
What are JavaScript Promises?
JavaScript Promises are used to handle asynchronous operations in JavaScript. They are easy to manage when dealing with multiple asynchronous operations where callbacks can create callback hell leading to unmanageable code.
A JavaScript Promise object can be:
Pending
Fulfilled
Rejected

The Promise object supports two properties: state and result. While a Promise object is "pending" (working), the result is undefined. When a Promise object is "fulfilled", the result is a value. When a Promise object is "rejected", the result is an error object.
Syntax:
let myPromise = new Promise(function(myResolve, myReject)
{
// "Producing Code" (May take some time)
myResolve(); // when successful
myReject(); // when error
});
// "Consuming Code" (Must wait for a fulfilled Promise)
myPromise.then(
function(value) { /* code if successful */ },
function(error) { /* code if some error */ }
);
Code:
<!DOCTYPE html>
<html>
<body>
<h2>JavaScript Promise</h2>
<p>Wait 3 seconds (3000 milliseconds) for this page to change.</p>
<h1 id="demo"></h1>
<script>
const myPromise = new Promise(function(myResolve, myReject) {
setTimeout(function(){ myResolve("The Tech Platform"); }, 3000);
});
myPromise.then(function(value) {
document.getElementById("demo").innerHTML = value;
});
</script>
</body>
</html>
Output:

What is JavaScript Observable?
Observable JavaScript represents a progressive way of handling events, async the activity, and multiple values in JavaScript. These observables are just the functions that throw values and Objects known as observers subscribe to such values that define the callback functions such as error(), next() and complete(). These objects are passed as arguments to observable functions and these observable functions call observer methods based on the HTTP requests, events, etc. This allows the observer to “listen” for state change emitted by observable function. Here, we shall see in depth and how it is implemented with few examples.
Syntax:
import { Observable } from 'rxjs';
Code:
import { Observable } from 'rxjs';
let observable = new Observable(observer => {
setTimeout(() => {
observer.next('Hi This will invoke Observables')
},5000)
console.log('Observables invoked!');
});
observable.subscribe();
Output:
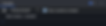
Difference Between JavaScript Promise and Observable
Features | Promises | Observable |
---|---|---|
Definition | Represents a single future value or event. | Represents a stream of multiple values or events. |
Handling values | Resolves to a single value or rejects with an error. | Emits multiple values or completes with an error. |
Execution control | Executes immediately upon creation. | Executes lazily only when subscribed to. |
Value emission | Single value emission. | Multiple values emission over time. |
Asynchronous handling | Handles asynchronous operations. | Handles both synchronous and asynchronous operations |
Completion handling | Resolves or rejects once. | Emits multiple values and completes or errors. |
Chaining | Uses .then() to chain operations. | Uses .pipe() to chain operations. |
Error handling | Uses .catch() or .finally() for error handling. | Uses .catch() or .subscribe() for error handling. |
Backpressure handling | Not applicable. | Supports backpressure handling with operators. |
Cancellation | Not built-in, but can be implemented manually. | Supports cancellation through subscription disposal. |
Note: JavaScript Observables are typically associated with libraries like RxJS, which provide additional functionalities and operators for working with observables.
JavaScript Promises and JavaScript Observables serve different purposes in handling asynchronous operations and data streams:
Promises are suitable for handling a single future value or event. They are executed immediately upon creation, resolve to a single value, and handle asynchronous operations. Promises are ideal for scenarios where you expect a single result or need to chain asynchronous operations.
Observables, often associated with libraries like RxJS, represent a stream of multiple values or events. They can emit multiple values over time and handle both synchronous and asynchronous operations. Observables are useful for scenarios where you have continuous data streams, such as real-time updates or user interactions.
Both Promises and Observables offer error-handling mechanisms, but Observables provide additional features like backpressure handling and the ability to cancel subscriptions. Promises are simpler to use and have native support in JavaScript, while Observables provide more advanced capabilities and a rich set of operators for manipulating data streams.