Mastering Java Switch Statements
- The Tech Platform
- Oct 3, 2023
- 8 min read
In the vast world of programming, decision-making is a fundamental concept that forms the backbone of almost every software application. The ability to make choices, direct program flow, and execute specific code based on conditions is what empowers developers to create dynamic and responsive applications. One indispensable tool in a programmer's arsenal for effective decision-making is the Java Switch statement.
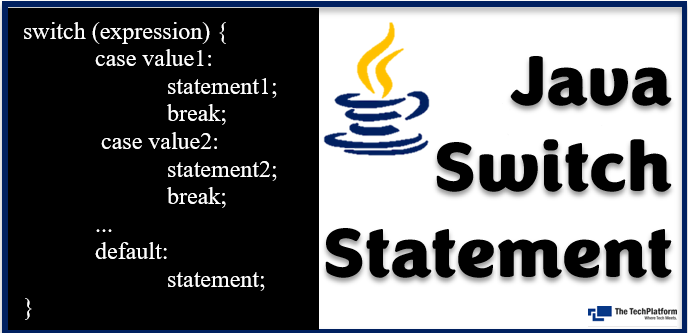
In this comprehensive guide, we'll explore Java Switch statements, exploring their syntax, common scenarios where they excel, and when to choose them over the traditional if-else statements.
Table of Contents:
Syntax and structure of Java Switch Statement
How does it compare to if-else statements for multiple conditional checks
Advantages of Java Switch Statement
Disadvantages of Java Switch Statement
Menu System
Data Validation
State Machines
Int
Char
String
Enum
Fall-through behavior
Introduction to Java Switch Statement
A Java switch statement is a control flow statement that allows you to execute one statement from multiple conditions. It is similar to an if-else-if ladder statement, but it is more efficient and concise for multiple conditional checks.
Syntax and structure of Java Switch statement
The general syntax of a Java switch statement is as follows:
switch (expression) {
case value1:
statement1;
break;
case value2:
statement2;
break;
...
default:
statement;
}
The expression can be a byte, short, char, int, long, enum, or String. The value1, value2, ... are the values to be compared against the expression. If the expression matches any of the values, then the corresponding statement1, statement2, ... is executed. If the expression does not match any of the values, then the default statement is executed.
How does it compare to if-else statements for multiple conditional checks
The Java switch statement is more concise and efficient than the if-else-if ladder statement for multiple conditional checks because it only needs to evaluate the expression once and then compare it to the case values. The if-else-if ladder statement, on the other hand, needs to evaluate the expression multiple times, once for each if-else statement.
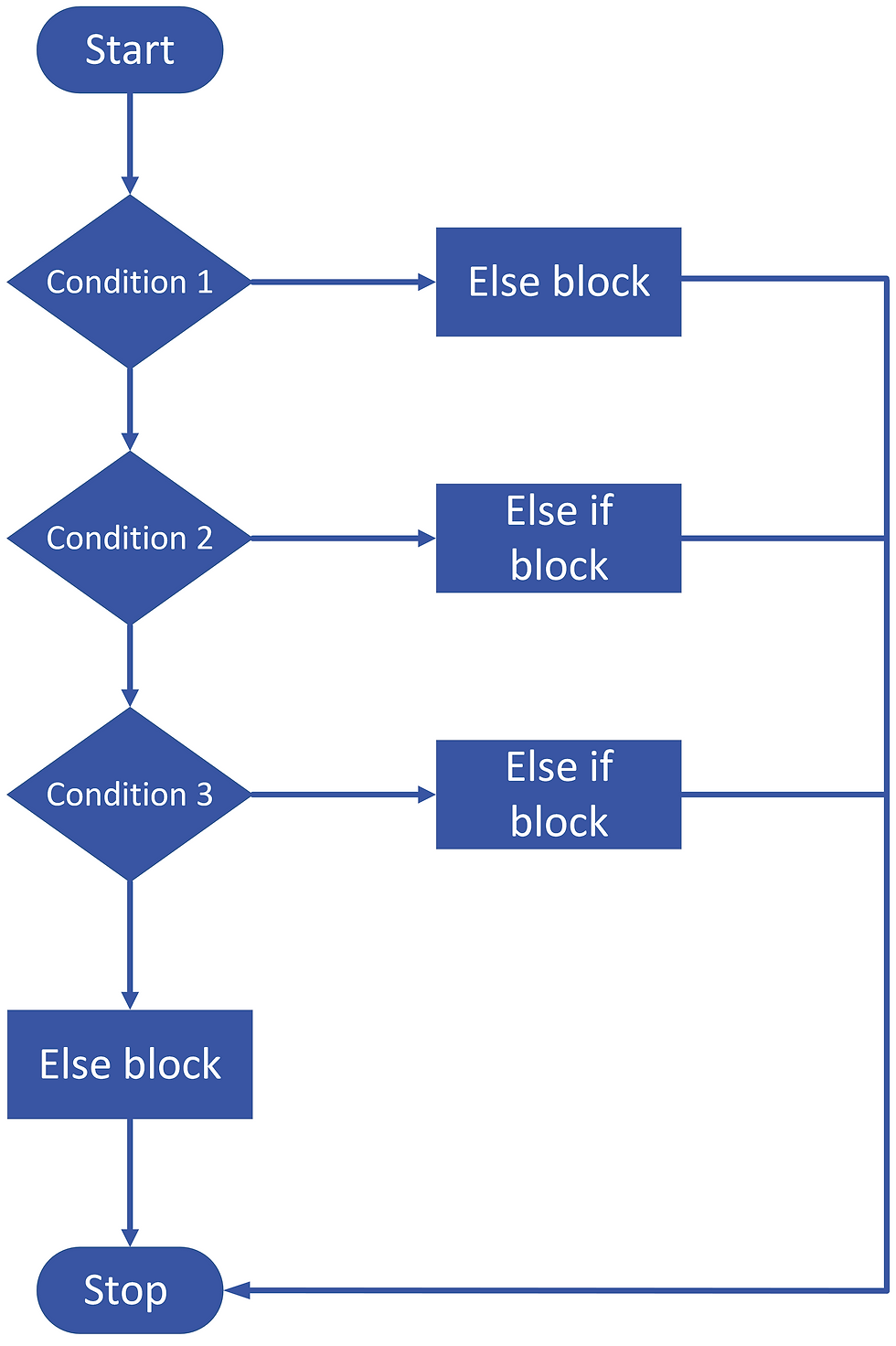
Here is an example of an if-else-if ladder statement for multiple conditional checks:
int day = 1;
if (day == 1) {
System.out.println("Monday");
} else if (day == 2) {
System.out.println("Tuesday");
} else if (day == 3) {
System.out.println("Wednesday");
} else if (day == 4) {
System.out.println("Thursday");
} else if (day == 5) {
System.out.println("Friday");
} else if (day == 6) {
System.out.println("Saturday");
} else if (day == 7) {
System.out.println("Sunday");
} else {
System.out.println("Invalid day");
}
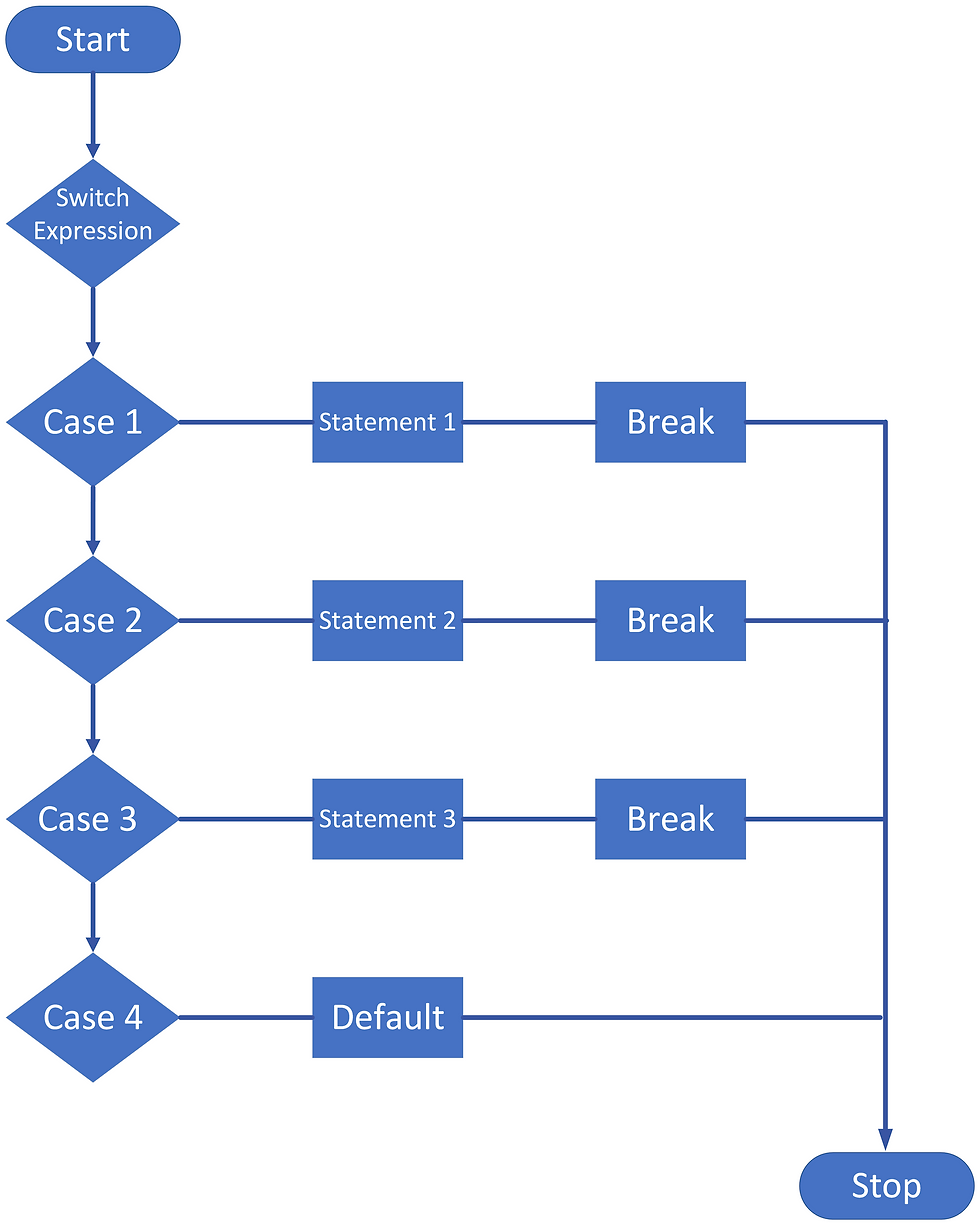
Here is the equivalent Java switch statement for the above code:
int day = 1;
switch (day) {
case 1:
System.out.println("Monday");
break;
case 2:
System.out.println("Tuesday");
break;
case 3:
System.out.println("Wednesday");
break;
case 4:
System.out.println("Thursday");
break;
case 5:
System.out.println("Friday");
break;
case 6:
System.out.println("Saturday");
break;
case 7:
System.out.println("Sunday");
break;
default:
System.out.println("Invalid day");
}
You should use a Java switch statement instead of an if-else-if ladder statement when you have multiple conditional checks to perform, especially if the conditional checks are complex.
However, there are a few cases where you may want to use an if-else-if ladder statement instead of a switch statement:
If the Java switch statement is complex in many cases, it can be difficult to read and maintain.
If the expression is evaluated many times, the switch statement can be inefficient.
If you need to execute different code blocks for different ranges of values, an if-else-if chain may be more suitable.
Advantages of using a Java switch statement:
More concise and efficient than if-else-if ladder statements.
Easier to read and maintain.
Can be used for multiple conditional checks, including string comparisons.
Disadvantages of using a Java switch statement:
Can be difficult to read and maintain complex switch statements in many cases.
Can be inefficient if the expression is evaluated many times.
Common Scenarios where Java Switch Statements are Beneficial
Switch statements are beneficial in common scenarios where multiple conditional checks are required, such as:
Menu systems: Java Switch statements can be used to implement menu systems in a concise and efficient manner. For example, the following switch statement could be used to implement a simple menu with three options:
int choice = 1;
switch (choice) {
case 1:
System.out.println("Option 1 selected");
break;
case 2:
System.out.println("Option 2 selected");
break;
case 3:
System.out.println("Option 3 selected");
break;
default:
`System.out.println("Invalid choice");
}
Data validation: Java Switch statements can be used to validate user input or data from a database. For example, the following switch statement could be used to validate a user's input for a month:
String month = "January";
switch (month) {
case "January":
case "February":
case "March":
case "April":
case "May":
case "June":
case "July":
case "August":
case "September":
case "October":
case "November":
case "December":
System.out.println("Valid month");
break;
default:
System.out.println("Invalid month");
}
State machines: Java Switch statements can be used to implement state machines, which are used to model the behavior of a system in different states. For example, the following switch statement could be used to implement a simple state machine for a traffic light:
enum TrafficLightState {
RED,
YELLOW,
GREEN
}
TrafficLightState state = TrafficLightState.RED;
switch (state) {
case RED:
System.out.println("Traffic light is red");
// Wait for a certain amount of time
state = TrafficLightState.YELLOW;
break;
case YELLOW:
System.out.println("Traffic light is yellow");
// Wait for a certain amount of time
state = TrafficLightState.GREEN;
break;
case GREEN:
System.out.println("Traffic light is green");
// Wait for a certain amount of time
state = TrafficLightState.RED;
break;
}
When to use Java Switch instead of if-else
In general, you should use a Java switch statement instead of an if-else chain when you have multiple conditional checks that need to be performed. Switch statements are more concise, efficient, and easier to read and maintain than if-else chains.
However, there are a few cases where you may want to use an if-else chain instead of a switch statement:
If the Java switch statement is complex in many cases, it can be difficult to read and maintain.
If the expression is evaluated many times, the switch statement can be inefficient.
If you need to execute different code blocks for different ranges of values, an if-else chain may be more suitable.
Overall, Java switch statements are a powerful tool for multiple conditional checks in Java. They are more concise, efficient, and easier to read and maintain than if-else chains. However, it is important to use switch statements wisely, especially for complex cases.
Switch Statement with Data Types
To use the Java switch statement with various data types, you simply need to make sure that the expression and the case values are of the same data type.
Example of using a Java switch statement with an int data type:
int day = 1;
switch (day) {
case 1:
System.out.println("Monday");
break;
case 2:
System.out.println("Tuesday");
break;
case 3:
System.out.println("Wednesday");
break;
// ...
}
Example of using a Java switch statement with a char data type:
char grade = 'A';
switch (grade) {
case 'A':
System.out.println("Excellent");
break;
case 'B':
System.out.println("Good");
break;
case 'C':
System.out.println("Average");
break;
// ...
}
Example of using a Java switch statement with a String data type:
String fruit = "Apple";
switch (fruit) {
case "Apple":
System.out.println("Apple is a good source of fiber");
break;
case "Banana":
System.out.println("Banana is a good source of potassium");
break;
case "Orange":
System.out.println("Orange is a good source of vitamin C");
break;
// ...
}
Example of using a Java switch statement with an enum data type:
enum TrafficLightState {
RED,
YELLOW,
GREEN
}
TrafficLightState state = TrafficLightState.RED;
switch (state) {
case RED:
System.out.println("Traffic light is red");
// Wait for a certain amount of time
state = TrafficLightState.YELLOW;
break;
case YELLOW:
System.out.println("Traffic light is yellow");
// Wait for a certain amount of time
state = TrafficLightState.GREEN;
break;
case GREEN:
System.out.println("Traffic light is green");
// Wait for a certain amount of time
state = TrafficLightState.RED;
break;
}
Fall-through behavior
The fall-through behavior is a feature of the Java switch statement that allows you to execute multiple case statements without having to break after each one. This is done by omitting the break statement after the case statement.
Example of fall-through behavior:
String fruit = "Apple";
switch (fruit) {
case "Apple":
System.out.println("Apple is a good source of fiber");
case "Banana":
System.out.println("Banana is a good source of potassium");
case "Orange":
System.out.println("Orange is a good source of vitamin C");
// ...
}
In the above example, the code will execute the System.out.println statements for all three case statements, even if the fruit variable is equal to "Apple". This is because the break statement is omitted after the first case statement.
When to use fall-through behavior intentionally
There are a few cases where you may want to use fall-through behavior intentionally:
When you want to execute the same code for multiple case values.
When you want to implement a state machine.
When you want to implement a menu system.
However, it is important to use fall-through behavior carefully, as it can make your code difficult to read and maintain.
Enhanced Java Switch
The enhanced switch statement, introduced in Java 12, is a more powerful and expressive version of the traditional switch statement. It offers a number of new features, including:
Case expressions: Case expressions allow you to evaluate expressions and return a value, which can then be used to determine the case to execute.
Yield: The yield keyword allows you to return a value from a switch statement, which can then be used in the surrounding code.
Multiple case labels: You can now specify multiple case labels for a single case statement.
Arrow syntax: You can now use an arrow (->) to separate the case labels from the case body.
Code examples showcasing the enhanced Switch in action:
Example of a case expression:
int dayOfWeek = 3;
String day = switch (dayOfWeek) {
case 1 -> "Monday";
case 2 -> "Tuesday";
case 3 -> "Wednesday";
// ...
};
System.out.println(day);
In the above example, the case expression (switch (dayOfWeek)) evaluates to the value of the dayOfWeek variable. The resulting value is then used to determine the case to execute. In this case, the Wednesday case will be executed.
Example of the yield keyword:
int dayOfWeek = 3;
String day = switch (dayOfWeek) {
case 1 -> yield "Monday";
case 2 -> yield "Tuesday";
case 3 -> yield "Wednesday";
// ...
};
System.out.println(day);
In the above example, the yield keyword is used to return a value from the switch statement. The returned value is then assigned to the day variable. In this case, the Wednesday string will be assigned to the day variable.
Example of multiple case labels:
int dayOfWeek = 3;
String day = switch (dayOfWeek) {
case 1, 7 -> yield "Weekend";
case 2, 3, 4, 5, 6 -> yield "Weekday";
default -> yield "Invalid day of week";
};
System.out.println(day);
In the above example, the switch statement has two cases with multiple case labels. The first case will be executed if the dayOfWeek variable is equal to 1 or 7. The second case will be executed if the dayOfWeek variable is equal to 2, 3, 4, 5, or 6.
Example of arrow syntax:
int dayOfWeek = 3;
String day = switch (dayOfWeek) {
case 1 -> "Monday";
case 2 -> "Tuesday";
case 3 -> "Wednesday";
// ...
};
System.out.println(day);
The above code example is equivalent to the following code example, which uses the traditional switch statement syntax:
int dayOfWeek = 3;
String day;
switch (dayOfWeek) {
case 1: day = "Monday";
break;
case 2: day = "Tuesday";
break;
case 3: day = "Wednesday";
break;
// ...
}
System.out.println(day);
The arrow syntax is simply a more concise and expressive way to write the same code.
Conclusion
The Java Switch statement is a powerful tool in a programmer's toolkit, offering an efficient and organized way to make decisions in your code. By understanding its syntax, usage, and when to apply it in place of if-else constructs, you can write more concise, readable, and maintainable code. Whether you're handling menu-driven programs, working with enumerations, or optimizing performance, the Java Switch statement provides a versatile and elegant solution to a wide range of programming challenges.
Comments