What is the JavaScript Map function?
- The Tech Platform
- Jul 16, 2021
- 6 min read
Updated: Jun 19, 2023
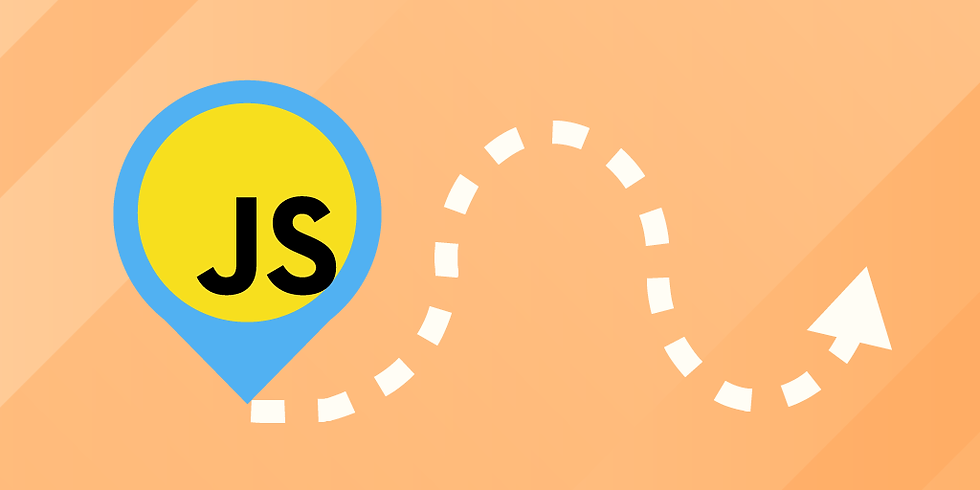
JavaScript used to be limited in its collection capabilities. While other programming languages offered sets, associative maps, lists, and dictionaries, JavaScript only provided arrays as a built-in collection type. However, JavaScript developers devised a workaround to map keys to values using objects. Nevertheless, this approach had its own limitations.
With the release of ECMAScript 6 (ES6), JavaScript introduced a new built-in class called Map, which greatly enhanced the language's collection capabilities. In this tutorial, we will explore JavaScript Map with code examples, cover its methods, demonstrate use cases, and introduce advanced concepts for further learning.
What is a JavaScript Map function?
A JavaScript Map function is a built-in collection type introduced in the ES6 release. Before ES6, JavaScript developers often used objects to map keys to values, but this approach had certain limitations. With objects,
There was no reliable way to iterate over keys
The keys() method would convert fields to strings leading to the potential key collision
Adding new keys and values was not straightforward
To address these issues, the ES6 release introduced the Map collection type in JavaScript. A Map can store key-value pairs of any type and maintains the insertion order of keys. It allows any value, whether objects or primitives, to be used as keys or values.
The Map in JavaScript creates a new array that contains the results of iterating over the elements of the array and calling the provided function once for each element, in order. It is an important data structure with various essential uses.
It's important to note that JavaScript also has another similar collection type called WeakMap, but all keys in a WeakMap must be objects.
To create a new Map, you can use the following syntax:
let map = new Map([iterable]);
For example, let's say we want to create a Map that stores names as keys and scores as values:
'use strict';
const scores = new Map([['Rahul', 10], ['Steven', 12], ['Sam', 13], ['Robin', 18]]);
scores.set('Jason', 12);
console.log(scores.size);
The above code initializes a Map named scores with initial key-value pairs. The keys represent names, and the values represent scores. After that, a new key-value pair is added to the scores map using the set() method, with the key 'Jason' and the value 12. Finally, the console.log() statement outputs the size of the scores map using the size property, which returns the number of key-value pairs in the map. In this case, the output will be 5 because there are five key-value pairs in the scores map.
Output:
5
JavaScript Map offers many more functionalities, including:
Iterating through maps: You can use loops or the forEach() method to iterate over key-value pairs in a Map.
Initializing maps with iterable objects: You can pass an iterable object, such as an array, to the Map constructor to initialize the map.
Getting elements from maps by key: The get() method allows you to retrieve the value associated with a specific key.
Getting the number of elements in a map: The size property returns the number of key-value pairs in a Map.
Converting map keys or values to arrays: The keys() and values() methods return iterable objects that can be converted to arrays.
Invoking callbacks for each key-value pair in insertion order: The forEach() method allows you to execute a callback function for each key-value pair, maintaining the insertion order.
JavaScript Map Syntax
This JavaScript map syntax is as follows:

Let's break down the syntax components:
array: The array on which the map method is called.
map: The method called for the array with the required parameters.
function(currentValue, index, arr): The function to be executed for each element in the array.
currentValue: The value of the current element being processed.
index: The index of the current element being processed.
arr: The array object on which the map method is called.
thisValue (optional): The value used as the this value when the function is executed.
Example of Implementation of map() Function:
Example Implementation of map() Function:
// Creating an array
var an_array = [5, 3, 1, 4, 2];
// The map() method calls a function with "item" as the parameter
// It passes each element of the "an_array" as "item" to the function
// The function triples each element and returns it as the result
var result = an_array.map(function(item)
{
return item * 3;
});
// Printing the new array with the tripled values
console.log(result);
Output: `[ 15, 9, 3, 12, 6 ]`
In the example above, the map() method is called on the an_array. The provided function is applied to each element of the array, tripling the value of each element. The resulting array [15, 9, 3, 12, 6] is then printed to the console.
JavaScript Map Methods
Now that we know the JavaScript map syntax and walked through an example implementation, let’s discuss some commonly used map methods along with their use cases.
Method 1. Initialize a Map with an Iterable Object: The Map() constructor initializes a map with an iterable object. The iterable object should contain arrays representing key-value pairs.
let userRoles = new Map([
['ruby', 'director'],
['steven', 'producer'],
['foo', 'writer'],
['robin', 'actress']
]);
Method 2. Return a Value Mapped to a Specific Key: The get() method returns a value mapped to a specific key. If the key is not present, it returns undefined.
userRoles.get('robin'); // Output: 'actress'
Method 3. Check if a Specified Key Exists in the Map: The has() method returns a boolean indicating whether a specified key exists in the map.
userRoles.has('ruby'); // Output: true
Method 4. Return the Number of Entries in the Map: The size property returns the number of entries (key-value pairs) in the map.
console.log(userRoles.size);
// Output: 4
Method 5. Return an Iterator Object with the Values of Each Element: The values() method returns an iterator object that includes the values of each element in the map.
for (let role of userRoles.values()) {
console.log(role);
}
// Output:
// 'director'
// 'producer'
// 'writer'
// 'actress'
Method 6. Remove Specified Elements from the Map: The delete() method removes specified elements from the map object.
userRoles.delete('ruby');
Method 7. Remove All Elements from the Map: The clear() method removes all elements from the map object.
userRoles.clear();
Other Important Map Methods
JavaScript Maps provide several other important methods that offer additional functionality. Let's explore these methods in detail along with code examples:
Method 1. forEach(callback[, thisArg]):
The forEach() method invokes a callback function for each key-value pair in the map, in the order of insertion. It allows you to perform an action or operation on each element of the map.
let userRoles = new Map([
['ruby', 'director'],
['steven', 'producer'],
['foo', 'writer'],
['robin', 'actress']
]);
userRoles.forEach((value, key) => {
console.log(`${key}: ${value}`);
});
Output:
ruby: director
steven: producer
foo: writer
robin: actress
In the example above, the forEach() method is used to iterate over each key-value pair in the userRoles map. The callback function is executed for each entry, printing the key-value pair to the console.
Method 2. set(key, value):
The set() method is used to set a value for a specific key in the map object. It allows you to add or update entries in the map.
let userRoles = new Map();
userRoles.set('ruby', 'director');
userRoles.set('steven', 'producer');
userRoles.set('foo', 'writer');
console.log(userRoles);
Output:
Map(3) {
'ruby' => 'director',
'steven' => 'producer',
'foo' => 'writer'
}
In the example above, the set() method is used to add key-value pairs to the userRoles map. Each call to set() sets a value for a specific key.
Method 3. keys():
The keys() method returns a new iterator containing the keys of the elements in the map, in the order of insertion. It allows you to retrieve all the keys from the map.
let userRoles = new Map([
['ruby', 'director'],
['steven', 'producer'],
['foo', 'writer'],
['robin', 'actress']
]);
let keys = userRoles.keys();
for (let key of keys) {
console.log(key);
}
Output:
ruby
steven
foo
robin
In the example above, the keys() method is used to obtain an iterator containing the keys of the userRoles map. The for...of loop is then used to iterate over the keys and print them to the console.
Method 4. entries():
The entries() method returns a new iterator that iterates over a collection of key-value pairs in the map, in the order of insertion. It allows you to access both keys and values together.
let userRoles = new Map([
['ruby', 'director'],
['steven', 'producer'],
['foo', 'writer'],
['robin', 'actress']
]);
let entries = userRoles.entries();
for (let [key, value] of entries) {
console.log(`${key}: ${value}`);
}
Output:
ruby: director
steven: producer
foo: writer
robin: actress
In the example above, the entries() method is used to obtain an iterator that iterates over the key-value pairs in the userRoles map. The for...of loop is used to extract both the key and value from each entry and print them to the console.
Advanced JavaScript Map Concepts
JavaScript Map is an extremely valuable collection type that brings significant benefits to programming with JavaScript. It provides a cleaner and more efficient approach to working with key-value pairs and collections of data.
Throughout this tutorial, we have covered map syntax, various methods, and common use cases. By understanding these fundamentals, you are now equipped to dive into more advanced concepts that will enhance your JavaScript skills. Here are some recommended concepts to explore next:
Metaprogramming: Metaprogramming involves writing code that can manipulate other code at runtime. Maps can be used in metaprogramming scenarios where you dynamically store and retrieve information about objects, functions, or properties.
Prototypal Inheritance: Maps can be utilized to implement prototypal inheritance patterns, where you can associate parent objects with their child objects and access them efficiently.
Implementing Constructors: By using Maps, you can implement custom constructors that store key-value pairs representing object properties and values.
Literals: JavaScript Maps can also be used to create complex object literals by storing and retrieving data with descriptive keys.
Remember to experiment, practice, and seek additional resources to further solidify your knowledge and skills in JavaScript development. Happy coding!
Comments