
// Release any unmanaged resources here
}
}
Step 3: Use the Dispose() method to release any unmanaged resources.
The service is now registered with the DI container and will be automatically disposed of when it is no longer needed.
Best Practices for Using IDisposable
When using IDisposable in ASP.NET Core, it's important to follow these best practices:
Only implement IDisposable when your class uses unmanaged resources. Implementing IDisposable when it's not necessary can result in unnecessary code and performance issues.
Always call the Dispose() method when you're finished using an object that implements IDisposable. This ensures that any unmanaged resources are properly cleaned up.
Use the using statement to create instances of classes that implement IDisposable. This ensures that the Dispose() method is called when the block of code is finished executing.
When using IDisposable in DI, make sure that your service class is registered with the DI container and that it is configured to dispose of the object when it is no longer needed.
Benefits of using IDisposable
1. Proper resource management: IDisposable helps in the proper management of resources used by an application. When an object implements IDisposable, it can release unmanaged resources such as file handles, database connections, and network sockets. This ensures that the resources are freed up when they are no longer needed, preventing resource leaks and improving application performance.
2. Cleaner code: Implementing IDisposable makes your code cleaner and more maintainable. By implementing the IDisposable interface, you can encapsulate the cleanup logic for your objects in a single place. This makes your code easier to read, understand, and modify, and reduces the risk of introducing bugs.
3. Improved performance: Properly disposing of unmanaged resources can help improve the performance of your application. When an object is no longer needed, its resources are released immediately, freeing up system resources that can be used by other parts of the application.
4. Reduced memory usage: Implementing IDisposable can help reduce memory usage in your application. When you dispose of an object, any unmanaged resources it was using are released immediately, and the object can be garbage collected more quickly. This helps reduce memory usage and can improve the overall performance of your application.
Conclusion
The IDisposable interface provides a mechanism for releasing unmanaged resources used by a class. In ASP.NET Core, IDisposable can be used to manage the lifetime of objects that use unmanaged resources. By following best practices and implementing IDisposable only when necessary, you can ensure that your ASP.NET Core application is efficient and performs well.