Difference Between Const vs Readonly vs Static in C#
- The Tech Platform
- May 21, 2022
- 2 min read
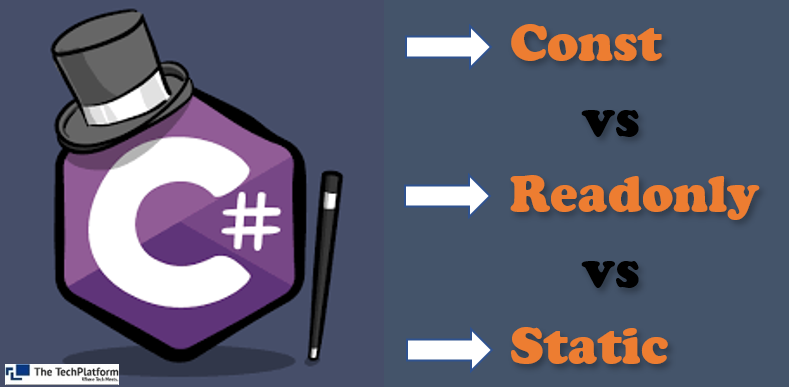
Const:
Constant fields or local variables must be assigned a value at the time of declaration and after that, they cannot be modified. By default constant are static, hence you cannot define a constant type as static. A const field is a compile-time constant. A constant field or local variable can be initialized with a constant expression which must be fully evaluated at compile time.
public const int X = 10;
Example:
using System;
namespace ConstantDemo
{
class Constant
{
// Initializing const variable.
public const double PI = Math.PI;
}
class Program
{
static void Main(string[] args)
{
Console.WriteLine($"PI = {Constant.PI}");
Console.ReadLine();
}
}
}
Output:

Readonly:
A readonly field can be initialized either at the time of declaration or within the constructor of the same class. Therefore, readonly fields can be used for run-time constants.
class MyClass
{
readonly int X = 10; // initialized at the time of declaration
readonly int X1;
public MyClass(int x1)
{
X1 = x1; // initialized at run time
}
}
Example:
using System;
namespace ReadOnlyDemo
{
class User
{
public readonly double id = 123;
public readonly DateTime currentDate;
public User()
{
currentDate = DateTime.Now;
// Value can be changed inside the default/parameterized constructor
id = 456;
}
}
class Program
{
static void Main(string[] args)
{
// readonly int localVariable = 10;
// error : The modifier readonly is not valid for this item
User user = new User();
Console.WriteLine($"Id = {user.id} CurrentDate :{user.currentDate}");
Console.ReadLine();
}
}
}
Output:

Static:
The static keyword is used to specify a static member, which means static members are common to all the objects and they do not tie to a specific object. This keyword can be used with classes, fields, methods, properties, operators, events, and constructors, but it cannot be used with indexers, destructors, or types other than classes.
class MyClass
{
static int X = 10;
int Y = 20;
public static void Show()
{
Console.WriteLine(X);
Console.WriteLine(Y); //error, since you can access only static members
}
}
Example:
using System;
namespace StaticDemo
{
public class User
{
// static variable
public static int userId = 12003;
// instance variable
public string userName = "Sonam Khan";
}
class Program
{
static void Main(string[] args)
{
//Static members can access directly by the class name.
Console.WriteLine($"Id= {User.userId}");
//error, because we can access only static variable by using class name.
//Console.WriteLine($"{User.name}");
//To access instance variable we have to create object of the class.
User objUser = new User();
Console.WriteLine($"Name = {objUser.userName}");
Console.ReadLine();
}
}
}
Output:

Difference Between Const, Readonly and Static
Const | Readonly | Static |
Const members can be accessed using ClassName.ConstVariableName, but cannot be accessed using object. | Readonly members can be accessed using object, but not ClassName.ReadOnlyVariableName. | Static members can be accessed using ClassName.StaticMemberName, but cannot be accessed using object. |
The constant fields must be initialized at the time of declaration. Therefore, const variables are used for compile-time constants. | Readonly fields can be initialized at declaration or in the constructor. Therefore, readonly variables are used for the run-time constants. | Static members can only be accessed within the static methods. The non-static methods cannot access static members |
Declred using the const keyword. By default a const is static that cannot be changed. | Declared using the readonly keyword. | Declared using the static keyword. |
Only the class level fields or variables can be constant. | Only the class level fields can be readonly. The local variables of methods cannot be readonly. | Classes, constructors, methods, variables, properties, event and operators can be static. The struct, indexers, enum, destructors, or finalizers cannot be static. |
Constant variables cannot be modified after declaration. | Readonly variable cannot be modified at run-time. It can only be initialized or changed in the constructor. | Value of the static members can be modified using ClassName.StaticMemberName. |
The Tech Platform
Comments