Program to check if a variable is undefined or null in JavaScript
- The Tech Platform
- Jun 14, 2022
- 1 min read
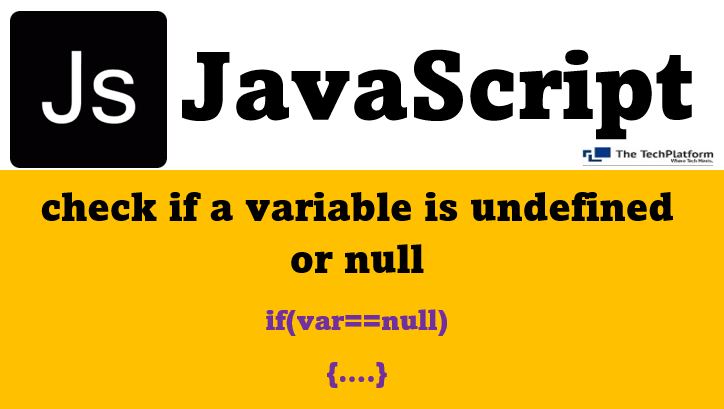
You can easily check if a variable Is Null or Undefined in JavaScript by applying if-else condition to the given variable.
Syntax:
if(var) {
....
}
If var is null then given if condition will not execute because null is a falsy value in JavaScript, but in JavaScript there are many pre-defined falsy values like
undefined
null
0
“” ( empty string)
false
NaN
The value null represents the intentional absence of any object value. It is one of JavaScript's primitive values and is treated as falsy for boolean operations.
Code:
function checkVariable(variable) {
if(variable == null) {
console.log('The variable is null');
}
else {
console.log('The variable is neither undefined nor null');
}
}
let newVariable;
checkVariable();
checkVariable('hello');
checkVariable(null);
checkVariable('12');
Output:

What is the Difference Between Null and Undefined?
Undefines
has not been assigned
typeof Undefined
undefined==null // true
Null
could be assigned
typeif object
undefined===null //false
The Tech Platform
Comments