What is "Render Props" in React.JS?
- The Tech Platform
- Apr 26, 2022
- 3 min read
Props in React are properties that are passed from a parent component to a child component. Basically, props are plain JavaScript objects. Unlike the state, which is managed within the component, props are received as input from another component. As mentioned, props are JavaScript objects. But props are not limited to this only. There is another way of using props and it is known as “render props”. In this article, we will discuss what “render props” are and how to use this technique in React with the help of examples.
What is “render props” in React.js?
“Render props” is a technique using which code can be shared among the components. In simple words, passing functions as props from a parent component to a child component is known as “render props”.
Let’s understand with the help of a simple example.
const ChildComp = (props) => {
console.log(props);
return <> </>;
};
export default function App() {
const sayHi = () => {
alert("Hi there!");
};
return <ChildComp data={sayHi} />;
}
In the above code, “ChildComp” is rendered in the “App” component. “sayHi” is a function in the “App” component and it is passed as props to the “ChildComp”.
<ChildComp data={sayHi} />;
Now, let’s see what is the value of props in the “ChildComp”.

The value of props in the “ChildComp” is of course “data” and “data” contains the “sayHi” function. So this is how functions are passed from one component to another. Let’s understand “render props” with the help of a working example.
Working with “render props”
Suppose, we need to create a counter in React. The app will contain two buttons, one for incrementing the value by one and the other for decreasing the value by one. Now, here's a twist. The state and the displayed value on the UI should be in the parent component while the buttons should be in the child component. How to start? Well, there are different ways but we will use render props! Observe the “ParentComp” Component.
import { useState } from "react";
const ParentComp = () => {
const [count, setCount] = useState(0);
const incrementHandler = () => {
setCount(count + 1);
};
const decrementHandler = () => {
setCount(count - 1);
};
return (
<>
<ChildComp />
<h2>{count}</h2>
</>
);
};
export default ParentComp;
The state is defined in the “ParentComp” using the useState React hook and two functions - “incrementHandler” and “decrementHandler”. Moreover, the state, i.e. “count” is being rendered in the <h2> tag along with the “ChildComp”. Now, observe the “ChildComp”.
const ChildComp = () => {
return (
<>
<button>Increment</button>
<button>Decrement</button>
</>
);
};
export default ChildComp;
Both the buttons are in this component. As of now, nothing happens when either of the buttons is clicked. This is how the UI looks with some additional CSS.:
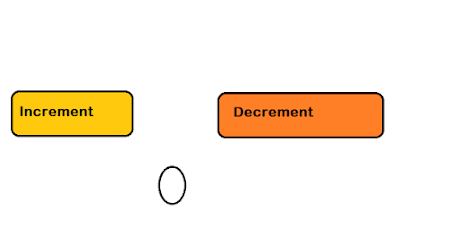
Now, we need to invoke those two functions on the button clicks. How to do it? We will do it by using “render props''. We will pass them as props from the “ParentComp” to the “ChildComp”.
import { useState } from "react";
import ChildComp from "./ChildComp";
const ParentComp = () => {
const [count, setCount] = useState(0);
const incrementHandler = () => {
setCount(count + 1);
};
const decrementHandler = () => {
setCount(count - 1);
};
return (
<>
<ChildComp increment={incrementHandler} decrement={decrementHandler} />
<h2>{count}</h2>
</>
);
};
export default ParentComp;
Observe the “ChildComp” that is rendered in the “ParentComp”.
<ChildComp increment={incrementHandler} decrement={decrementHandler} />;
Now, we can access “incrementHandler” and “decrementHandler” as “increment” and “decrement” respectively in the “ChildComp” using props.
const ChildComp = (props) => {
return (
<>
<button onClick={props.increment}>Increment</button>
<button onClick={props.decrement}>Decrement</button>
</>
);
};
export default ChildComp;
Whenever any button will be clicked, the specified function will be called even though the function is not present in the “ChildComp”. This is the power of “render props”.

Conclusion
“render props” is a simple but powerful and useful technique to work with functions in React. Generally, a React app has several components, and there might arise a situation where functions need to be shared among them, as we discussed in the example above. So the render props technique can be used to share code or functions among components in a simple way.
Resource: Java67
The Tech Platform
Comments