Performance Improvements in .NET 6
- The Tech Platform
- Mar 2, 2023
- 7 min read
Performance improvement in .NET 6 refers to the various enhancements made to the .NET Framework that improve the performance of applications built with it. These improvements include faster garbage collection, improved Just-In-Time (JIT) compiler, SIMD improvements, WebAssembly support, tiered compilation, and improved HTTP/HTTPS performance, among others.
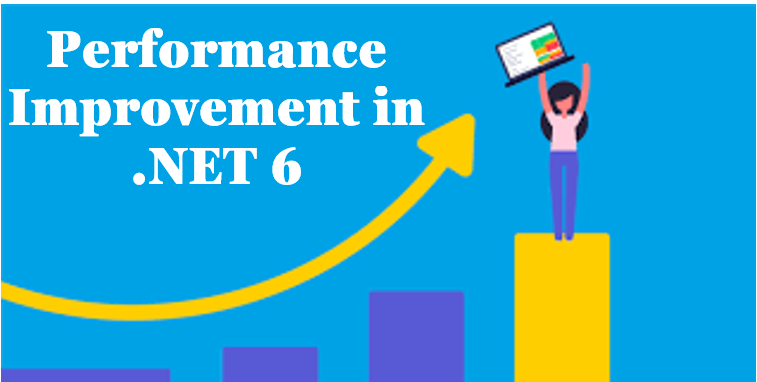
Performance improvement in .NET 6 is required because modern applications demand high performance to meet the expectations of end-users. Performance is a critical factor that affects the user experience of an application. Applications that are slow and unresponsive can lead to frustration, decreased productivity, and a negative impact on the user's perception of the application and the organization that developed it.
Improving performance in .NET 6 allows developers to build applications that are faster and more responsive, which can lead to a better user experience and increased user engagement. Faster applications also help increase the productivity of users and reduce the risk of user abandonment. Additionally, improved performance can help reduce the infrastructure costs associated with running an application by reducing the number of resources required to achieve the same level of performance.
Improve Performance in .NET 6
Here are some techniques you can use to improve performance in .NET 6:
Use Async/Await
Avoid Boxing and Unboxing
Use LINQ
Use StringBuilder
Use the Using Statement
Use Caching
Use Parallel processing
Use lazy loading
Use dependency injection
Use value types
1. Use Async/Await:
Async/await is a technique for writing asynchronous code that can improve the performance of your application by allowing it to continue executing while waiting for I/O operations to complete. In .NET 6, you can use async/await to improve the performance of your application.
using System;
using System.Threading.Tasks;
public class AsyncService
{
public async Task<int> LongRunningOperationAsync()
{
await Task.Delay(1000); // Simulate a long running operationreturn 42;
}
}
public class MainClass
{
public async Task Main()
{
var service = new AsyncService();
var result = await service.LongRunningOperationAsync();
Console.WriteLine(result);
}
}
In this code, we create an AsyncService class with a LongRunningOperationAsync method that simulates a long running operation by waiting for one second. We also create a MainClass with a Main method that calls the LongRunningOperationAsync method of AsyncService using the await keyword. This allows the application to continue executing while the LongRunningOperationAsync method is waiting for the one second delay to complete.
2. Avoid Boxing and Unboxing:
Boxing and unboxing are operations that can have a negative impact on the performance of your application. Boxing is the process of converting a value type to an object type, while unboxing is the process of converting an object type back to a value type. In .NET 6, you can avoid boxing and unboxing by using generics and value types.
using System;
using System.Collections.Generic;
public class BoxingService
{
public void DoSomethingWithInt(int value)
{
Console.WriteLine(value);
}
public void DoSomethingWithObject(object value)
{
Console.WriteLine(value);
}
}
public class MainClass
{
public void Main()
{
var service = new BoxingService();
int value = 42;
service.DoSomethingWithInt(value); // No boxingobject boxedValue = value;
service.DoSomethingWithObject(boxedValue); // Boxingint unboxedValue = (int)boxedValue;
service.DoSomethingWithInt(unboxedValue); // Unboxing
}
}
In this code, we create a BoxingService class with DoSomethingWithInt and DoSomethingWithObject methods. The DoSomethingWithInt method takes an int parameter, while the DoSomethingWithObject method takes an object parameter. We also create a MainClass with a Main method that calls these methods with an int value and a boxed int value, respectively. The boxed int value is then unboxed to call the DoSomethingWithInt method again. By avoiding boxing and unboxing, we can improve the performance of our application.
3. Use LINQ:
LINQ is a powerful feature of .NET 6 that allows you to query collections of data in a concise and efficient way. By using LINQ, you can avoid writing complex loops and conditionals, which can improve the performance of your application.
using System;
using System.Linq;
public class LINQService
{
public void DoSomethingWithNumbers(int[] numbers)
{
var result = numbers
.Where(n => n % 2 == 0)
.OrderByDescending(n => n)
.Take(3)
.ToArray();
foreach (var number in result)
{
Console.WriteLine
In this code, we create a LINQService class with a DoSomethingWithNumbers method that takes an array of integers. We use LINQ to filter the even numbers, order them in descending order, take the top 3 numbers, and convert the result to an array. Finally, we iterate over the resulting array and print each number to the console.
By using LINQ, we can write concise and efficient code that is easier to read and maintain. This can lead to improved performance and reduced development time.
4. Use StringBuilder:
String concatenation can be a slow operation, especially when concatenating large strings or when concatenating strings in a loop. In .NET 6, you can use the StringBuilder class to improve the performance of string concatenation.
using System;
using System.Text;
public class StringBuilderService
{
public string ConcatenateStrings(string[] strings)
{
var builder = new StringBuilder();
foreach (var str in strings)
{
builder.Append(str);
}
return builder.ToString();
}
}
public class MainClass
{
public void Main()
{
var service = new StringBuilderService();
var strings = new string[] { "foo", "bar", "baz" };
var result = service.ConcatenateStrings(strings);
Console.WriteLine(result);
}
}
In this code, we create a StringBuilderService class with a ConcatenateStrings method that takes an array of strings. We create a new StringBuilder instance and use a foreach loop to append each string in the array to the StringBuilder. Finally, we convert the StringBuilder to a string and return the result.
By using StringBuilder, we can concatenate strings more efficiently and avoid the performance overhead of string concatenation.
5. Use the Using Statement:
The using statement is a convenient way to ensure that an object is disposed of when it is no longer needed. In .NET 6, you can use the using statement to improve the performance of your application by avoiding memory leaks and reducing the overhead of garbage collection.
using System;
using System.IO;
public class UsingStatementService
{
public void DoSomethingWithFile(string path)
{
using (var reader = new StreamReader(path))
{
while (!reader.EndOfStream)
{
var line = reader.ReadLine();
Console.WriteLine(line);
}
}
}
}
public class MainClass
{
public void Main()
{
var service = new UsingStatementService();
var path = "data.txt";
service.DoSomethingWithFile(path);
}
}
In this code, we create a UsingStatementService class with a DoSomethingWithFile method that takes a file path. We use the using statement to create a new StreamReader instance, which will be automatically disposed of when the using block is exited. We then use a while loop to read each line of the file and print it to the console.
By using the using statement, we can ensure that resources are properly disposed of and avoid memory leaks. This can improve the performance of our application by reducing the overhead of garbage collection.
6. Use caching:
Caching is a technique that can be used to store frequently accessed data in memory for fast access. By using caching, you can reduce the number of times your application needs to access the database or perform other expensive operations. In .NET 6, caching can be implemented using the built-in MemoryCache class.
using System;
using System.Collections.Generic;
using Microsoft.Extensions.Caching.Memory;
public class CachingService
{
private readonly IMemoryCache _cache;
public CachingService(IMemoryCache cache)
{
_cache = cache;
}
public T GetFromCache<T>(string key, Func<T> func)
{
if (_cache.TryGetValue(key, out T result))
{
return result;
}
result = func();
_cache.Set(key, result, TimeSpan.FromMinutes(5));
return result;
}
}
In this code, we create a CachingService class that takes an IMemoryCache object in its constructor. The GetFromCache method takes a key and a func parameter, where key is used as a key to store the result of the func in the cache. The TryGetValue method of the IMemoryCache object is used to check if the result is already in the cache. If it is, the method returns the cached result. Otherwise, the func is executed to get the result, which is then stored in the cache using the Set method.
7. Use parallel processing
Parallel processing can be used to execute multiple tasks simultaneously. This can improve the performance of CPU-bound tasks, such as complex calculations. In .NET 6, parallel processing can be implemented using the Parallel class.
using System;
using System.Threading.Tasks;
public class ParallelProcessingService
{
public void ProcessInParallel()
{
Parallel.For(0, 10, i =>
{
// Do some CPU-bound task
});
}
}
In this code, we create a ParallelProcessingService class that has a ProcessInParallel method. This method uses the Parallel.For method to execute a CPU-bound task for each value of i between 0 and 10. The Parallel.For method automatically distributes the work among available CPU cores, improving performance.
8. Use Lazy loading
Lazy loading is a technique that allows data to be loaded on demand, rather than all at once. This can improve performance by reducing the amount of data being retrieved from the database. In .NET 6, lazy loading can be implemented using the Lazy<T> class.
using System;
using System.Collections.Generic;
public class LazyLoadingService
{
private readonly Lazy<List<string>> _data;
public LazyLoadingService()
{
_data = new Lazy<List<string>>(() =>
{
// Load data from the databasereturn new List<string>();
});
}
public List<string> GetData()
{
return _data.Value;
}
}
In this code, we create a LazyLoadingService class that has a _data field of type Lazy<List<string>>. The LazyLoadingService constructor initializes the _data field with a lambda expression that loads the data from the database. The GetData method returns the value of the _data field, which is only loaded on the first call to the method.
9. Use dependency injection
Dependency injection can be used to improve the performance of your application by reducing the amount of boilerplate code and by making it easier to manage dependencies. This can make your code easier to read, maintain, and test. In .NET 6, dependency injection can be implemented using the built-in Microsoft.Extensions.DependencyInjection package.
using System; public interface IService
{
void DoSomething();
}
public class Service : IService
{
public void DoSomething()
{
// Do something
}
}
public class DependencyInjectionService
{
private readonly IService _service;
public DependencyInjectionService(IService service)
{
_service = service;
}
public void DoSomething()
{
_service.DoSomething();
}
}
In this code, we create an IService interface and a Service class that implements it. We also create a DependencyInjectionService class that takes an IService object in its constructor. The DoSomething method of DependencyInjectionService calls the DoSomething method of the _service object. This allows us to swap out the implementation of the IService interface without changing the code in DependencyInjectionService, making it easier to manage dependencies.
10. Use value types instead of reference types
Value types are types that store their values directly, while reference types store a reference to the value. Value types can be faster to access than reference types because they don't require a dereference operation. In .NET 6, you can use value types instead of reference types to improve performance.
using System;
public struct Point
{
public int X { get; set; }
public int Y { get; set; }
public Point(int x, int y)
{
X = x;
Y = y;
}
}
public class ValueTypeService
{
public void DoSomethingWithPoint(Point point)
{
// Do something with the point
}
}
In this code, we create a Point struct that stores an X and Y coordinate. We also create a ValueTypeService class that has a DoSomethingWithPoint method that takes a Point parameter. Because Point is a value type, it is stored directly in memory, making it faster to access than a reference type.
Conclusion
By using these techniques, you can improve the performance of your .NET 6 applications. However, it's important to note that performance improvements can vary depending on the specific use case and the hardware being used. Therefore, it's important to test your application thoroughly to ensure that your performance improvements are effective.
Comments