How to throw Exception in Java
- The Tech Platform
- Jun 23, 2023
- 5 min read
Exception handling is an essential aspect of Java programming that allows you to handle and manage unexpected or exceptional situations gracefully. One of the fundamental concepts in exception handling is throwing exceptions. In Java, you can deliberately throw an exception to indicate that an exceptional condition has occurred during the execution of your program. By throwing an exception, you can interrupt the normal flow of execution and transfer control to an appropriate exception-handling mechanism.
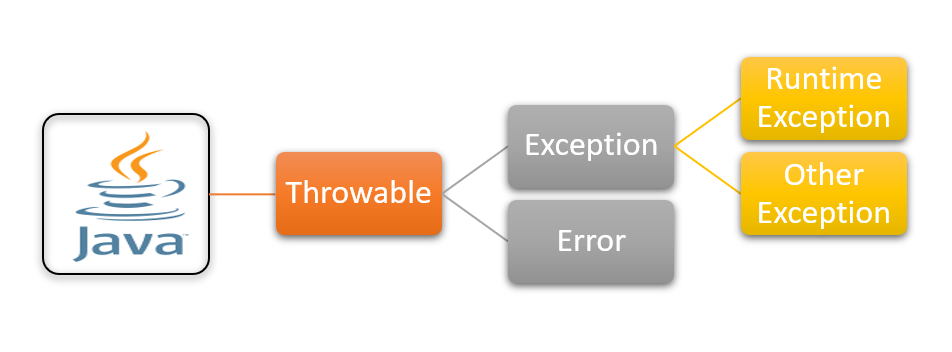
In this article, we will explore how to throw exceptions in Java. We will delve into the syntax and usage of throwing exceptions, understand when and why you should throw exceptions, and examine how exceptions can be caught and handled.
Table of content:
What is an Exception in Java?
Syntax
Types of Exception in Java
Exception handling Techniques
Conclusion
What is an Exception in Java?
In Java, an exception is an event or condition that disrupts the normal flow of program execution and indicates the occurrence of an error or exceptional situation. It is represented by an object that contains information about the exception type and additional details about the error.
The primary purpose of throwing exceptions in Java is to handle and communicate exceptional conditions or errors that may occur during the execution of a program. By throwing an exception, developers can indicate that something unexpected or erroneous has happened and provide a mechanism for dealing with such situations.
Here are the key purposes of throwing exceptions:
Error Signaling: Exceptions serve as a means to signal errors or exceptional conditions in the code. When an exceptional situation arises, such as invalid input, file not found, or division by zero, throwing an exception allows the program to transfer control to an appropriate exception handler.
Error Propagation: Exceptions can be propagated up the call stack, allowing higher-level code or the caller of a method to handle the exception. This enables a clear separation of error-handling logic from the regular flow of code execution, promoting modular and maintainable code.
Error Handling: Throwing exceptions allows developers to write specific error-handling code to gracefully handle exceptional situations. Exception handlers can catch and handle specific types of exceptions, perform necessary recovery actions, provide meaningful error messages, or take appropriate corrective measures.
Program Robustness: By throwing exceptions, developers can anticipate and handle exceptional cases, making their programs more robust. This helps prevent unexpected crashes, provides informative error messages to users, and allows for graceful recovery or termination of the program when necessary.
Debugging and Troubleshooting: Exceptions aid in debugging and troubleshooting by providing a stack trace that shows the sequence of method calls leading to the exception. This information helps identify the source of the error and assists developers in pinpointing and resolving issues.
The syntax for Throwing Exceptions
Here's the syntax for throwing an exception:
throw new ExceptionClass("Exception message");
throw: The throw keyword is used to explicitly throw an exception.
new ExceptionClass: It creates a new instance of the exception class that represents the specific type of exception being thrown. You can use any built-in exception class or create your own custom exception class.
"Exception message": This is an optional message that provides additional information about the exception. It helps in identifying the cause or nature of the exception.
Code Example:
public class CustomExceptionExample {
public static void main(String[] args) {
try {
int result = divide(10, 0);
System.out.println("Result: " + result);
} catch (ArithmeticException ex) {
System.out.println("Error: " + ex.getMessage());
}
}
public static int divide(int dividend, int divisor) {
if (divisor == 0) {
throw new ArithmeticException("Cannot divide by zero");
}
return dividend / divisor;
}
}
In the above example, the divide method throws an ArithmeticException if the divisor is zero. The exception is thrown using throw new ArithmeticException("Cannot divide by zero"). The message "Cannot divide by zero" provides information about the cause of the exception.
When the divide method is called with a divisor of 0, the exception is thrown and caught in the catch block, where the error message is displayed. Without catching the exception, the program would terminate abruptly and display an error stack trace.
By throwing exceptions, you can indicate exceptional conditions or errors in your code and provide a mechanism to handle and communicate those errors effectively. It helps in separating error-handling logic from regular program flow and promotes robust and reliable code execution.
Types of Exception in Java
Exceptions are categorized into two types:
Checked exceptions
Unchecked exceptions.
Here are examples of each:
1. Checked Exceptions:
Checked exceptions are the exceptions that must be either caught or declared in the method signature using the throws keyword. These exceptions typically represent recoverable conditions or expected error scenarios.
Types of Checked Exceptions:
ClassNotFoundException
InterruptedException
IOException
InstantiationException
SQLException
FileNotFoundException
Example: FileNotFoundException
import java.io.File;
import java.io.FileNotFoundException;
import java.util.Scanner;
public class FileProcessor {
public void processFile(String filePath) throws FileNotFoundException {
File file = new File(filePath);
Scanner scanner = new Scanner(file);
// Perform operations on the file// ...
scanner.close();
}
}
In the above example, the FileNotFoundException is a checked exception that may occur if the specified file is not found. The processFile method declares that it can throw this exception, indicating to the caller that they need to handle it or propagate it further.
2. Unchecked Exceptions (Runtime Exception):
Unchecked exceptions, also known as runtime exceptions, do not require explicit handling or declaration. They occur at runtime and represent unexpected conditions or programming errors that are typically not recoverable.
Types of Unchecked Exceptions:
ArithmeticException
ClassCastException
NullPointerException
ArrayIndexOutOfBoundsException
ArrayStoreException
IllegalThreadStateException
Example: ArithmeticException
public class Calculator {
public int divide(int dividend, int divisor) {
if (divisor == 0) {
throw new ArithmeticException("Cannot divide by zero");
}
return dividend / divisor;
}
}
In the above example, the ArithmeticException is an unchecked exception that occurs if the divisor is zero. Instead of declaring it in the method signature or using a try-catch block, we directly throw the exception using the throw keyword.
The unchecked exceptions can be caught and handled if desired, but it's not mandatory. They are often used to indicate programming errors that should be fixed during development.
Checked vs Unchecked Exception
Both checked and unchecked exceptions are subclasses of Throwable, and they both inherit from the Exception class. The distinction between them lies in how they are handled and enforced by the Java compiler.
Here we have the difference:
Factors | Checked Exceptions | Unchecked Exception |
---|---|---|
Handling | Must be handled explicitly using try-catch blocks or declared in the method signature using the throws keyword. | Not required to be handled explicitly. Can be handled optionally. |
Compile-time checking | Checked at compile-time, and the compiler enforces handling or declaration. | Not checked at compile-time. Compilation is not affected. |
Inheritance | Generally, subclasses of Exception (except for RuntimeException and its subclasses). | Subclasses of RuntimeException and its subclasses. |
Recoverability | Typically represents recoverable conditions or expected error scenarios. | Typically represents programming errors or unexpected conditions that are not easily recoverable. |
Examples | IOException, SQLException, ClassNotFoundException | NullPointerException, ArrayIndexOutOfBoundsException, ArithmeticException |
Exception Handling Techniques
In Java, exception handling allows you to gracefully handle and recover from unexpected or exceptional situations that can occur during the execution of your program. Here are some common exception handling techniques in Java along with code examples:
1. Try-Catch:
The try-catch block is used to catch and handle exceptions. Code that may potentially throw an exception is placed within the try block, and the corresponding exception handling logic is placed within the catch block.
Example:
try {
// Code that may throw an exception
int result = divide(10, 0);
System.out.println("Result: " + result);
} catch (ArithmeticException ex) {
// Exception handling logic
System.out.println("Error: " + ex.getMessage());
}
public static int divide(int dividend, int divisor)
{
if (divisor == 0) {
throw new ArithmeticException("Cannot divide by zero");
}
return dividend / divisor;
}
2. Multiple Catch Blocks:
You can use multiple catch blocks to handle different types of exceptions separately. This allows you to provide specific handling logic for each type of exception.
Example:
try {
// Code that may throw an exception
FileReader fileReader = new FileReader("file.txt");
BufferedReader bufferedReader = new BufferedReader(fileReader);
String line = bufferedReader.readLine();
System.out.println("First line: " + line);
bufferedReader.close();
} catch (FileNotFoundException ex) {
System.out.println("File not found: " + ex.getMessage());
} catch (IOException ex) {
System.out.println("Error reading file: " + ex.getMessage());
}
3. Finally Block:
The finally block is used to specify code that should be executed regardless of whether an exception occurs or not. It is typically used to release resources or perform cleanup operations.
Example:
BufferedReader bufferedReader = null;
try {
// Code that may throw an exception
FileReader fileReader = new FileReader("file.txt");
bufferedReader = new BufferedReader(fileReader);
String line = bufferedReader.readLine();
System.out.println("First line: " + line);
} catch (IOException ex) {
System.out.println("Error reading file: " + ex.getMessage());
} finally {
// Cleanup code
try {
if (bufferedReader != null) {
bufferedReader.close();
}
} catch (IOException ex) {
System.out.println("Error closing file: " + ex.getMessage());
}
}
These exception handling techniques allow you to control the flow of your program and handle exceptional situations effectively. By catching and handling exceptions, you can provide meaningful error messages, take appropriate actions, and ensure the smooth execution of your Java programs.
so cool!
https://depositphotos.com/vector/vector-2021-03-02-sitemap-4705.xml
https://depositphotos.com/vector/vector-2021-03-02-sitemap-4706.xml
https://depositphotos.com/vector/vector-2021-03-04-sitemap-4707.xml
https://depositphotos.com/vector/vector-2021-03-04-sitemap-4708.xml
https://depositphotos.com/vector/vector-2019-12-05-sitemap-4709.xml
https://depositphotos.com/vector/vector-2021-03-05-sitemap-4710.xml
https://depositphotos.com/vector/vector-2021-02-23-sitemap-4711.xml
https://depositphotos.com/vector/vector-2021-03-04-sitemap-4712.xml
https://depositphotos.com/vector/vector-2021-03-07-sitemap-4713.xml
https://depositphotos.com/vector/vector-2021-02-26-sitemap-4714.xml
https://depositphotos.com/vector/vector-2021-03-07-sitemap-4715.xml
https://depositphotos.com/vector/vector-2021-02-16-sitemap-4716.xml
https://depositphotos.com/vector/vector-2021-02-19-sitemap-4717.xml
https://depositphotos.com/vector/vector-2021-03-08-sitemap-4718.xml
https://depositphotos.com/vector/vector-2021-03-10-sitemap-4719.xml
https://depositphotos.com/vector/vector-2021-03-10-sitemap-4720.xml
https://depositphotos.com/vector/vector-2021-03-10-sitemap-4721.xml
https://depositphotos.com/vector/vector-2021-03-03-sitemap-4722.xml
https://depositphotos.com/vector/vector-2021-03-10-sitemap-4723.xml
https://depositphotos.com/vector/vector-2021-03-12-sitemap-4724.xml