Q# Programming Language
- The Tech Platform
- Apr 9, 2021
- 3 min read
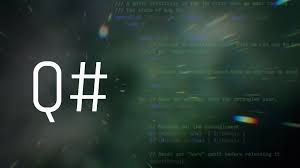
Q# (pronounced as Q sharp) is a domain-specific programming language used for expressing quantum algorithms. It was initially released to the public by Microsoft as part of the Quantum Development Kit.
A Q# program recombines these operations as defined by a target machine to create new, higher-level operations to express quantum computation. In this way, Q# makes it easy to express the logic underlying quantum and hybrid quantum–classical algorithms, while also being general with respect to the structure of a target machine or simulator.
Syntax:
A simple example is the following program, which allocates one qubit in the |0⟩|0⟩ state, then applies a Hadamard operation H to it and measures the result in the PauliZ basis.
Example:
@EntryPoint()
operation MeasureOneQubit() : Result {
// The following using block creates a fresh qubit and initializes it
// in the |0〉 state.
use qubit = Qubit();
// We apply a Hadamard operation H to the state, thereby preparing the
// state 1 / sqrt(2) (|0〉 + |1〉).
H(qubit);
// Now we measure the qubit in Z-basis.
let result = M(qubit);
// As the qubit is now in an eigenstate of the measurement operator,
// we reset the qubit before releasing it.
if result == One
{
X(qubit);
}
// Finally, we return the result of the measurement.
return result;
}
History:
During a Microsoft Ignite Keynote on September 26, 2017, Microsoft announced that they were going to release a new programming language geared specifically towards quantum computers. On December 11, 2017, Microsoft released Q# as a part of the Quantum Development Kit.
At Build 2019, Microsoft announced that it is open-sourcing the Quantum Development Kit, including its Q# compilers and simulators.
Uses:
Q# is available as a separately downloaded extension for Visual Studio, but it can also be run as an independent tool from the Command line and/or Visual Studio Code. The Quantum Development Kit ships with a quantum simulator which is capable of running Q#.
In order to invoke the quantum simulator, another .NET programming language, usually C#, is used, which provides the (classical) input data for the simulator and reads the (classical) output data from the simulator.
Features:
A primary feature of Q# is the ability to create and use qubits for algorithms. As a consequence, some of the most prominent features of Q# are the ability to entangle and introduce superpositioning to qubits via Controlled NOT gates and Hadamard gates, respectively, as well as Toffoli Gates, Pauli X, Y, Z Gate, and many more which are used for a variety of operations; see the list at the article on quantum logic gates.
The hardware stack that will eventually come together with Q# is expected to implement Qubits as topological qubits. The quantum simulator that is shipped with the Quantum Development Kit today is capable of processing up to 32 qubits on a user machine and up to 40 qubits on Azure.
Similarities with C#
Uses namespace for code isolation
All statements end with a ;
Curly braces are used for statements of scope
Single line comments are done using //
Variable data types such as Int Double String and Bool are similar, although capitalised (and Int is 64-bit)
Qubits are allocated and disposed inside a using block.
Lambda functions using the => operator.
Results are returned using the return keyword.
Similarities with F#
Variables are declared using either let or mutable
First-order functions
Modules, which are imported using the open keyword
The datatype is declared after the variable name
The range operator ..
for … in loops
Every operation/function has a return value, rather than void. Instead of void, an empty Tuple () is returned.
Definition of record datatypes (using the newtype keyword, instead of type).
Differences
Functions are declared using the function keyword
Operations on the quantum computer are declared using the operation keyword
Lack of multiline comments
Asserts instead of throwing exceptions
Documentation is written in Markdown instead of XML-based documentation tags
Source: Wikipedia
The Tech Platform
Comments