Polymorphism is the ability of objects of different types to provide a unique interface for different implementations of methods. It is usually used in the context of late binding, where the behavior of an object to respond to a call to its method members is determined based on object type at run time. Polymorphism enables redefining methods in derived classes.
Polymorphism forms one of the fundamental concepts of object-oriented programming, along with encapsulation and inheritance.
ADVANTAGES OF POLYMORPHISM
It helps programmers reuse the code and classes once written, tested and implemented. They can be reused in many ways.
Single variable name can be used to store variables of multiple data types(Float, double, Long, Int etc).
Polymorphism helps in reducing the coupling between different functionalities.
DISADVANTAGES OF POLYMORPHISM
One of the disadvantages of polymorphism is that developers find it difficult to implement polymorphism in codes.
Run time polymorphism can lead to the performance issue as machine needs to decide which method or variable to invoke so it basically degrades the performances as decisions are taken at run time.
Polymorphism reduces the readability of the program. One needs to identify the runtime behavior of the program to identify actual execution time.
Classification of Polymorphism
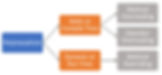
There are two types of polymorphism:
Static Polymorphism / Compile Time Polymorphism
Dynamic Polymorphism / Run Time Polymorphism
Compile Time Polymorphism
Whenever an object is bound with their functionality at the compile-time, this is known as the compile-time polymorphism. At compile-time, java knows which method to call by checking the method signatures. So this is called compile-time polymorphism or static or early binding. Compile-time polymorphism is achieved through method overloading. Method Overloading says you can have more than one function with the same name in one class having a different prototype. Function overloading is one of the ways to achieve polymorphism but it depends on technology that which type of polymorphism we adopt. In java, we achieve function overloading at compile-Time.
Example:
using System;
namespace Tutlane
{
public class Calculate
{
public void AddNumbers(int a, int b)
{
Console.WriteLine("This is the Example of Compile Time Polymorphism","\n");
Console.WriteLine("a + b = {0}", a + b);
}
public void AddNumbers(int a, int b, int c)
{
Console.WriteLine("a + b + c = {0}", a + b + c);
}
}
class Program
{
static void Main(string[] args)
{
Calculate c = new Calculate();
c.AddNumbers(4, 8);
c.AddNumbers(6, 3, 1);
Console.WriteLine("\nPress Enter Key to Exit..");
Console.ReadLine();
}
}
}
Output:

Types:
Compile Time Polymorphism can be classified as
Function Overloading or Method Overloading
Operator Overloading
Method Overloading
Method Overloading allows a class to have multiple methods with the same name but with a different signature. The functions or methods can be overloaded based on the number, type (int, float, etc), order, and kind (Value, Ref or Out) of parameters. The signature of a method consists of the name of the method and the data type, number, order, and kind (Value, Ref or Out) of parameters.
We can compare the function overloading with a person overloading. For example, if a person has already some work to do and if we are assigning some additional work to that person then the person’s work will be overloaded. In the same way, a function will have already some work to do and if we are assigning some additional work to that function, then we can say that the function is overloaded.
Operator Overloading
Overloading can be defined as a process of defining and implementing the polymorphism technique, which allows the variables or objects in the program to take on various other forms during the code execution. This technique can be used when the method properties are not similar to the type of arguments, different order of execution, when there is more than one method with same name and different properties, etc. This can be achieved in a program in different methods, such as The different number of parameters, different types of parameters, different order of parameters, optional parameters and named arguments.
Run Time Polymorphism
Whenever an object is bound with the functionality at run time, this is known as runtime polymorphism. The runtime polymorphism can be achieved by method overriding. Java virtual machine determines the proper method to call at the runtime, not at the compile time. It is also called dynamic or late binding. Method overriding says child class has the same method as declared in the parent class. It means if child class provides the specific implementation of the method that has been provided by one of its parent class then it is known as method overriding.
Example:
using System;
namespace Tutlane
{
// Base Class
public class BClass
{
public virtual void GetInfo()
{
Console.WriteLine("This is the Example of Run Time Polymorphism","\n");
}
}
// Derived Class
public class DClass : BClass
{
public override void GetInfo()
{
Console.WriteLine("Welcome to The Tech Platform");
}
}
class Program
{
static void Main(string[] args)
{
DClass d = new DClass();
d.GetInfo();
BClass b = new BClass();
b.GetInfo();
Console.WriteLine("\nPress Enter Key to Exit..");
Console.ReadLine();
}
}
}
Output:

Types:
Run Time Polymorphism can be classified as
Function Overriding or Method Overriding
Method Overriding
Method Overriding is a technique that allows the invoking of functions from another class (base class) in the derived class. Creating a method in the derived class with the same signature as a method in the base class is called as method overriding.
Overriding is a feature that allows a subclass or child class to provide a specific implementation of a method that is already provided by one of its super-classes or parent classes. When a method in a subclass has the same name, same parameters or signature and same return type(or sub-type) as a method in its super-class, then the method in the subclass is said to override the method in the super-class.
Important:
Method overriding is possible only in derived classes. Because a method is overridden in the derived class from the base class.
A non-virtual or a static method can’t be overridden.
Both the override method and the virtual method must have the same access level modifier.
Difference Between Compile Time Polymorphism and Run Time Polymorphism
Compile Time Polymorphism | Run Time Polymorphism |
In this, the call is resolved by the compiler. | In this, the call is not resolved by the compiler. |
Method overloading is the compile-time polymorphism where more than one methods share the same name with different parameters or signature and different return type. | Method overriding is the runtime polymorphism having same method with same parameters or signature, but associated in different classes. |
It is achieved by function overloading and operator overloading. | It is achieved by virtual functions and pointers. |
It provides fast execution because the method that needs to be executed is known early at the compile time. | It provides slow execution as compare to early binding because the method that needs to be executed is known at the runtime. |
Compile time polymorphism is less flexible as all things execute at compile time. | Run time polymorphism is more flexible as all things execute at run time. |
Difference Between Method Overloading and Method Overriding
Method Overloading | Method Overriding |
It is used to increase the readability of the program | Provides a specific implementation of the method already in the parent class |
It is performed within the same class | It involves multiple classes |
Parameters must be different in case of overloading | Parameters must be same in case of overriding |
Return type can be different but you must change the parameters as well. | Return type must be same in overriding |
Static methods can be overloaded | Overriding does not involve static methods. |
The Tech Platform