Enum as Required Field in ASP.NET Core WebAPI
- The Tech Platform
- Oct 22, 2021
- 3 min read
Updated: Mar 18, 2023
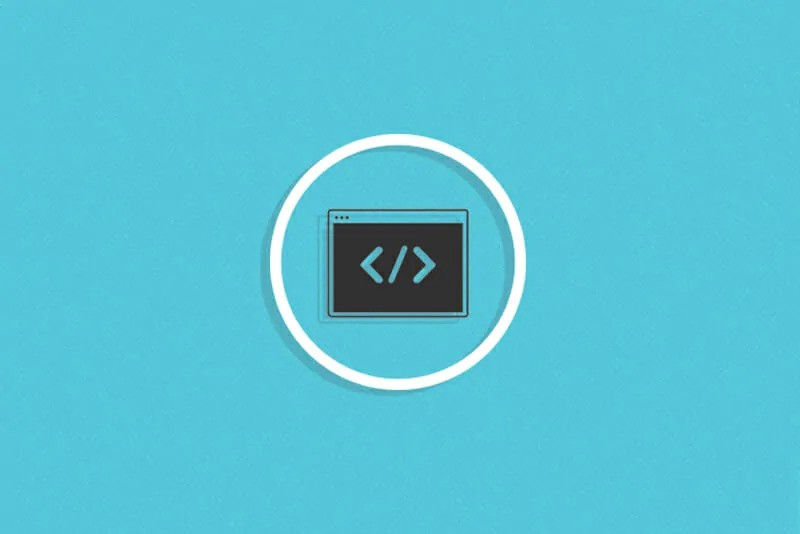
Enums in C# are a set of named constants that represent a finite list of values. They are used to define a set of related named constants, which can be used in place of primitive data types. In ASP.NET Core WebAPI, enums can be used as properties in models to represent a fixed set of values for a specific field.
Using [Required] element
To make an enum property a required field in ASP.NET Core WebAPI, you can use the [Required] attribute from the System.ComponentModel.DataAnnotations namespace.
Here's an example of how to use the [Required] attribute on an enum property in a model:
using System.ComponentModel.DataAnnotations;
public enum Gender
{
Male,
Female,
Other
}
public class Person
{
public int Id { get; set; }
[Required]
public string Name { get; set; }
[Required]
public Gender Gender { get; set; }
}
In this example, we have an enum called Gender, which represents the gender of a person. We also have a Person class with two properties: Name, which is a required string property, and Gender, which is a required Gender property.
By applying the [Required] attribute on the Gender property, we ensure that the client cannot submit an empty or null value for the Gender property when making requests to the WebAPI.
When a request is made with an empty or null value for the Gender property, the WebAPI returns a 400 Bad Request response with a message indicating that the Gender field is required.
{
"errors":
{
"Gender": [
"The Gender field is required."
]
},
"title": "One or more validation errors occurred.",
"status": 400,
"traceId": "..."
}
Note that in order for the [Required] attribute to work on an enum property, the enum must have a defined value for each of its members. In other words, you cannot have an enum member without a defined value or a duplicate value.
When you use enums as required fields in ASP.NET Core WebAPI models, it allows you to ensure that only valid values are accepted by your API, reducing the possibility of errors caused by incorrect or missing data.
Using [JSON Property]
In addition to the [Required] attribute, you can also use the [JsonProperty] attribute from the Newtonsoft.Json namespace to make an enum property a required field in ASP.NET Core WebAPI.
To make an enum property required using the [JsonProperty] attribute, you need to set the Required property of the attribute to Required.Always.
Here's an example of how to use the [JsonProperty] attribute with Required.Always on an enum property in a model:
using Newtonsoft.Json;
using System.Runtime.Serialization;
public enum Gender
{
Male,
Female,
Other
}
public class Person
{
public int Id { get; set; }
[JsonProperty(Required = Required.Always)]
public string Name { get; set; }
[JsonProperty(Required = Required.Always)]
public Gender Gender { get; set; }
}
In this example, we have an enum called Gender, which represents the gender of a person. We also have a Person class with two properties: Name, which is a required string property, and Gender, which is a required Gender property.
By applying the [JsonProperty] attribute with Required.Always on the Gender property, we ensure that the client cannot submit an empty or null value for the Gender property when making requests to the WebAPI.
When a request is made with an empty or null value for the Gender property, the WebAPI returns a 400 Bad Request response with a message indicating that the Gender field is required.
{
"errors":
{
"Gender": [
"The Gender field is required."
]
},
"title": "One or more validation errors occurred.",
"status": 400,
"traceId": "..."
}
Note that when you use the [JsonProperty] attribute with Required.Always on an enum property, you need to include the Newtonsoft.Json package in your project, as this attribute is not available in the built-in System.Text.Json library.
Also, make sure to add the [DataContract] attribute to your model class and the [DataMember] attribute to each of your properties if you're using DataContractJsonSerializer for serialization, as this attribute tells the serializer which properties to include in the serialized JSON object.
Comments