JavaScript Tricks for Developers: Boosting Your Code Efficiency and Productivity
- The Tech Platform
- May 13, 2023
- 12 min read
In the ever-evolving landscape of web development, JavaScript remains a fundamental language that empowers developers to create dynamic and interactive experiences. Whether you're a seasoned JavaScript guru or just starting your coding journey, mastering the art of JavaScript tricks can greatly enhance your productivity and elevate the quality of your applications. In this article, we will delve into a collection of invaluable JavaScript Tricks for Developers: Boosting your Code Efficiency and Productivity that will help you to tackle coding challenges, optimize your code for performance and uncover hidden gems within the language.
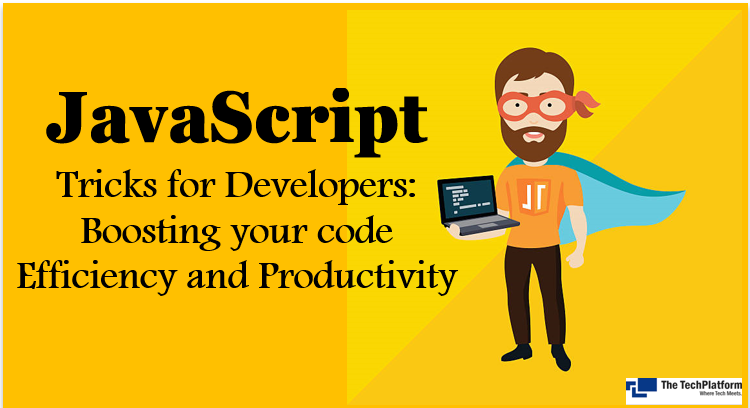
JavaScript Tricks for Developers: Boosting your Code Efficiency and Productivity
Below are some of the JavaScript Tricks for Developers which will help the development to the next level.
Tricks 1: Use Array.prototype.at() to simplify accessing elements from the end of an array.
The Array.prototype.at() method allows us to simplify accessing elements from the end of an array. Prior to this method, we typically used negative indices or calculated the index by subtracting the length of the array. However, with the at() method, we can directly specify the desired index starting from the end of the array without any additional calculations.
Here's an example that demonstrates the usage of Array.prototype.at():
const fruits = ['Apple', 'Banana', 'Cherry', 'Date', 'Blueberry'];
console.log(fruits.at(-1)); // Access the last element of the arrayconsole.log(fruits.at(-2)); // Access the second-to-last element of the array
In this code snippet, we have an array called fruits containing five elements. By using the at() method and passing a negative index as an argument, we can easily access elements from the end of the array.
The output of the above code will be:
Blueberry
Date
As you can see, fruits.at(-1) returns the last element of the fruits array, which is "elderberry", and fruits.at(-2) returns the second-to-last element, which is "date". This approach simplifies the process of accessing elements from the end of an array, making the code more concise and readable.
When to use:
Use Array.prototype.at() when you need to access an element at a specific index in an array and want to ensure that the index is within bounds.
It can be particularly useful when dealing with large arrays or when you want to avoid accessing elements that are out of range, as it provides a more controlled and safe way to access array elements.
Pros:
It provides a concise and straightforward syntax for accessing array elements at specific indices.
The at() method helps prevent errors that would occur when accessing out-of-range indices, as it throws an error if the index is invalid.
It can enhance code readability by clearly indicating the intention to access a specific element at a known index.
Cons:
The at() method is not widely supported in all JavaScript environments or older browsers. Before using it, ensure that your target environment supports it, or consider using a polyfill or alternative solution.
It is important to note that the at() method is a relatively new addition to the JavaScript language, and some developers may not be familiar with it, potentially leading to code readability issues.
In scenarios where you are confident that the index is within bounds or when handling negative indices, using the standard bracket notation (array[index]) may be more appropriate and widely understood.
Tricks 2: Use ?. (optional chaining operator) to simplify checking for null or undefined values.
The optional chaining operator (?.) in JavaScript provides a concise way to simplify checking for null or undefined values in nested object properties or function calls. Let's see how it works with an example:
// Creating a nested object with some properties
const user =
{
name: "John",
address: {
street: "123 Main Street",
city: "New York",
country: "USA"
}
};
// Accessing a property using optional chaining
const city = user.address?.city;
console.log(city);
Output:
New York
In the above code, we have an object user that contains a nested address object with various properties. We want to access the city property of the address object but want to handle the case where the address or city may be undefined.
By using the optional chaining operator (?.), we can directly access the city property without worrying about potential null or undefined values. If any intermediate property is null or undefined, the expression will short-circuit and return undefined. Otherwise, it will return the value of the property.
In the code snippet, user.address?.city uses optional chaining to check if the address property exists and then accesses the city property if it exists. If the address is undefined, the expression will evaluate to undefined and assign it to the city variable.
The final console.log(city) statement outputs the value of the city, which in this case is "New York", demonstrating the successful use of optional chaining to handle potentially missing properties without throwing an error.
When to use:
The optional chaining operator (?.) in JavaScript is useful in situations where you want to safely access properties or call methods on objects that may be nullish or undefined.
Pros:
It allows you to write cleaner and more concise code by avoiding the need for verbose nullish or undefined checks.
It helps prevent errors and exceptions that would occur if you accessed properties or called methods directly on nullish or undefined values.
It improves code readability by clearly indicating that a property or method access is optional.
Cons:
It is a relatively new feature and may not be supported in older JavaScript environments or browsers. Transpiling your code using a tool like Babel can help overcome this limitation.
It may hide errors or unintended nullish values, so it's important to use it judiciously and ensure that you handle fallback values or potential nullish scenarios appropriately.
Tricks 3: Use import() (dynamic import) to realize the on-demand loading of modules.
The import() function, also known as dynamic import allows you to dynamically load and import modules at runtime. It provides a convenient way to load modules on demand, rather than including them all upfront in the initial bundle.
To demonstrate the usage of import() for the on-demand loading of modules in JavaScript, let's create a simple example.
Suppose we have two JavaScript files: module1.js and module2.js. We want to load module2.js dynamically only when it is needed, instead of loading it upfront when the page loads. Here's how you can achieve that:
module1.js:
export function sayHello()
{
console.log('Hello from module 1!');
}
export async function loadModule2() {
const module2 = await import('./module2.js');
return module2;
}
module2.js:
export function sayGoodbye()
{
console.log('Goodbye from module 2!');
}
In module1.js, we have two exported functions. The sayHello() function simply logs a message to the console. The loadModule2() function demonstrates the dynamic loading of module2.js using the import() function. It specifies the relative path to module2.js and awaits the imported module. The imported module can then be used as needed.
Now, let's create an HTML file, index.html, that will use module1.js and dynamically load module2.js when required.
index.html:
<!DOCTYPE html>
<html>
<head>
<title>Dynamic Import Example</title>
<script type="module">import { sayHello, loadModule2 } from './module1.js';
sayHello();
// Calling a function from module1.js
// Dynamically load module2.js on button click
document.getElementById('loadButton').addEventListener('click', async () => {
const module2 = await loadModule2();
module2.sayGoodbye();
// Calling a function from module2.js
});
</script>
</head>
<body>
<h1>Dynamic Import Example</h1>
<button id="loadButton">Load Module 2</button>
</body>
</html>
In this HTML file, we first import the sayHello() function and loadModule2() function from module1.js. We then call sayHello() to verify that module1.js is functioning correctly.
Next, we add an event listener to the button with the ID loadButton. When the button is clicked, it triggers an async function that uses await to dynamically load module2.js using loadModule2(). Finally, it calls the sayGoodbye() function from module2.js.
Now, if you open index.html in a web browser and click the "Load Module 2" button, you will see the following output in the browser's console:
Hello from module 1!
Goodbye from module 2!
By using import() (dynamic import), we can selectively load JavaScript modules when needed, improving the performance of our applications by reducing initial loading times.
When to use:
Use import() when you need to load modules dynamically at runtime, especially in scenarios where the module may not be needed immediately or may depend on certain conditions.
It is useful for lazy loading modules, code splitting, or optimizing the initial loading time of your application by deferring the loading of less critical or rarely used modules.
Pros:
It helps reduce the initial bundle size of your application by loading modules on demand, improving the overall performance.
Allows for more flexible and efficient module management by loading modules based on specific conditions or user interactions.
Provides a clean and standardized way to dynamically import modules, avoiding the need for workaround techniques.
Cons:
It is not supported in older browsers or JavaScript environments. To use import(), you may need to transpile your code or use a module bundler that supports dynamic imports.
Dynamic imports add complexity to the codebase, as it introduces an asynchronous loading mechanism that needs to be handled appropriately.
Overuse of dynamic imports can make the codebase harder to understand and maintain. It's essential to use them judiciously and consider the impact on code organization and readability.
Tricks 4: Use await to simplify async functions.
await is an operator used in conjunction with async functions to pause the execution of the function until a Promise is fulfilled or rejected. It allows for writing asynchronous code in a more synchronous and readable manner.
The await keyword simplifies working with asynchronous functions. It allows you to pause the execution of an async function until a promise is resolved or rejected. Let's see how it works with a simple example:
// An asynchronous function that returns a promise after a delay
function delay(ms)
{
return new Promise(resolve => setTimeout(resolve, ms));
}
// An async function that uses the `await` keyword to simplify asynchronous code
async function fetchData()
{
console.log("Fetching data...");
await delay(2000); // Pause execution for 2000 milliseconds (2 seconds)
console.log("Data fetched!");
}
// Call the async function
fetchData();
In this example, we have an async function called fetchData that demonstrates the use of the await keyword. Inside the function, we call the delay function, which returns a promise that resolves after a specified number of milliseconds. The await keyword is used before the delay function call, allowing the function execution to pause until the promise is resolved.
When we run this code, the output will be as follows:
Fetching data...
(wait for 2 seconds)
Data fetched!
As you can see, the execution of the fetchData function pauses for 2 seconds (as specified in the delay function), simulating an asynchronous operation. Once the promise is resolved, the execution continues, and "Data fetched!" is logged to the console.
By using await in async functions, you can write asynchronous code that resembles synchronous code, making it easier to understand and maintain. It eliminates the need for callbacks or complex promise chaining, leading to cleaner and more readable code.
When to use:
Use await when you want to write asynchronous code in a more sequential and synchronous style, making it easier to read and reason about.
You can use it when working with Promises, allowing you to wait for the resolution of a Promise before proceeding with further execution.
It is useful when making asynchronous API calls, performing database operations, or any task that involves waiting for an asynchronous result.
Pros:
It simplifies asynchronous code by making it appear more like traditional synchronous code, improving readability and maintainability.
It allows for better error handling by using try/catch blocks to handle Promise rejections and exceptions.
It promotes a more straightforward and intuitive flow of control, making it easier to write and understand complex asynchronous logic.
Cons:
await can only be used inside an async function, which means you need to structure your code accordingly. This can require refactoring existing code or adjusting the program flow.
It is important to use await judiciously, as excessive use can lead to blocking and slower execution, defeating the purpose of asynchronous programming.
It is not compatible with non-Promise-based asynchronous operations or callback-based APIs without additional modifications or wrapper functions.
Tricks 5: Use JavaScript syntax (ES6+) to write more concise and readable code.
JavaScript syntax in ES6+ (ECMAScript 2015 and newer) refers to the specific rules and conventions for writing JavaScript code that incorporates the features introduced in ES6 and subsequent versions. It includes syntax elements such as arrow functions, template literals, destructuring assignments, spread syntax, classes, modules, and more. The ES6+ syntax provides a more modern and expressive way to write JavaScript code, improving code readability, modularity, and productivity.
To demonstrate how JavaScript syntax (ES6+) can make your code more concise and readable, let's look at a few examples.
1. Template Literals: Template literals are a convenient way to concatenate strings and variables, making your code more readable and reducing the need for string concatenation.
// Without template literals
const name = "Alice";
const age = 25;
console.log("My name is " + name + " and I am " + age + " years old.");
// With template literals
console.log(`My name is ${name} and I am ${age} years old.`);
Output:
My name is Alice and I am 25 years old.
2. Arrow Functions: Arrow functions provide a concise syntax for writing function expressions. They are especially useful for shorter functions and help improve code readability.
// Without arrow function
const multiply = function (a, b)
{
return a * b;
};
// With arrow function
const multiply = (a, b) => a * b;
3. Destructuring Assignment: Destructuring assignment allows you to extract values from arrays or objects into distinct variables, making your code more concise and eliminating the need for repetitive syntax.
// Without destructuring assignment
const person = { name: "John", age: 30, city: "London" };
const name = person.name;
const age = person.age;
// With destructuring assignment
const { name, age } = person;
4. Spread Syntax: The spread syntax allows you to expand arrays or objects into multiple elements, making it easier to combine or clone data structures.
// Combining arrays
const numbers1 = [1, 2, 3];
const numbers2 = [4, 5, 6];
const combined = [...numbers1, ...numbers2];
console.log(combined);
// Output: [1, 2, 3, 4, 5, 6]// Cloning objects
const original = { name: "Alice", age: 25 };
const clone = { ...original };
console.log(clone); // Output: { name: "Alice", age: 25 }
These are just a few examples of how JavaScript syntax (ES6+) can make your code more concise and readable. By utilizing these features and exploring other ES6+ improvements, you can write cleaner, more expressive code that is easier to understand and maintain.
When to use:
Use ES6+ features when developing modern JavaScript applications, as they provide more expressive and concise ways to write code. They can improve code quality, readability, and maintainability.
ES6+ is particularly beneficial in large-scale projects where code organization, modularity, and reusability are essential. Features like modules, classes, and arrow functions can simplify code structure and promote better organization and maintainability.
ES6+ features also provide performance optimizations and efficiencies, such as the use of const and let for variable declaration, which has block scope and better support for hoisting.
Pros:
Enhanced syntax and features in ES6+ make code more readable, reducing the chances of errors and improving code maintainability.
The introduction of new language constructs in ES6+ allows for more concise and expressive code, leading to increased productivity.
ES6+ provides better support for complex programming patterns and advanced techniques, such as functional programming and module bundling.
It aligns with industry best practices and is widely supported in modern JavaScript environments, enabling you to leverage the latest language features and benefits.
Cons:
Compatibility with older browsers can be a limitation, as some ES6+ features may not be supported or require transpilation. This adds complexity to the development process.
The adoption of ES6+ may require developers to learn new syntax and concepts, especially if they are not familiar with the latest JavaScript features.
Using ES6+ features excessively or inappropriately can lead to code that is harder to understand or maintain, especially if other developers on the project are not familiar with ES6+ features.
Tricks 6: Use nullish coalescing operator (??)
The nullish coalescing operator (??) is a powerful feature introduced in JavaScript that allows you to handle default values for nullish (null or undefined) values. It provides a concise and elegant way to assign a fallback value when the original value is bullish.
Let's see an example:
// Example 1
const foo = null;
const result = foo ?? 'Default Value';
console.log(result); // Output: Default Value
In this example, we have a variable foo that is assigned the value null. We then use the nullish coalescing operator (??) to assign a fallback value of 'Default Value' to the variable result. Since foo is nullish, the fallback value is used, and the output of console.log(result) will be 'Default Value'.
Here's another example where the original value is not nullish:
// Example 2
const bar = 'Hello';
const result2 = bar ?? 'Default Value';
console.log(result2); // Output: Hello
In this case, the variable bar is assigned the string 'Hello'. Since bar is not nullish, the value of bar is used, and the output of console.log(result2) will be 'Hello'.
The nullish coalescing operator (??) provides a concise and readable way to handle default values, especially when dealing with nullish values that need to be replaced. It saves you from writing more verbose conditional statements to check for nullish values.
Remember, the nullish coalescing operator only checks for nullish values (null or undefined). It does not consider falsy values such as an empty string (''), 0, NaN, or false.
By utilizing the nullish coalescing operator, you can write cleaner and more efficient code when handling default values in JavaScript.
When to use:
Use the ?? operator when you want to assign a default value to a variable if it is nullish, providing a concise alternative to longer conditional statements.
It is particularly useful when working with variables or properties that may be null or undefined, allowing you to provide a fallback value if they are.
The nullish coalescing operator helps handle cases where nullish values should be replaced with a specific default value.
Pros:
The ?? operator provides a clear and concise syntax for handling nullish values and assigning default values, reducing the need for lengthy conditional checks.
It improves code readability by explicitly indicating that a fallback value is being used in cases of nullish values.
It allows for more robust and resilient code by ensuring that nullish values are handled properly, preventing unexpected errors.
Cons:
The nullish coalescing operator is a relatively new addition to JavaScript and may not be supported in older JavaScript environments or browsers.
It only checks for nullish values (null or undefined), so it may not be suitable for cases where you need to handle other false values as fallbacks.
Overuse of the nullish coalescing operator can make code less readable if default values are assigned without proper consideration or documentation.
Conclusion
Embracing these tricks allows developers to impress with their skills and become more effective and efficient in their coding journey.
Comentários