Top 10 JavaScript Array Functions
- The Tech Platform
- Jun 30, 2022
- 3 min read
Updated: Jul 2, 2022
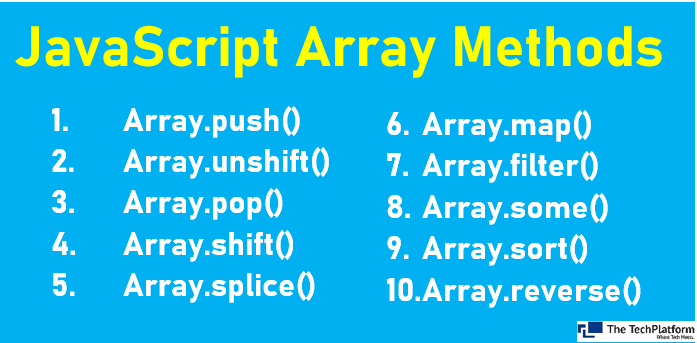
JavaScript array is an object that represents a collection of similar type of elements.
There are 3 ways to construct array in JavaScript
By array literal
By creating instance of Array directly (using new keyword)
By using an Array constructor (using new keyword)
1. Array.push() :
Adding Element at the end of an Array. As array in JavaScript are mutable object, we can easily add or remove elements from the Array. And it dynamically changes as we modify the elements from the array.
Syntax
Array.push(item1, item2 …)
Example:
<!DOCTYPE html>
<html>
<body>
<h2>The Array.push()</h2>
<p>push() adds new items to the end of an array:</p>
<p id="demo"></p>
<script>
const fruits = ["Banana", "Orange", "Apple", "Mango"];
fruits.push("Grapes", "Lemon");
document.getElementById("demo").innerHTML = fruits;
</script>
</body>
</html>
Output:

2. Array.unshift() :
Adding elements at the front of an Array
Syntax
Array.unshift(item1, item2 …)
Example:
<!DOCTYPE html>
<html>
<body>
<h2>The Array.unshift() Method</h2>
<p>unshift() adds new items to the beginning of an array:</p>
<p id="demo"></p>
<script>
const fruits = ["Banana", "Orange", "Apple", "Mango"];
fruits.unshift("Grapes", "Lemon");
document.getElementById("demo").innerHTML = fruits;
</script>
</body>
</html>
Output:
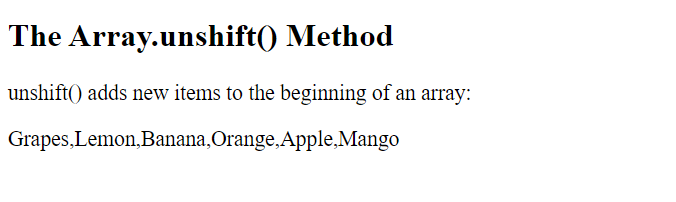
3. Array.pop() :
Removing elements from the end of an array
Syntax
Array.pop()
Example:
<!DOCTYPE html>
<html>
<body>
<h2>The Array.pop() Method</h2>
<p>pop() removes the last element of an array.</p>
<p id="demo"></p>
<script>
const fruits = ["Banana", "Orange", "Apple", "Mango"];
fruits.pop();
document.getElementById("demo").innerHTML = fruits;
</script>
</body>
</html>
Output:
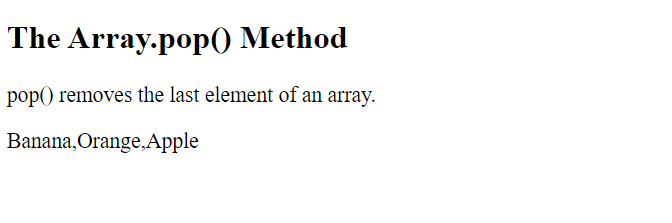
4. Array.shift() :
Removing elements at the beginning of an array
Syntax
Array.shift() Parameter : it takes no parameter
Example:
<!DOCTYPE html>
<html>
<body>
<h2>The Array.shift() Method</h2>
<p>shift() removes the first item of an array:</p>
<p id="demo"></p>
<script>
const fruits = ["Banana", "Orange", "Apple", "Mango"];
fruits.shift();
document.getElementById("demo").innerHTML = fruits;
</script>
</body>
</html>
Output:
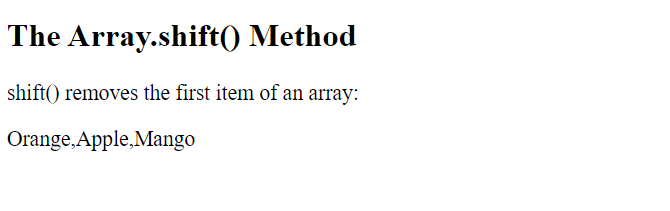
5. Array.splice() :
Insertion and Removal in between an Array
Syntax:
Array.splice (start, deleteCount, item 1, item 2….)
Example:
<!DOCTYPE html>
<html>
<body>
<h2>The Array.splice() Function</h2>
<p>The Array.splice() method adds array elements:</p>
<p id="demo"></p>
<script>
const fruits = ["Banana", "Orange", "Apple", "Mango"];
// At position 3, add 2 elements:
fruits.splice(3, 0, "Grapes", "Lemon");
document.getElementById("demo").innerHTML = fruits;
</script>
</body>
</html>
Output:
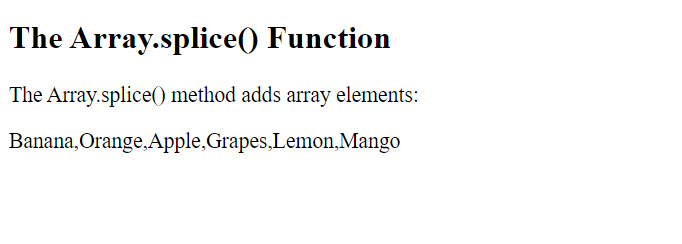
6. Array.map()
Array.map() creates a new array from calling a function for every array element. It calls a function once for each element in an array. It does not execute the function for empty elements and also does not change the original array.
Syntax:
array.map(function(currentValue, index, arr), thisValue)
Example:
<!DOCTYPE html>
<html>
<body>
<h2>The Array.map() Method</h2>
<p>The Array.map() method creates a new array from the results of calling a function for every element.</p>
<p id="demo"></p>
<script>
const numbers = [4, 9, 16, 25];
document.getElementById("demo").innerHTML = numbers.map(Math.sqrt);
</script>
</body>
</html>
Output:

7. Array.filter()
The Array.filter() method creates a new array filled with elements that pass a test provided by a function. The filter() method does not execute the function for empty elements and also does not change the original array.
Syntax:
array.filter(function(currentValue, index, arr), thisValue)
Example:
<!DOCTYPE html>
<html>
<body>
<h2>The Array.filter() Method</h2>
<p>Get every element in the array that has a value of 18 or more:</p>
<p id="demo"></p>
<script>
const ages = [32, 24, 19, 40];
document.getElementById("demo").innerHTML = ages.filter(checkAdult);
function checkAdult(age) {
return age >= 25;
}
</script>
</body>
</html>
Output:
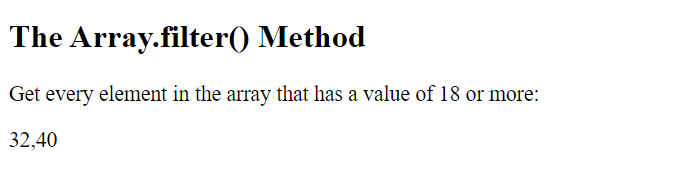
8. Array.some()
The some() method checks if any array elements pass a test (provided as a callback function). This method executes the callback function once for each array element. This method returns true (and stops) if the function returns true for one of the array elements. It also returns false if the function returns false for all of the array elements. It does not execute the function for empty array elements and also does not change the original array.
Syntax:
array.some(function(value, index, arr), this)
Example:
<!DOCTYPE html>
<html>
<body>
<h2>The Array.some() method</h2>
<p>The Array.some() method checks if any of the elements in an array pass a test (provided as a function).</p>
<p id="demo"></p>
<script>
const ages = [3, 10, 18, 20];
document.getElementById("demo").innerHTML = ages.some(checkAdult);
function checkAdult(age) {
return age > 18;
}
</script>
</body>
</html>
Output:
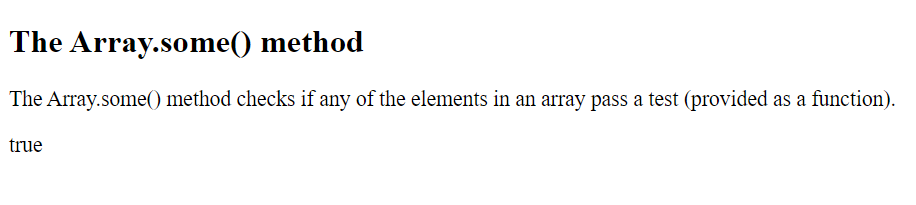
9. Array.sort()
The sort() sorts the elements of an array. This method overwrites the original array. The sort() sorts the elements as strings in alphabetical and ascending order.
Syntax:
array.sort(compareFunction)
Example:
<!DOCTYPE html>
<html>
<body>
<h2>The Array.sort() Method</h2>
<p>The Array.sort() method sorts the elements of an array.</p>
<p id="demo"></p>
<script>
const fruits = ["Banana", "Orange", "Apple", "Mango"];
document.getElementById("demo").innerHTML = fruits.sort();
</script>
</body>
</html>
Output:
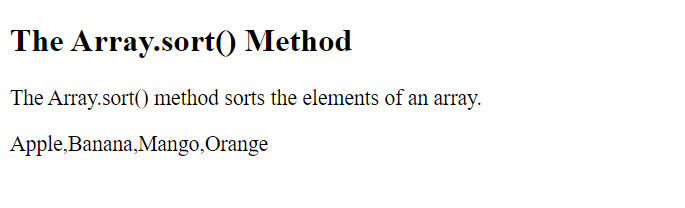
10. Array.reverse()
The reverse() method reverses the order of the elements in an array. This method overwrites the original array.
Syntax:
array.reverse()
Example:
<!DOCTYPE html>
<html>
<body>
<h2>The Array.reverse() Method</h2>
<p>The Array.reverse() method reverses the order of the elements in an array.</p>
<p id="demo"></p>
<script>
const fruits = ["Banana", "Orange", "Apple", "Mango"];
document.getElementById("demo").innerHTML = fruits.reverse();
</script>
</body>
</html>
Output:
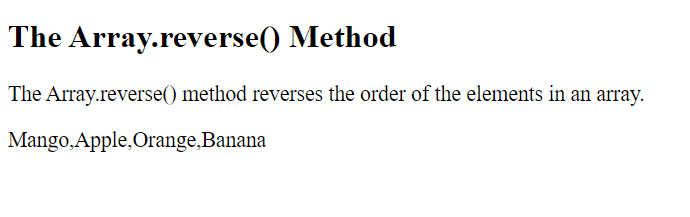
The Tech Platform
According to Google, Dinosaur Game is played millions of times every month worldwide, proving the enduring appeal of this simple yet entertaining game.