Repository Pattern with Entity framework in ASP.NET Core 6.0
- The Tech Platform
- Dec 27, 2021
- 1 min read
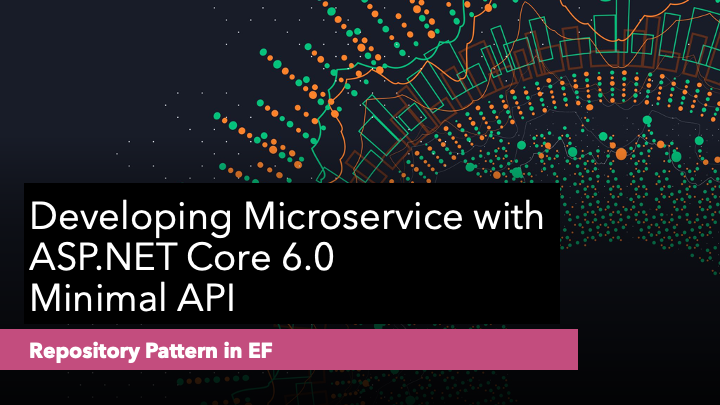
Repository pattern is quite famous pattern in .NET world while accessing backend data using ORM (Object-Relational Mapping) system such as Entity framework. You can use any ORM based system like
Dapper
Venflow
DevExpress XPO
Having an Repository pattern will create an abstraction from the ORM itself.
Repository pattern can help insulate our application from changes in the data store and can facilitate automated unit testing or test-driven development
Creating Repository Pattern
The first step in creating repository pattern is to create an Interface with all the methods declaration used by your application data access layer with ORM. In our case, we are using Entity framework and we need to access data from SQL Server. So, the code will look something like this
using System.Collections.Generic;
namespace MiniDemo.Model
{
public interface IDataRepository
{
List<Employee> AddEmployee(Employee employee);
List<Employee> GetEmployees();
Employee PutEmployee(Employee employee);
Employee GetEmployeeById(string id);
}
}
And the implementation of the interface code will look as shown below
public class DataRepository : IDataRepository
{
private readonly EmployeeDbContext db;
public DataRepository(EmployeeDbContext db)
{
this.db = db;
}
public List<Employee> GetEmployees() => db.Employee.ToList();
public Employee PutEmployee(Employee employee)
{
db.Employee.Update(employee);
db.SaveChanges();
return db.Employee.Where(x => x.EmployeeId == employee.EmployeeId)
.FirstOrDefault();
}
public List<Employee> AddEmployee(Employee employee)
{
db.Employee.Add(employee);
db.SaveChanges();
return db.Employee.ToList();
}
public Employee GetEmployeeById(string Id)
{
return db.Employee.Where(x => x.EmployeeId == Id).FirstOrDefault();
}
Adding to DI service container
Finally, we need to add the Dependency Injection in Service container
builder.Services.AddScoped<IDataRepository, DataRepository>();
Complete Video
Here is the complete video of the above article
Source: Medium - ExecuteAutomation
The Tech Platform
Comments