Java Design Pattern Interview Questions
- The Tech Platform
- Feb 2, 2023
- 7 min read
Updated: Mar 27, 2023
Java design patterns are reusable solutions to commonly occurring software design problems. They provide a structured approach to solving a particular design problem and can be adapted to specific situations. Understanding and using design patterns can improve the efficiency and maintainability of a software project.
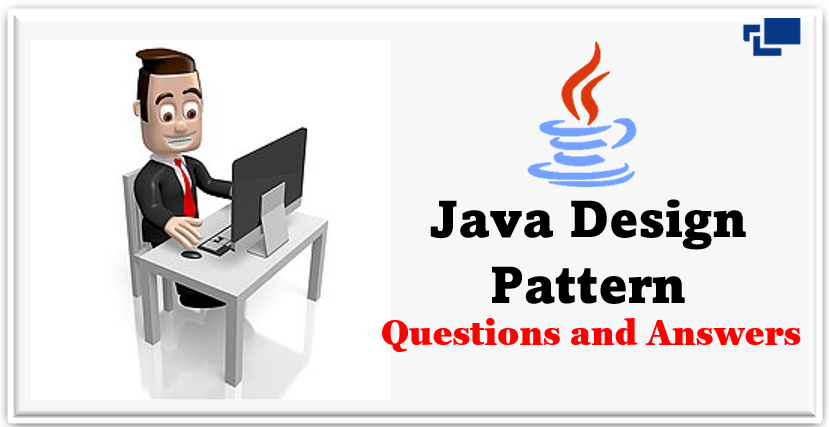
Below are some of the questions which can be asked. Let's begin...
Java Design Pattern Interview Questions
Question 1: What is a design pattern and why is it important in software development?
Answer: A design pattern is a general repeatable solution to a commonly occurring problem in software design. They are important because they provide a common vocabulary and understanding for software developers, making communication and collaboration easier. Design patterns also help to ensure that the software is designed in a way that is maintainable, scalable, and flexible.
Question 2: How many design patterns are there in Java?
Answer: There are 23 Gang of Four (GoF) design patterns and many more additional design patterns in Java.
Question 3: What are the types of design patterns in Java?
Answer: There are three types of design patterns in Java:
Creational,
Structural, and
Behavioral.
Question 4: Explain the difference between creational, structural, and behavioral design patterns.
Answer: Creational design patterns deal with object creation mechanisms, trying to create objects in a manner suitable to the situation. Structural design patterns deal with object composition, creating relationships between objects to form larger structures. Behavioral design patterns focus on communication between objects, what goes on between objects, and how they operate together.
Question 5: What is the strategy to describe a design pattern?
Answer: The strategy to describe a design pattern involves the following steps:
Identify the problem: Start by identifying the problem that the design pattern solves. Define the problem in a clear and concise manner.
Define the components: Outline the key components that make up the design pattern, including the context, the strategy interface, concrete strategies, and the client.
Explain the pattern's purpose: Explain why the design pattern is useful and how it solves the problem identified in step 1. Discuss the benefits of using the pattern and how it can improve the design of a software project.
Provide an example: Give a practical example of the design pattern in use. This could be a code example or a real-world scenario.
Compare to other patterns: Compare the design pattern to other related patterns and discuss the differences and similarities between them.
Summarize: Summarize the key points about the design pattern, including its purpose, components, benefits, and how it can be used.
Question 6: Explain the Adapter Design Pattern and its use case.
Answer: The Adapter Design Pattern is a structural design pattern that allows classes with incompatible interfaces to work together by wrapping its own interface around that of an already existing class. The Adapter Design Pattern is useful when you have an existing class that does not fit into your current system, but you want to reuse it.
Question 7: Explain the Template Method Design Pattern and its purpose.
Answer: The Template Method Design Pattern is a behavioral design pattern that defines the steps of an algorithm and allows subclasses to provide concrete implementation for one or more steps. This pattern is used to define the basic steps of an algorithm while allowing subclasses to provide the implementation for some or all of the steps.
Question 8: What is the Bridge Design Pattern and when do you use it?
Answer: The Bridge Design Pattern is a structural design pattern that decouples an abstraction from its implementation so that the two can vary independently. This pattern is useful when you have an abstraction that can have different implementations and you want to be able to switch between the implementations at runtime.
Question 9: Explain the Facade Design Pattern and its use case.
Answer: The Facade Design Pattern is a structural design pattern that provides a simplified interface to a complex system of classes, making it easier to use. The Facade Design Pattern is useful when you have a complex system that you want to simplify for clients so that they don't have to worry about the details of the underlying system.
Question 10: What is the Flyweight Design Pattern and when do you use it?
Answer: The Flyweight Design Pattern is a structural design pattern that is used to minimize memory usage by sharing as much data as possible with other similar objects. The Flyweight Design Pattern is useful when you have a large number of objects with similar data, and you want to minimize memory usage by sharing the data between the objects.
Question 11: Explain the Prototype Design Pattern and its use case.
Answer: The Prototype Design Pattern is a creational design pattern that allows you to create new objects by cloning existing objects, instead of creating new objects from scratch. The Prototype Design Pattern is useful when you want to create a large number of similar objects and you want to minimize the cost of creating the objects.
Question 12: What is the State Design Pattern and when do you use it?
Answer: The State Design Pattern is a behavioral design pattern that allows an object to change its behavior when its internal state changes. This pattern is useful when you have an object that can have multiple states and you want to change its behavior based on the state it is in.
Question 13: Explain the Chain of Responsibility Design Pattern and its use case.
Answer: The Chain of Responsibility Design Pattern is a behavioral design pattern that allows a series of objects to handle a request, with each object having the opportunity to handle the request or pass it on to the next object in the chain. The Chain of Responsibility Design Pattern is useful when you have a series of objects that can handle a request and you want to decouple the sender of the request from the receiver.
Question 14: What is the Mediator Design Pattern and when do you use it?
Answer: The Mediator Design Pattern is a behavioral design pattern that allows multiple objects to communicate with each other through a mediator object, instead of directly with each other. The Mediator Design Pattern is useful when you have a complex system with many objects that need to communicate with each other, and you want to simplify the communication between the objects.
Question 15: Explain the Memento Design Pattern and its purpose.
Answer: The Memento Design Pattern is a behavioral design pattern that allows an object to be saved and restored to its previous
Question 16: Explain the Singleton Design Pattern and why it's considered an anti-pattern in some cases.
Answer: The Singleton Design Pattern is a creational design pattern that ensures a class has only one instance and provides a global point of access to it. While this pattern can be useful in certain situations, it can also lead to tight coupling, making it difficult to test the code, and violating the principles of object-oriented programming. For these reasons, it's considered an anti-pattern in some cases.
Question 17: Describe the Command Design Pattern and how it's used in event-driven programming.
Answer: The Command Design Pattern is a behavioral design pattern that encapsulates a request as an object, allowing for deferred execution of operations and the ability to queue or log requests. It's often used in event-driven programming, where a user's action generates an event that is handled by a command object. The command object then carries out the requested action, decoupling the request from the object that handles it.
Question 18: Explain the Factory Method Design Pattern and how it's different from the Abstract Factory Design Pattern.
Answer: The Factory Method Design Pattern is a creational design pattern that provides an interface for creating objects in a superclass, but allows subclasses to alter the type of objects that will be created. It's different from the Abstract Factory Design Pattern, which provides an interface for creating families of related or dependent objects without specifying their concrete classes.
Question 19: What is the Observer Design Pattern and how does it enable communication between objects?
Answer: The Observer Design Pattern is a behavioral design pattern that enables communication between objects by allowing objects to observe changes in other objects and respond accordingly. In this pattern, objects can register to be notified when changes occur in other objects, and the notified objects can then take the appropriate action. This allows for loosely coupled communication between objects, making it easier to add or remove objects without affecting the rest of the system.
Question 20: Describe the Decorator Design Pattern and how it can be used to dynamically add new behavior to an object.
Answer: The Decorator Design Pattern is a structural design pattern that allows behavior to be added to an individual object, either statically or dynamically, without affecting the behavior of other objects from the same class. This pattern is achieved by wrapping an object in a decorator object that adds new behavior so that the decorated object inherits both the behavior of the original class and the behavior of the decorator. This allows for the new behavior to be added dynamically to an object, making it possible to add or remove behavior without affecting the rest of the system.
Question 21: Explain the Proxy Design Pattern and its use case.
Answer: The Proxy Design Pattern is a structural design pattern that provides a surrogate or placeholder object that controls access to another object, which may be remote, expensive to create, or in need of protection. The proxy object acts as an intermediary, forwarding requests to the real object, and providing additional functionality, such as caching, access control, or the ability to queue requests. This pattern is useful when you need to control access to an object, or when you want to provide additional functionality without affecting the real object.
Question 22: What are the different types of Proxy Design Patterns?
Answer: There are several types of Proxy Design Patterns, including:
Remote Proxy: It represents an object located remotely and acts as its representative or proxy in a local context.
Virtual Proxy: It is used to load the objects on demand or when required, and the loading of objects is done in the background.
Protection Proxy: It is used to control access to an object, and deciding who is authorized to access it.
Smart Reference: It is used to provide additional operations on a referenced object other than the operations defined in its interface.
Caching Proxy: It is used to cache the results of an expensive computation and returns the cached result for subsequent invocations.
Synchronization Proxy: It is used to synchronize access to a shared resource in a multithreaded environment.
Firewall Proxy: It is used to provide controlled access to a network resource, acting as a firewall.
The Tech Platform
Comments