
GitHub uses GitHub Actions to launch CI / CD, the documentation for which can be found here and read in full. Here’s an example of how to set up a CI / CD using GitHub.
We built a sample project and did a sample test to see how it worked.
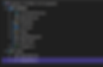
Because we want to see the process of CI CD, we write our test simply.
using Xunit;
namespace UiTest
{
public class SampleTest
{
[Fact]
public void Just_For_Run_Sample_Test()
{
// Arrange
var myvar = "test";
//Act
bool result = (myvar == "test");
//Assert
Assert.True(result);
}
}
}
We see that our testament, which has no business, is passed.

Inside the repository we created on the GitHub site, go to the Action tab and select .NET.
Note that we can use different languages and platforms to use this service, because our project example is .NET, we choose this option.

Click on the Configure button to get GitHub a sample code for the settings for us.

As you can see, in the .github workflows folder, it creates a file called dotnet.yml, and we just need to write the settings we want in this file.
The file is with yml extension and is for our settings.
For the sample project, we use the following code.
name: .NET
on:
push:
branches: [ master ]
pull_request:
branches: [ master ]
jobs:
build-test-deploy:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v3
- name: 🚀 Setup .NET
uses: actions/setup-dotnet@v2
with:
dotnet-version: 6.0.x
- name: 🔨 Restore dependencies
run: dotnet restore
- name: 💣 Build
run: dotnet build --no-restore
- name: 🔍 Test
run: dotnet test --no-build --verbosity normal
- name: 📂 Sync files
uses: SamKirkland/FTP-Deploy-Action@4.3.0
with:
server: ${{ secrets.ftp_server }}
username: ${{ secrets.ftp_user }}
password: ${{ secrets.ftp_password }}
At the beginning of the file, as specified in., We said in which actions the operation should be performed
on:
push:
branches: [ master ]
When we Push it on Master branch, the operation starts .
Next we created a job and called it build-test-deploy, which means what operations are performed in this job
jobs:
build-test-deploy:
And the most important part is our steps or our step, which is performed in turn and we have the following steps:
Setup .NET
I tell GitHub what our project code is and what version it is
uses: actions/setup-dotnet@v2
with:
dotnet-version: 6.0.x
Restore dependencies
Which captures the packages that are within the project
run: dotnet test — no-build — verbosity normal
Test
Runs our tests (the important part of the job is that the automated tests we wrote must be passed to continue the process)
run: dotnet test — no-build — verbosity normal
Sync files
uses: SamKirkland/FTP-Deploy-Action@4.3.0
with:
server: ${{ secrets.ftp_server }}
username: ${{ secrets.ftp_user }}
password: ${{ secrets.ftp_password }}
If all the steps are passed, our file will be transferred to the FTP address that we gave it.
We defined the FTP settings by secret so that we can use them but they are not visible.
By $ {secrets.ftp_password} We mean that the GitHub reads this value from the secrets we defined for it.
To define the secret we go to
Settings> Secrets> Actions
Go and click on New repository secret, then enter the name of your variable, for example ftp_password, and enter its value, and in our settings code this way we can return its value
${{ secrets.ftp_password }}

This will read our values, but they will not be accessible or visible.
If the gateway encounters a problem in any of the steps, the process will stop and will not continue.
At the end, our project will be published.
Well, we commit the file and it PUSH and start CI CD automatically.

The file is added to the .github / workflows / dotnet.yml address and we must remember the values
ftp_server
ftp_user
ftp_password
Be sure to create it in Settings> Secrets> Actions and define it.
Let’s go and see what happens. :)
Well, we did the first push and GitHub Actions starts
Go to the Action tab and see that we got an error

By clicking and viewing, we can see the details of the error

In the 📂 Sync files section for transferring files to FTP we got an error !
Error: Input needed and not supplied: server
We ate because we did not define the secret for the server and did not set the value.
As you can see, GitHub shows all the steps with details and logs that you can see by clicking on each step.
An email will also be sent to you informing that we made a mistake and faild job.

To fix the problem, we define the secrets and run them again.

Note that you can do both a push to re-run and the re-run button inside the actions.

We put a simple Push inside the project itself to see the result

Yes :) We see that the action was successfully executed and our version was published, that done with just a simple push.
From now on, it is enough to just push our changes to get our version on the server, just as easily.
But keep in mind that if any of the steps are not done, our version will not publish , for example, our tests will not pass, for example, I will pass the test result false (I will put a ! next to it) and I will push it to see the result.
Assert.True( ! result);
The result will be

My test did not pass and as a result, my version was not published.
Of course, I will fix the test again to pass.

I hope you like this article and it will be useful for you :).
Source: Medium - Reza Mansouri
The Tech Platform