How to Migrate ASP.NET HTTP Handlers and Modules to ASP.NET Core Middleware
- The Tech Platform
- Dec 24, 2021
- 8 min read
Updated: Feb 2, 2024
ASP.NET, a stalwart in web application development, has undergone significant transformations over the years. Among these changes, the evolution from traditional ASP.NET HTTP Handlers and Modules to the more robust ASP.NET Core Middleware stands out. This article delves into this transition, exploring the journey from the old guard to the modern Middleware architecture, and elucidating the importance of making this migration for improved performance and scalability.
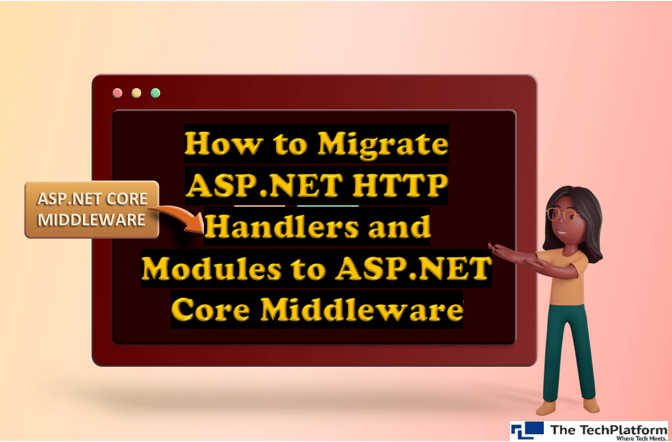
Let’s get started!
Understanding ASP.NET HTTP Handlers
HTTP Handlers in ASP.NET serve as integral components for processing incoming HTTP requests and generating appropriate responses. These handlers intercept requests before reaching the page or controller, allowing developers to customize request processing based on specific criteria. A fundamental aspect of handlers is their ability to execute custom logic for different types of requests.
Example:
Consider a scenario where a custom HTTP handler is created to handle requests for pages with a ".aspx" extension and redirect them to a new page. The handler class, RedirectionHandler, implements the IHttpHandler interface, defining the IsReusable property and the ProcessRequest method.
Let's understand this in detail.
Custom HTTP Handler Class (RedirectionHandler):
This class implements the IHttpHandler interface, which requires the implementation of two members: IsReusable and ProcessRequest.
IsReusable indicates whether the handler can be reused for multiple requests. In this case, it is set to false, meaning a new instance of the handler will be created for each request.
ProcessRequest is the method where the logic for handling the HTTP request is implemented. In this example, it writes a simple HTML message to the response output, indicating that the handler is processing files with a ".aspx" extension.
public class RedirectionHandler : IHttpHandler
{
public bool IsReusable
{
get { return false; }
}
public void ProcessRequest(HttpContext context)
{
var response = context.Response;
response.Write("<p>Process files with .aspx extension</p>");
// Any redirection logic can be written here.
}
}
Configuration in web.config:
The web.config file includes a configuration for the custom HTTP handler inside the <system.webServer> section under the <handlers> element.
The <add> element specifies details about the handler, such as its name, the HTTP verbs it responds to (in this case, "*" meaning all verbs), the path pattern it matches (.aspx extension), the type of the handler (MyWebApplication.RedirectionHandler), and the resource type (Unspecified).
<!-- Code inside configuration -> system.webServer -> handlers section --> <add name="RedirectionHandler" verb="*" path="*.aspx" type="MyWebApplication.RedirectionHandler" resourceType="Unspecified"/>
With this setup, any request for a page with a ".aspx" extension will be processed by the custom HTTP handler (RedirectionHandler), and you have the flexibility to implement redirection logic or any other custom processing within the ProcessRequest method.
Examples of common scenarios using HTTP Handlers:
Image Processing: Use an HTTP Handler to dynamically resize or manipulate images based on requested parameters.
File Downloads: Implement a custom handler for serving files with specific permissions or logging download activities.
RESTful Endpoints: Employ HTTP Handlers to create RESTful APIs by processing requests and generating appropriate JSON or XML responses.
Custom File Types: Handle requests for non-standard file types or custom resources based on specific criteria.
Understanding ASP.NET HTTP Modules
HTTP Modules in ASP.NET provide a flexible and extensible mechanism to inject custom logic into the processing pipeline of each incoming request and outgoing response. These modules enable developers to inspect, modify, or augment both the request and response at various stages, before and after the execution of the HTTP handler. HTTP Modules are beneficial for tasks such as authentication, logging, caching, security checks, and other global-level operations that need to be performed consistently across an application.
Module Class for Restricting Users Based on IP Address:
Consider a scenario where an HTTP Module is implemented to restrict access to users based on their IP addresses. The IPRestrictionModule class, implementing IHttpModule, is registered in the application to execute logic before the request begins (BeginRequest) and after the request ends (EndRequest).
public class IPRestrictionModule : IHttpModule
{
public void Init(HttpApplication context)
{
context.BeginRequest += (source, arguments) =>
{
var application = (HttpApplication)source;
var beginContext = application.Context;
beginContext.Response.Write("<p>Restrict Users based on IP</p>"); // Code logic for IP restriction comes here.
};
context.EndRequest += (source, arguments) =>
{
var application = (HttpApplication)source;
var endContext = application.Context;
endContext.Response.Write("<p>Request ended.</p>");
};
}
}
Code in web.config to Register the Module:
The web.config file includes a configuration entry inside the <system.webServer> section under the <modules> element.
<!-- Code inside configuration -> system.webServer -> modules section --> <add name="RestrictionModule" type="MyWebApplication.IPRestrictionModule" />
In this example, the IPRestrictionModule is registered as "RestrictionModule," and it will execute its logic before and after the HTTP handler processes the request. This showcases how HTTP Modules offer a powerful way to customize the request/response pipeline in ASP.NET applications.
Examples of how HTTP Modules can be utilized in traditional ASP.NET applications:
Authentication and Authorization: An HTTP Module can be employed to perform custom authentication and authorization checks for each incoming request. This allows developers to enforce security policies, validate user credentials, and control access to different parts of the application.
Logging and Error Handling: Create an HTTP Module to log information about each request, including details like request parameters, execution time, and any encountered errors. This is valuable for tracking application behavior, troubleshooting issues, and analyzing performance.
Caching: Implement an HTTP Module to handle caching at the global level. This module can inspect requests and determine whether the response can be served from the cache, optimizing performance by reducing the load on the server.
Compression: Develop an HTTP Module to compress outgoing responses before they are sent to the client. This helps in minimizing bandwidth usage and improving the overall speed and responsiveness of the application, especially for clients with slower network connections.
URL Rewriting: Use an HTTP Module for URL rewriting to modify incoming URLs based on predefined rules. This is beneficial for creating clean and user-friendly URLs that enhance search engine optimization (SEO) and user experience.
Custom Headers: Implement an HTTP Module to add, modify, or remove custom headers from both incoming requests and outgoing responses. This can be useful for setting security-related headers, handling CORS (Cross-Origin Resource Sharing), or other specific requirements.
Request Filtering: Develop an HTTP Module to filter incoming requests based on specific criteria such as IP addresses, user agents, or request methods. This allows for custom request filtering logic before it reaches the application's core functionality.
Localization: Create an HTTP Module for handling localization by inspecting incoming requests and modifying the application's culture or language settings accordingly. This ensures that the application serves content in the preferred language based on user preferences or browser settings.
Introducing ASP.NET Core Middleware
Middleware in ASP.NET Core refers to a pipeline of components that are executed in sequence to handle requests and responses. Each middleware component in the pipeline plays a specific role in processing HTTP requests and generating responses. The purpose of middleware is to provide a modular and flexible approach to handle cross-cutting concerns, such as authentication, logging, and caching, in a centralized manner.
Key Differences Middleware and HTTP Handlers/Modules:
Execution Model: Unified pipeline vs. separate handling in traditional ASP.NET.
Flexibility: Middleware is more flexible and reusable.
Request Delegate Pattern: Middleware uses a request delegate pattern for dynamic control.
Advantages of using Middleware in ASP.NET Core
Unified Pipeline: Simplifies application architecture.
Granular Control: Customize request/response flow dynamically.
Dependency Injection: Supports dependency injection for better testability.
Reusability and Composition: Promotes code modularity and reusability.
Asynchronous Support: Fully supports asynchronous programming for scalability.
In ASP.NET Core, the migration from HTTP Handlers to Middleware involves utilizing the IApplicationBuilder interface in the Configure method of the Startup class. Four key methods of the Application Builder file are used to construct the middleware pipeline: Use, Map, Run, and MapWhen.
These methods enable the addition of middleware components to handle various aspects of the request/response lifecycle.
Let’s see how to migrate ASP.NET HTTP handlers to ASP.NET Core middleware!
Step-by-step Migration Process:
Updating HTTP Handlers to Middleware
In traditional ASP.NET, HTTP Handlers are components designed to process incoming requests. The example below showcases a RedirectionHandler HTTP Handler.
public class RedirectionHandler : IHttpHandler
{
public void ProcessRequest(HttpContext context)
{
context.Response.Write("<p>Process files with .aspx extension</p>");
// Redirection logic here.
}
public bool IsReusable => false;
}
The ProcessRequest method is responsible for handling the incoming request and generating the appropriate response. In this case, it writes a message to the response output and includes logic for redirection.
Handling Requests and Responses in Migrated Middleware:
In the ASP.NET Core Middleware, handling requests and responses is achieved through the InvokeAsync method. Here's an example of migrating the logic to a RedirectionHandlerMiddleware:
public class RedirectionHandlerMiddleware : IMiddleware
{
public async Task InvokeAsync(HttpContext context, RequestDelegate next)
{
await context.Response.WriteAsync("<p>Process files with .aspx extension</p>");
// Redirection logic here.
}
}
In the Middleware, the InvokeAsync method takes the HttpContext and a RequestDelegate parameter. It asynchronously handles the incoming request and generates the appropriate response. The logic is adapted to the ASP.NET Core Middleware conventions.
Adapting Existing Logic to Middleware Conventions:
Original HTTP Handler Logic: The logic in the original HTTP Handler is typically implemented within the ProcessRequest method.
Migrated Middleware Logic: In the Middleware, the logic is moved to the InvokeAsync method, adhering to ASP.NET Core conventions. This method allows for more flexibility and aligns with the asynchronous nature of ASP.NET Core.
Migrating Specific HTTP Handler to Middleware (Example):
STEP 1: Add a Class Named RedirectionHandlerMiddleware:
Create a new class named RedirectionHandlerMiddleware implementing IMiddleware.
public class RedirectionHandlerMiddleware : IMiddleware
{
public async Task InvokeAsync(HttpContext context, RequestDelegate next)
{
await context.Response.WriteAsync("<p>Process files with .aspx extension</p>");
// Redirection logic here.
}
}
STEP 2: Create an Extension Method on the ApplicationBuilder File:
Add an extension method to the ApplicationBuilder for using the RedirectionHandlerMiddleware in the request pipeline.
public static class MiddlewareExtension
{
public static IApplicationBuilder UseRedirectionHandlerMiddleware(this IApplicationBuilder app)
{
return app.UseMiddleware<RedirectionHandlerMiddleware>();
}
}
STEP 3: Create a Class Named MiddlewareExtension for the Created Extension:
Create a class named MiddlewareExtension containing the extension method.
public static class MiddlewareExtension
{
public static IApplicationBuilder UseRedirectionHandlerMiddleware(this IApplicationBuilder app)
{
return app.UseMiddleware<RedirectionHandlerMiddleware>();
}
}
STEP 4: Include the Code in the Startup.cs File for Using the RedirectionHandlerMiddleware:
In the Startup.cs file, use the extension method within a specific condition.
app.MapWhen(context => context.Request.Path.ToString().EndsWith(".aspx"), appBuilder =>
{
appBuilder.UseRedirectionHandlerMiddleware();
});
Now, you have successfully migrated the ASP.NET HTTP Handler (RedirectionHandler) to ASP.NET Core Middleware (RedirectionHandlerMiddleware). Repeat these steps for other HTTP Handlers as needed. This process ensures a smooth transition to the modular and unified approach provided by ASP.NET Core Middleware.
Migrating HTTP modules to middleware
In the migration process from HTTP Modules to Middleware, the first step involves rewriting the functionality of the existing HTTP Module using the middleware paradigm. This ensures that the module's logic is adapted to the ASP.NET Core middleware conventions.
It's crucial to ensure that the migrated middleware seamlessly integrates with the ASP.NET Core pipeline. This involves creating extension methods, updating existing extension classes, and including the middleware in the request pipeline.
Migrating Specific HTTP Module to Middleware (Example):
STEP 1: Add a Class Named IPRestrictionModuleMiddleware:
Create a new class named IPRestrictionModuleMiddleware to encapsulate the functionality of the existing HTTP Module. Implement the IMiddleware interface.
public class IPRestrictionModuleMiddleware : IMiddleware
{
private readonly RequestDelegate next;
public IPRestrictionModuleMiddleware(RequestDelegate next)
{
next = next;
}
public async Task InvokeAsync(HttpContext context)
{
await context.Response.WriteAsync("<p>Begin request</p>");
await _next.Invoke(context);
await context.Response.WriteAsync("<p>End request</p>");
}
}
STEP 2: Add an Extension Method to Add the Middleware in the Request Pipeline:
Add an extension method to the MiddlewareExtensions class for using the IPRestrictionModuleMiddleware in the request pipeline.
public static class MiddlewareExtensions
{
// Existing extension method for RedirectionHandlerMiddleware
public static IApplicationBuilder UseRedirectionHanlderMiddleware(this IApplicationBuilder app)
{
return app.UseMiddleware<RedirectionHandlerMiddleware>();
}
// New extension method for IPRestrictionModuleMiddleware
public static IApplicationBuilder UseIPRestrictionModuleMiddleware(this IApplicationBuilder builder)
{
return builder.UseMiddleware<IPRestrictionModuleMiddleware>();
}
}
STEP 3: Add the Code in the Existing Extension Class MiddlewareExtension:
Include the newly created extension method for IPRestrictionModuleMiddleware in the existing MiddlewareExtensions class.
public static class MiddlewareExtensions
{
// Existing extension method for RedirectionHandlerMiddleware
public static IApplicationBuilder UseRedirectionHanlderMiddleware(this IApplicationBuilder app)
{
return app.UseMiddleware<RedirectionHandlerMiddleware>();
}
// New extension method for IPRestrictionModuleMiddleware
public static IApplicationBuilder UseIPRestrictionModuleMiddleware(this IApplicationBuilder builder)
{ return builder.UseMiddleware<IPRestrictionModuleMiddleware>();
}
}
STEP 4: Include the Middleware in the Startup.cs File:
In the Startup.cs file, include the middleware for handling IP restrictions.
// For Module
app.UseIPRestrictionModuleMiddleware();
// For Handler
app.MapWhen(context => context.Request.Path.ToString().EndsWith(".aspx"), appBuilder =>
{
appBuilder.UseRedirectionHanlderMiddleware();
});
Thus, by following these steps, we have successfully migrated ASP.NET HTTP modules to ASP.NET Core middleware. The migration involves rewriting module functionality, ensuring seamless integration, creating extension methods, and including the middleware in the ASP.NET Core pipeline.
Conclusion
In this blog post, we have seen how to easily migrate ASP.NET HTTP handlers and modules to ASP.NET Core middleware.
Comments