Explore the PHP in_array Function
- The Tech Platform
- Oct 4, 2023
- 7 min read
PHP is a programming language that comes with a treasure trove of built-in tools to make developers' lives easier. Among these tools, there's one called PHP in_array function, which is like a magical detective that helps us find things in lists. Whether you're just starting with PHP or you've been coding for a while, understanding how PHP in_array works can be a game-changer.
Imagine you have a box full of different colored marbles, and you want to find out if there's a blue one in there. That's where PHP in_array comes in handy. It checks each marble to see if it's blue and tells you if it found one.
In this article, we're going to take a closer look at PHP in_array function. We'll learn what it is, how to use it, and see some real-life examples.
So, let's discover how to use the in_array function in PHP—it's like having a superpower for your code!
Table of Contents:
Syntax
How it works?
Benefits of using PHP in_array function
Case-Sensitive
Strict Type Comparison
What is the PHP in_array function?
The PHP in_array function is a built-in array function that is used to determine whether a specific value exists within an array or not. In essence, it checks if the array contains a particular item and returns a boolean (true or false) result.
Syntax
in_array($needle, $haystack, $strict = false);
$needle: This is the value you want to search for within the array.
$haystack: This is the array in which you want to perform the search.
$strict (optional): When set to true, it performs a strict type comparison (both value and data type must match); if set to false (default), it performs a loose comparison (data type is not considered).
How it Works:
The PHP in_array function takes the value you're searching for ($needle) and the array in which you're searching ($haystack) as parameters.
It then iterates through the elements in the array, comparing each element to the specified $needle.
If it finds a match, it returns true, indicating that the value is present in the array.
If no match is found after checking all elements, it returns false, indicating that the value is not in the array.
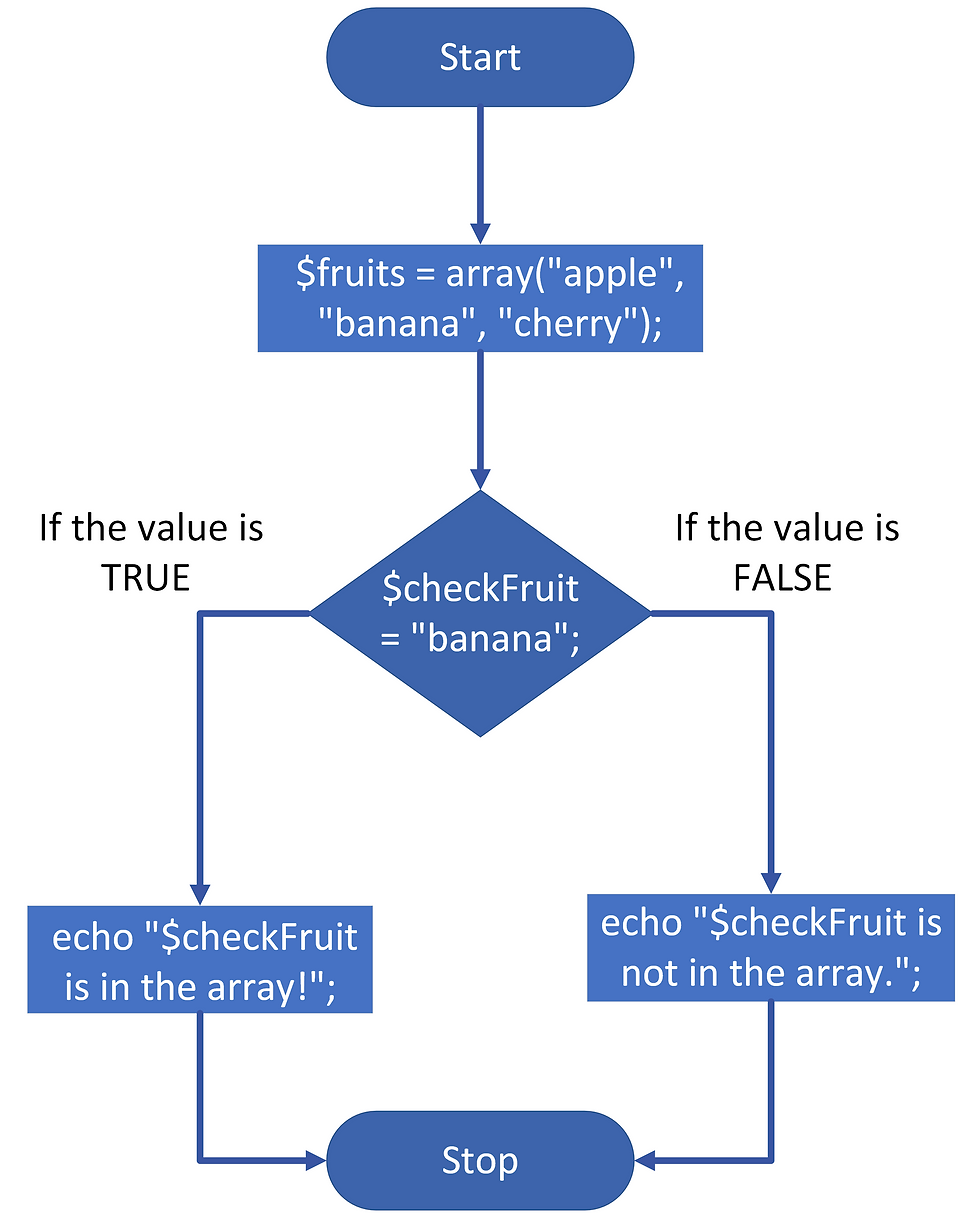
Example:
$fruits = array("apple", "banana", "cherry");
$checkFruit = "banana";
if (in_array($checkFruit, $fruits)) {
echo "$checkFruit is in the array!";
} else {
echo "$checkFruit is not in the array.";
}
In this example, PHP in_array checks if the value "banana" exists in the $fruits array. Since "banana" is present, it returns true, and the message "banana is in the array!" is displayed.
Benefits of using the in_array() function
Simplicity: It's easy to use, making it a great choice for beginners who want to check for the presence of specific values in an array.
Efficiency: in_array is efficient in terms of code writing and execution. It saves you from writing a custom loop to search for a value within an array.
Readability: The code using PHP in_array is quite readable and self-explanatory, which makes your code more maintainable.
Versatility: You can use PHP in_array with both indexed and associative arrays, allowing you to check for values in various data structures.
Boolean Result: It returns a simple true or false result, making it easy to use in conditional statements.
Examples
Here, you'll find two illustrative examples that will enhance your understanding of the PHP in_array function.
Example 1: Case-Sensitive
Case-sensitive comparisons are particularly important in scenarios where the capitalization of characters matters, as they ensure an exact match based on both the value and case of the elements in the array.
In this example, we have a case-sensitive demonstration to determine whether the color 'blue' exists within an array or not.
<!DOCTYPE html>
<html>
<head>
<title>Check Color in Array</title>
</head>
<body>
<h3>This is an case-sensitive example to check whether "blue" exists in an array or not.</h3>
<?php
$colors = array("Red", "Green", "Blue");
$checkColor = "blue";
echo "Colors in an array are:<ul>";
foreach ($colors as $color) {
echo "<li>$color</li>";
}
echo "</ul>";
echo "Color to check - $checkColor <br>";
if (in_array($checkColor, $colors, true)) {
echo "$checkColor is in the array (case-sensitive)!";
} else {
echo "$checkColor is not in the array (case-sensitive).";
}
?>
</body>
</html>
Output:
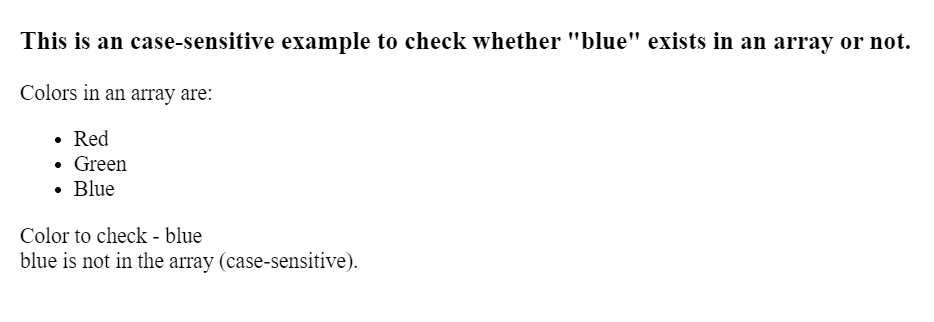
Example 2: Strict Type Comparison
Strict type comparison means that not only does the value being searched for need to match the value in the array, but the data types of both values also need to be the same for a match to occur.
<!DOCTYPE html>
<html>
<head>
<title>Check Number in Array</title>
</head>
<body>
<h3>Example of Strict Type Comparison</h3>
<?php
$values = array(1, 2, 3, "4", 5);
$checkValue = "4";
echo "The Array List is : <ul>";
foreach ($values as $value) {
echo "<li>$value</li>";
}
echo "</ul>";
echo "Number to Check - $checkValue <br>";
if (in_array($checkValue, $values, true)) {
echo "$checkValue is in the array (strict type comparison)!";
} else {
echo "$checkValue is not in the array (strict type comparison).";
}
?>
</body>
</html>
In the above code, we are using the PHP in_array function with the third argument set to true. This true argument tells PHP to perform a strict type comparison while searching for $checkValue in the $values array.
The if statement checks the result of in_array. If a match is found, it means that not only does the value "4" exist in the array, but it also has the same data type as the searched value (a string).
If a match is found, it echoes the message "$checkValue is in the array (strict type comparison)!" indicating that the value "4" is present in the array with the same data type.
If no match is found, it echoes the message "$checkValue is not in the array (strict type comparison).
Output:
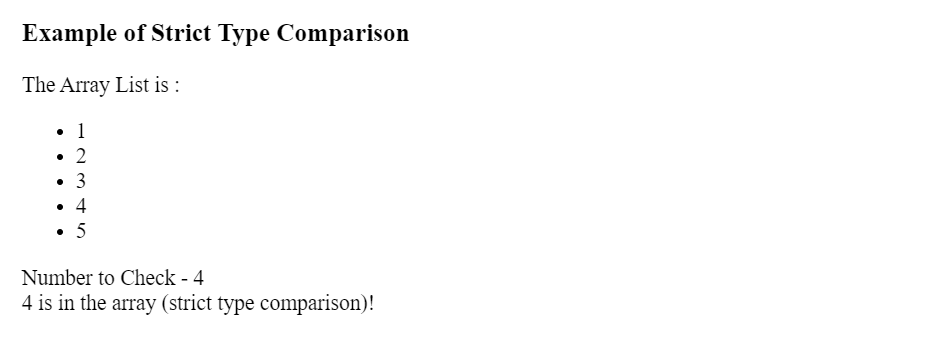
Next, let's examine the following code, where the arrangement of the number array list is different.
<!DOCTYPE html>
<html>
<head>
<title>Check Number in Array</title>
</head>
<body>
<h3>Example of Strict Type Comparison</h3>
<?php
$values = array(1, 2, 3, "4", 5);
$checkValue = "4";
echo "The Array List is : <ul>";
foreach ($values as $value) {
echo "<li>$value</li>";
}
echo "</ul>";
echo "Number to Check - $checkValue <br>";
if (in_array($checkValue, $values, true)) {
echo "$checkValue is in the array (strict type comparison)!";
} else {
echo "$checkValue is not in the array (strict type comparison).";
}
?>
</body>
</html>
Since you are using strict type comparison (true as the third argument of in_array), it will perform a strict comparison, considering both the value and data type. In this case, "1" (a string) is not strictly equal to the integer 1 in the array. Therefore, it will display:
1 is not in the array (strict type comparison)
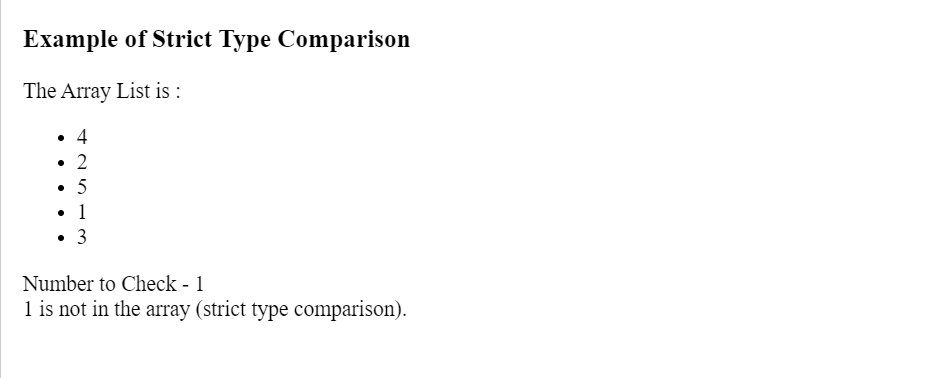
Difference between PHP in_array vs array_search function
The PHP in_array and array_search() functions are both useful for working with arrays. Below we have the difference between the two:
Factors | PHP in_array function | PHP array_search function |
Returns | The PHP in_array function only returns true or false depending on whether or not the value exists in the array. It cannot return the key of the value. | The array_search() function can return the key of the value if it is found in the array. This is useful if you need to perform some operation on the value, such as modifying it or deleting it. |
Type of comparison | Performs a loose type comparison by default. | Performs a loose type comparison by default, but can also perform a strict type comparison if the $strict parameter is set to true. |
Traverses the array | Traverses the entire array to find the value. | Traverses the array until it finds the value or reaches the end of the array. |
Performance | Generally slower than array_search(), especially for large arrays. | Generally faster than PHP in_array(), especially for large arrays. |
Implementation | PHP in_array function is implemented using linear search algorithm. | PHP array_search function is implemented using a binary search algorithm |
Multi-dimensional array | It cannot be used to search for value in a multi-dimensional array | It can be used to search for a value in a multi-dimensional array. |
When to use | It is more efficient to use when you need to know whether or not the value exists in an array. | It is more efficient to use when you need to get the key value you are searching for. |
Simplicity | Easy to use | Complex to use |
Which function you choose to use will depend on your specific needs. If you only need to know whether or not the value exists in the array, the PHP in_array() function is a good choice. If you need to get the key of the value you are searching for, or if you need to search for a value in a multi-dimensional array, the array_search() function is a better choice.
Applications
Below are some practical applications of the PHP in_array function that demonstrate its versatility and usefulness in various programming scenarios:
1. Validating user input
You can use the PHP in_array function to validate user input, such as checking if a user has selected a valid option from a drop-down menu.
For example, the following code shows how to check if a user has selected a valid color from a drop-down menu:
$colors = ['red', 'green', 'blue'];
$color = $_POST['color'];
if (!in_array($color, $colors))
{
echo 'Please select a valid color.';
}
If the user selects a valid color, the code will execute without any errors. However, if the user selects an invalid color, the code will display an error message.
2. Checking for duplicate values
You can use the PHP in_array function to check for duplicate values in an array. This can be useful for tasks such as cleaning up a database or ensuring that a user has not already entered a duplicate value into a form.
For example, the following code shows how to check for duplicate values in an array of email addresses:
$emails = ['john.doe@gmail.com', 'jane.doe@gmail.com', 'john.doe@gmail.com'];
$duplicateEmails = [];
foreach ($emails as $email)
{
if (in_array($email, $emails, true))
{
$duplicateEmails[] = $email;
}
}
if (count($duplicateEmails) > 0)
{
echo 'The following email addresses are duplicates: ' . implode(', ', $duplicateEmails);
}
If there are any duplicate email addresses in the array, the code will display a list of the duplicate email addresses.
3. Searching for specific values in an array
You can use the PHP in_array function to search for specific values in an array. This can be useful for tasks such as finding a particular product in a catalog or checking if a user has access to a specific resource.
For example, the following code shows how to search for the product ID 12345 in an array of products:
$products = [
[
'id' => 12345,
'name' => 'Product A',
],
[
'id' => 67890,
'name' => 'Product B',
],
];
$productId = 12345;
if (in_array($productId, array_column($products, 'id')))
{
echo 'The product ID ' . $productId . ' was found in the array.';
} else {
echo 'The product ID ' . $productId . ' was not found in the array.';
}
If the product ID is found in the array, the code will display a message saying that the product ID was found. Otherwise, the code will display a message saying that the product ID was not found.
4. Performing complex data analysis
The PHP in_array function can also be used to perform complex data analysis. For example, you could use the in_array() function to identify the most common values in an array or to find the values that are unique to each element in an array.
For example, the following code shows how to identify the most common values in an array of colors:
$colors = ['red', 'green', 'blue', 'red', 'green'];
$colorCounts = [];
foreach ($colors as $color)
{
if (!isset($colorCounts[$color])) {
$colorCounts[$color] = 0;
}
$colorCounts[$color]++;
}
$mostCommonColors = [];
foreach ($colorCounts as $color => $count)
{
if ($count > 1)
{
$mostCommonColors[] = $color;
}
}
if (count($mostCommonColors) > 0)
{
echo 'The most common colors in the array are: ' . implode(', ', $mostCommonColors);
}
If there are any colors that appear more than once in the array, the code will display a list of the most common colors.
Conclusion
The PHP in_array function is a handy tool that can simplify your coding tasks and make your PHP applications more powerful. Whether you're new to PHP or an experienced developer, mastering in_array allows you to efficiently search for values in arrays, perform strict type comparisons, and address various programming challenges.
Comments