Top 10 lesser-known useful NPM packages
- The Tech Platform
- Dec 22, 2021
- 6 min read
Node.js applications benefit from more than a million open-source packages available in the NPM package registry. Most popular packages get well over 10 million weekly downloads and are at the foundation of many applications, from small pet projects to well-known tech startups. Today, 97 percent of code in modern web applications comes from npm modules.
We’ll briefly cover the lesser-known useful ones that will save you from reinventing the wheel.
1. quick-validate
Quick configurable API validations for Express.js
Features:
Simple — Provides a simple and quick way to configure API validations.
Clean — Unlike other libraries, quick-validate does not require you to write a lot of code for adding simple validations. It uses simple JSON configuration files which do not pollute application code.
Readable — The validations are much more readable and comprehensible.
Installation
npm install quick-validate --save
Usage
Create a directory named validations inside root of your project. This is not required but is recommended as a good practice.
Create 2 files inside the validations directory - apiValidations.json and validationSchema.json
2. mquery
mquery is a fluent mongodb query builder designed to run in multiple environments.
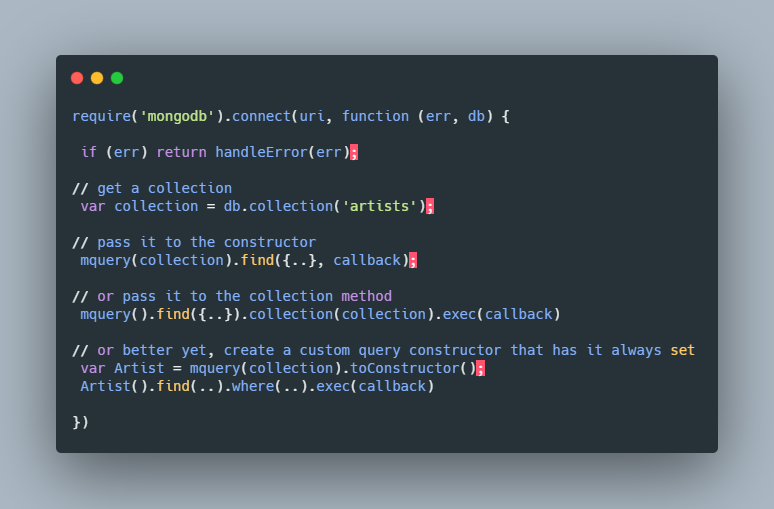
Features:
fluent query builder api
custom base query support
MongoDB 2.4 geoJSON support
method + option combinations validation
node.js driver compatibility
environment detection
debug support
separated collection implementations for maximum flexibility
Use
require('mongodb').connect(uri, function (err, db)
{
if (err) return handleError(err);
// get a collection
var collection = db.collection('artists');
// pass it to the constructor
mquery(collection).find({..}, callback);
// or pass it to the collection method
mquery().find({..}).collection(collection).exec(callback)
// or better yet, create a custom query constructor that has it always
set
var Artist = mquery(collection).toConstructor();
Artist().find(..).where(..).exec(callback)
})
mquery requires a collection object to work with. In the example above we just pass the collection object created using the official MongoDB driver.
#3 Luxon
Luxon is a library for working with dates and times in JavaScript.
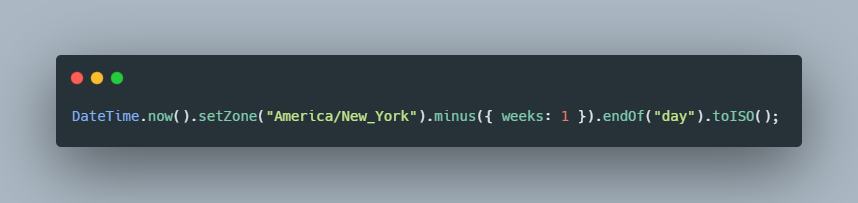
Luxon makes a major break in API conventions. Part of Moment’s charm is that you just call moment() on basically anything and you get date, whereas Luxon forces you to decide that you want to call fromISO or whatever. The upshot of all that is that Luxon feels like a different library; that’s why it’s not Moment 3.0.
Download/install
Documentation
General documentation
API docs
Quick tour
For Moment users
Why does Luxon exist?
A quick demo
#4 Helmet
Helmet helps you secure your Express apps by setting various HTTP headers. It’s not a silver bullet, but it can help!
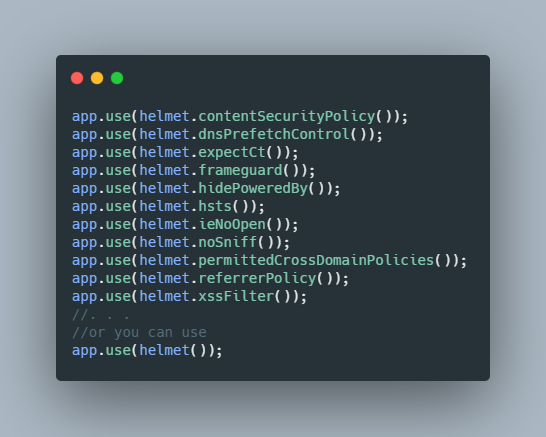
Quick start
First, run npm install helmet --save for your app. Then, in an Express app:
const express = require("express");
const helmet = require("helmet");
const app = express();
app.use(helmet());
// ...
How it works
Helmet is Connect-style middleware, which is compatible with frameworks like Express. (If you need support for Koa, see koa-helmet.)
The top-level helmet function is a wrapper around 15 smaller middlewares, 11 of which are enabled by default.
In other words, these two things are equivalent:
// This...
app.use(helmet());
// ...is equivalent to this:
app.use(helmet.contentSecurityPolicy());
app.use(helmet.dnsPrefetchControl());
app.use(helmet.expectCt());
app.use(helmet.frameguard());
app.use(helmet.hidePoweredBy());
app.use(helmet.hsts());
app.use(helmet.ieNoOpen());
app.use(helmet.noSniff());
app.use(helmet.permittedCrossDomainPolicies());
app.use(helmet.referrerPolicy());
app.use(helmet.xssFilter());
To set custom options for one of the middleware, add options like this:
// This sets custom options for the `referrerPolicy` middleware.
app.use(
helmet({
referrerPolicy: {
policy: "no-referrer" },
}
)
);
You can also disable a middleware:
// This disables the `contentSecurityPolicy` middleware but keeps the rest.app.use(
helmet({
contentSecurityPolicy: false,
})
);
#5 cors
CORS is a node.js package for providing a Connect/Express middleware that can be used to enable CORS with various options.
Installation
This is a Node.js module available through the npm registry. Installation is done using the npm install command:
$ npm install cors
Usage
1. Simple Usage (Enable All CORS Requests)
var express = require('express')
var cors = require('cors')
var app = express()
app.use(cors())
app.get('/products/:id', function (req, res, next) {
res.json({msg: 'This is CORS-enabled for all origins!'})
})
app.listen(80, function () {
console.log('CORS-enabled web server listening on port 80')
})
2. Enable CORS for a Single Route
var express = require('express')
var cors = require('cors')
var app = express()
app.get('/products/:id', cors(), function (req, res, next) {
res.json({msg: 'This is CORS-enabled for a Single Route'})
})
app.listen(80, function () {
console.log('CORS-enabled web server listening on port 80')
})
3. Configuring CORS
var express = require('express')
var cors = require('cors')
var app = express()
var corsOptions = {
origin: 'http://example.com',
optionsSuccessStatus: 200 // some legacy browsers (IE11, various SmartTVs) choke on 204
}
app.get('/products/:id', cors(corsOptions), function (req, res, next) {
res.json({msg: 'This is CORS-enabled for only example.com.'})
})
app.listen(80, function () {
console.log('CORS-enabled web server listening on port 80')
})
4. Configuring CORS w/ Dynamic Origin
var express = require('express')
var cors = require('cors')
var app = express()
var whitelist = ['http://example1.com', 'http://example2.com']
var corsOptions = {
origin: function (origin, callback) {
if (whitelist.indexOf(origin) !== -1) {
callback(null, true)
} else {
callback(new Error('Not allowed by CORS'))
}
}
}
app.get('/products/:id', cors(corsOptions), function (req, res, next) {
res.json({msg: 'This is CORS-enabled for a whitelisted domain.'})
})
app.listen(80, function () {
console.log('CORS-enabled web server listening on port 80')
})
If you do not want to block REST tools or server-to-server requests, add a !origin check in the origin function like so:
var corsOptions = {
origin: function (origin, callback) {
if (whitelist.indexOf(origin) !== -1 || !origin) {
callback(null, true)
} else {
callback(new Error('Not allowed by CORS'))
}
}
}
5. Enabling CORS Pre-Flight
Certain CORS requests are considered 'complex' and require an initial OPTIONS request (called the "pre-flight request"). An example of a 'complex' CORS request is one that uses an HTTP verb other than GET/HEAD/POST (such as DELETE) or that uses custom headers. To enable pre-flighting, you must add a new OPTIONS handler for the route you want to support:
var express = require('express')
var cors = require('cors')
var app = express()
app.options('/products/:id', cors()) // enable pre-flight request for DELETE request
app.del('/products/:id', cors(), function (req, res, next) {
res.json({msg: 'This is CORS-enabled for all origins!'})
})
app.listen(80, function () {
console.log('CORS-enabled web server listening on port 80')
})
You can also enable pre-flight across-the-board like so:
app.options('*', cors()) // include before other routes
6. Configuring CORS Asynchronously
var express = require('express')
var cors = require('cors')
var app = express()
var whitelist = ['http://example1.com', 'http://example2.com']
var corsOptionsDelegate = function (req, callback) {
var corsOptions;
if (whitelist.indexOf(req.header('Origin')) !== -1) {
corsOptions = { origin: true } // reflect (enable) the requested origin in the CORS response
} else {
corsOptions = { origin: false } // disable CORS for this request
}
callback(null, corsOptions) // callback expects two parameters: error and options
}
app.get('/products/:id', cors(corsOptionsDelegate), function (req, res, next) {
res.json({msg: 'This is CORS-enabled for a whitelisted domain.'})
})
app.listen(80, function () {
console.log('CORS-enabled web server listening on port 80')
})
#6 colors
Get color and style in your node.js console
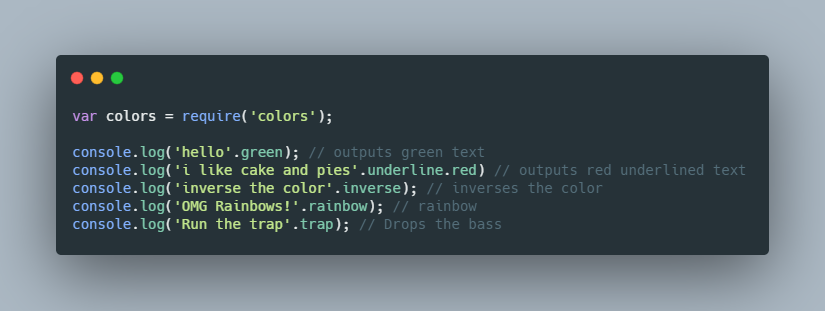
Installation
npm install colors
colors and styles!
1. text colors
black
red
green
yellow
blue
magenta
cyan
white
gray
grey
2. bright text colors
brightRed
brightGreen
brightYellow
brightBlue
brightMagenta
brightCyan
brightWhite
3. background colors
bgBlack
bgRed
bgGreen
bgYellow
bgBlue
bgMagenta
bgCyan
bgWhite
bgGray
bgGrey
4. bright background colors
bgBrightRed
bgBrightGreen
bgBrightYellow
bgBrightBlue
bgBrightMagenta
bgBrightCyan
bgBrightWhite
5. styles
reset
bold
dim
italic
underline
inverse
hidden
strikethrough
6. extras
rainbow
zebra
america
trap
random
#7 got
Human-friendly and powerful HTTP request library for Node.js
Highlights: -Promise API -Stream API -Pagination API -HTTP2 support -Request cancelation -Follows redirects -Retries on failure -Progress events -Handles gzip/deflate/brotli -Timeout handling -Errors with metadata -JSON mode -Hooks -Instances with custom defaults
Install
$ npm install got
Take a peek
JSON mode
Got has a dedicated option for handling JSON payload.
Furthermore, the promise exposes a .json<T>() function that returns Promise<T>.
import got from 'got';
const {data} = await got.post('https://httpbin.org/anything',
{
json:
{
hello: 'world'
}
}).json();
console.log(data);
//=> {"hello": "world"}
For advanced JSON usage, check out the parseJson and stringifyJson options.
#8 express-brute
A brute-force protection middleware for express routes that rate-limits incoming requests, increasing the delay with each request in a fibonacci-like sequence.
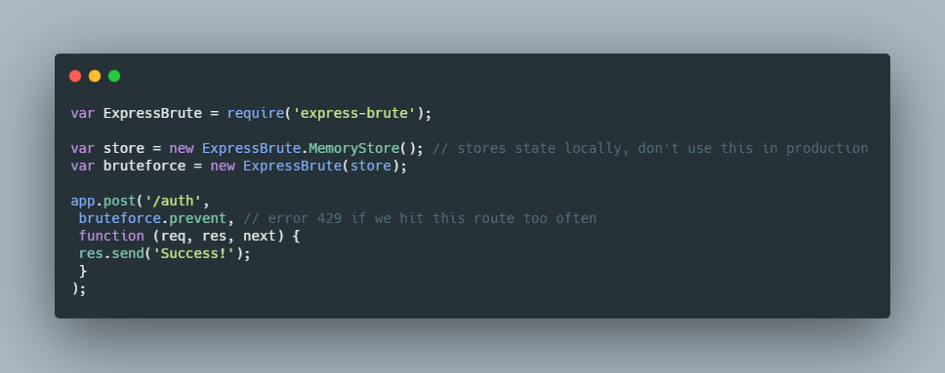
Installation
npm install express-mongo-sanitize
Usage
Add as a piece of express middleware, before defining your routes.
const express = require('express');const bodyParser = require('body-parser');const mongoSanitize = require('express-mongo-sanitize');const app = express();app.use(bodyParser.urlencoded({ extended: true }));app.use(bodyParser.json());// To remove data, use:app.use(mongoSanitize());// Or, to replace prohibited characters with _, use:app.use(mongoSanitize({replaceWith: '_',}),);
onSanitize
onSanitize callback is called after the request's value was sanitized.
app.use(mongoSanitize({onSanitize: ({ req, key }) => {console.warn(`This request[${key}] is sanitized`, req);},}),);
dryRun
You can run this middleware as dry run mode.
app.use(mongoSanitize({dryRun: true,onSanitize: ({ req, key }) => {console.warn(`[DryRun] This request[${key}] will be sanitized`, req);},}),);
Node Modules API
You can also bypass the middleware and use the module directly:
const mongoSanitize = require('express-mongo-sanitize');const payload = {...};// Remove any keys containing prohibited charactersmongoSanitize.sanitize(payload);// Replace any prohibited characters in keysmongoSanitize.sanitize(payload, {replaceWith: '_'});// Check if the payload has keys with prohibited charactersconst hasProhibited = mongoSanitize.has(payload);
#9 xss
Sanitize untrusted HTML (to prevent XSS) with a configuration specified by a Whitelist.
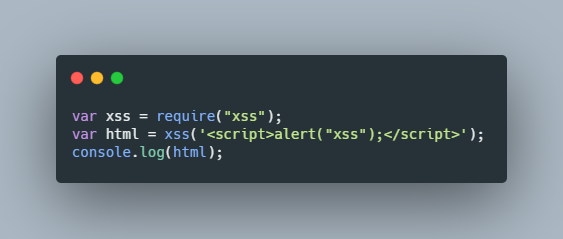
Features
Specifies HTML tags and their attributes allowed with whitelist
Handle any tags or attributes using custom function.
Reference
XSS Filter Evasion Cheat Sheet
Data URI scheme
XSS with Data URI Scheme
#10 express-mongo-sanitize
Express 4.x middleware which sanitizes user-supplied data to prevent MongoDB Operator Injection.
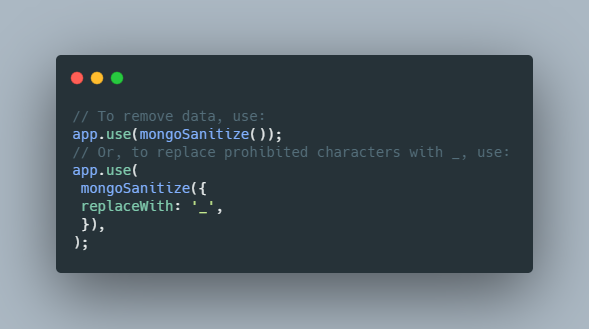
Installation
via npm:
$ npm install express-brute
A Simple Example
var ExpressBrute = require('express-brute');
var store = new ExpressBrute.MemoryStore(); // stores state locally, don't use this in production
var bruteforce = new ExpressBrute(store);
app.post('/auth',
bruteforce.prevent, // error 429 if we hit this route too often
function (req, res, next) {
res.send('Success!');
}
);
Final Words:
This was our list of the top lesser-known NPM packages that coders and web developers can give a shot.
Before summarizing, we must tell you that don’t go by the popularity of the NPM packages. Instead, go by your personal requirements.
Source: Medium - Akash Saggu
The Tech Platform
コメント