In this tutorial, we are going to create a full-screen slider using HTML, CSS3 and jQuery. You can us it for product presentations on your shop website.
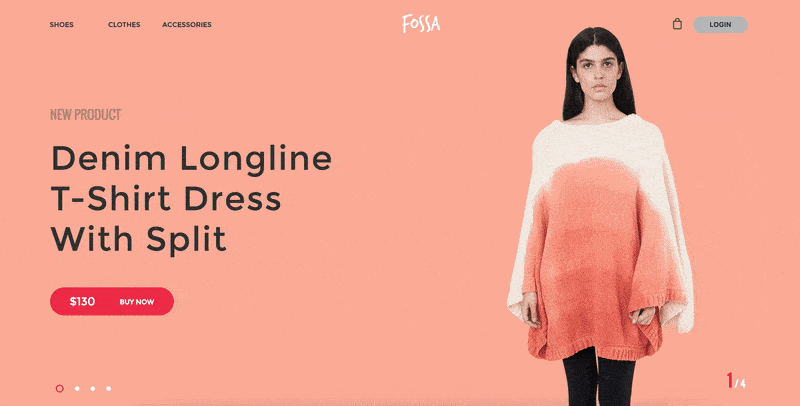
In this tutorial, we use Google Fonts, specifically Montserrat and Oswald. Make sure to include them before you start.
HTML
The first step is to create an HTML structure. Here’s what we need:
Navigation menu
A wrapper div for slider with a class name css-slider-wrapper
Four slides; each has elements such as buttons/images/text
Slider pagination
Here is the markup:
<!-- Navigation -->
<div class="navigation">
<div class="navigation-left">
<a href="#">Shoes</a>
<a href="#">Clothes</a>
<a href="#">Accessories</a>
</div>
<div class="navigation-center">
<img src="images/logo.png" alt="">
</div>
<div class="navigation-right">
<a href="#"><img src="images/shopping-bag.png" alt=""></a>
<button class="login-btn" href="#">Login</button>
</div>
</div>
<!-- Slider Wrapper -->
<div class="css-slider-wrapper">
<input type="radio" name="slider" class="slide-radio1" checked id="slider_1">
<input type="radio" name="slider" class="slide-radio2" id="slider_2">
<input type="radio" name="slider" class="slide-radio3" id="slider_3">
<input type="radio" name="slider" class="slide-radio4" id="slider_4">
<!-- Slider Pagination -->
<div class="slider-pagination">
<label for="slider_1" class="page1"></label>
<label for="slider_2" class="page2"></label>
<label for="slider_3" class="page3"></label>
<label for="slider_4" class="page4"></label>
</div>
<!-- Slider #1 -->
<div class="slider slide-1">
<img src="images/model-1.png" alt="">
<div class="slider-content">
<h4>New Product</h4>
<h2>Denim Longline T-Shirt Dress With Split</h2>
<button type="button" class="buy-now-btn" name="button">$130</button>
</div>
<div class="number-pagination">
<span>1</span>
</div>
</div>
<!-- Slider #2 -->
<div class="slider slide-2">
<img src="images/model-2.png" alt="">
<div class="slider-content">
<h4>New Product</h4>
<h2>Denim Longline T-Shirt Dress With Split</h2>
<button type="button" class="buy-now-btn" name="button">$130</button>
</div>
<div class="number-pagination">
<span>2</span>
</div>
</div>
<!-- Slider #3 -->
<div class="slider slide-3">
<img src="images/model-3.png" alt="">
<div class="slider-content">
<h4>New Product</h4>
<h2>Denim Longline T-Shirt Dress With Split</h2>
<button type="button" class="buy-now-btn" name="button">$130</button>
</div>
<div class="number-pagination">
<span>3</span>
</div>
</div>
<!-- Slider #4 -->
<div class="slider slide-4">
<img src="images/model-4.png" alt="">
<div class="slider-content">
<h4>New Product</h4>
<h2>Denim Longline T-Shirt Dress With Split</h2>
<button type="button" class="buy-now-btn" name="button">$130</button>
</div>
<div class="number-pagination">
<span>4</span>
</div>
</div>
</div>
Cool! Let’s style it now.
Online Email Template Builder
With Postcards you can create and edit email templates online without any coding skills! Includes more than 100 components to help you create custom emails templates faster than ever before.
CSS
First, let’s add basic styling to the body.
* {
box-sizing: border-box;
}
body {
overflow: hidden;
width: 100%;
height: 100%;
padding: 0;
margin: 0;
font-family: 'Montserrat', sans-serif;
}
Now let’s style the navigation menu; we’ll make it full width with some padding. We’ll use flexbox to align elements within it, which we’ll also style.
/* Navigation */
.navigation {
position: absolute;
width: 100%;
height: 100px;
padding: 0 100px;
display: flex;
align-items: center;
justify-content: space-between;
z-index: 1;
}
.navigation-left {
margin-left: -33px;
}
.navigation-left a {
text-decoration: none;
text-transform: uppercase;
color: #333745;
font-size: 12px;
font-weight: bold;
width: 107px;
height: 30px;
border: 2px solid transparent;
border-radius: 15px;
display: inline-block;
text-align: center;
line-height: 25px;
transition: all .2s;
}
.navigation-left a:hover,
.navigation-left a:focus {
border-color: rgb(234, 46, 73);
background-color: rgba(44, 45, 47, 0);
}
.navigation-center {
margin-right: 85px;
}
.navigation-right {
display: flex;
align-items: center;
}
.login-btn {
background-color: #b8b8b9;
width: 97px;
height: 30px;
display: inline-block;
text-align: center;
text-decoration: none;
font-size: 12px;
font-weight: bold;
border-radius: 15px;
border: none;
color: #333745;
text-transform: uppercase;
margin-left: 20px;
transition: all .2s;
cursor: pointer;
}
.login-btn:hover {
transform: scale(1.06);
}
Cool! Now let’s style the slider wrapper.
It will be a full-screen slider so we set it to position absolute.
.css-slider-wrapper {
display: block;
background: #FFF;
overflow: hidden;
position: absolute;
left: 0;
right: 0;
top: 0;
bottom: 0;
}
Let’s also style the slides, the common class we’ve applied and the class for each slide where we add a background color to each one.
/* Slider */
.slider {
width: 100%;
height: 100%;
position: absolute;
left: 0;
top: 0;
opacity: 1;
z-index: 0;
display: flex;
flex-direction: row;
flex-wrap: wrap;
align-items: center;
justify-content: center;
align-content: center;
-webkit-transition: -webkit-transform 1600ms;
transition: -webkit-transform 1600ms, transform 1600ms;
-webkit-transform: scale(1);
transform: scale(1);
}
/* Slides Background Color */
.slide-1 {
background: #fbad99;
left: 0;
}
.slide-2 {
background: #a9785c;
left: 100%
}
.slide-3 {
background: #9ea6b3;
left: 200%
}
.slide-4 {
background: #b1a494;
left: 300%;
}
.slider {
display: flex;
justify-content: flex-start;
}
.slider-content {
width: 635px;
padding-left: 100px;
}
Now style elements within each slide. Remember we have some text, a button and an image.
/* Slider Inner Slide Effect */
.slider h2 {
color: #333333;
font-weight: 900;
text-transform: capitalize;
font-size: 60px;
font-weight: 300;
line-height: 1.2;
opacity: 0;
-webkit-transform: translateX(500px);
transform: translateX(500px);
margin-top: 0;
letter-spacing: 2px;
}
.slider h4 {
font-size: 22px;
font-family: "Oswald";
color: rgba(51, 51, 51, 0.349);
font-weight: bold;
text-transform: uppercase;
line-height: 1.2;
opacity: 0;
-webkit-transform: translateX(500px);
transform: translateX(500px);
}
.slider > img {
position: absolute;
right: 10%;
bottom: 0;
height: 100%;
opacity: 0;
-webkit-transform: translateX(500px);
transform: translateX(500px);
}
.slide-1 > img {
right: 0;
}
.buy-now-btn {
background-color: #ea2e49;
width: 220px;
height: 50px;
border-radius: 30px;
border: none;
font-family: Montserrat;
font-size: 20px;
font-weight: 100;
color: #fff;
text-align: left;
padding-left: 35px;
position: relative;
cursor: pointer;
transition: all .2s;
}
.buy-now-btn:hover {
box-shadow: 0px 0px 60px -17px rgba(51,51,51,1);
}
.buy-now-btn:after {
content: 'Buy Now';
font-size: 12px;
font-weight: bold;
text-transform: uppercase;
position: absolute;
right: 35px;
top: 18px;
}
.slider .buy-now-btn:focus,
.navigation .login-btn:focus {
outline: none;
}
Let’s also make sure that when the slide changes, elements within it — such as text and images — animate.
/* Animations */
.slider h2 {
-webkit-transition: opacity 800ms, -webkit-transform 800ms;
transition: transform 800ms, opacity 800ms;
-webkit-transition-delay: 1s; /* Safari */
transition-delay: 1s;
}
.slider h4 {
-webkit-transition: opacity 800ms, -webkit-transform 800ms;
transition: transform 800ms, opacity 800ms;
-webkit-transition-delay: 1.4s; /* Safari */
transition-delay: 1.4s;
}
.slider > img {
-webkit-transition: opacity 800ms, -webkit-transform 800ms;
transition: transform 800ms, opacity 800ms;
-webkit-transition-delay: 1.2s; /* Safari */
transition-delay: 1.2s;
}
We also have number pagination in the right corner of each slide. Let’s style that, too.
Create Websites with Our Online Builders
With Startup App and Slides App you can build unlimited websites using the online website editor which includes ready-made designed and coded elements, templates and themes.
/* Number Pagination */
.number-pagination {
position: absolute;
bottom: 30px;
right: 100px;
font-family: "Oswald";
font-weight: bold;
}
.number-pagination span {
font-size: 30px;
color: #ea2e49;
letter-spacing: 4px;
}
.number-pagination span:after {
content: "/4";
font-size: 16px;
color: #fff;
}
Awesome! Now, style the slider pagination.
/* Slider Pagger */
.slider-pagination {
position: absolute;
bottom: 30px;
width: 575px;
left: 100px;
z-index: 1000;
display: flex;
align-items: center;
}
.slider-pagination label {
width: 8px;
height: 8px;
border-radius: 50%;
display: inline-block;
background: #fff;
margin: 0 10px;
cursor: pointer;
}
Ok, cool! Now here comes the fun part and where the magic happens.
Note that we’re going to use :checked pseudo class to control sliding.
The :checked pseudo-class selects elements when they are in the selected state. It is only associated with input (input type=”text”/) elements of type radio and checkbox. The :checked pseudo-class selector matches radio and checkbox input types when checked or toggled to an on state. If they are not selected or checked, there is no match.
/* Slider Pagger Event */
.slide-radio1:checked ~ .slider-pagination .page1,
.slide-radio2:checked ~ .slider-pagination .page2,
.slide-radio3:checked ~ .slider-pagination .page3,
.slide-radio4:checked ~ .slider-pagination .page4 {
width: 14px;
height: 14px;
border: 2px solid #ea2e49;
background: transparent;
}
/* Slider Slide Effect */
.slide-radio1:checked ~ .slider {
-webkit-transform: translateX(0%);
transform: translateX(0%);
}
.slide-radio2:checked ~ .slider {
-webkit-transform: translateX(-100%);
transform: translateX(-100%);
}
.slide-radio3:checked ~ .slider {
-webkit-transform: translateX(-200%);
transform: translateX(-200%);
}
.slide-radio4:checked ~ .slider {
-webkit-transform: translateX(-300%);
transform: translateX(-300%);
}
.slide-radio1:checked ~ .slide-1 h2,
.slide-radio2:checked ~ .slide-2 h2,
.slide-radio3:checked ~ .slide-3 h2,
.slide-radio4:checked ~ .slide-4 h2,
.slide-radio1:checked ~ .slide-1 h4,
.slide-radio2:checked ~ .slide-2 h4,
.slide-radio3:checked ~ .slide-3 h4,
.slide-radio4:checked ~ .slide-4 h4,
.slide-radio1:checked ~ .slide-1 > img,
.slide-radio2:checked ~ .slide-2 > img,
.slide-radio3:checked ~ .slide-3 > img,
.slide-radio4:checked ~ .slide-4 > img {
-webkit-transform: translateX(0);
transform: translateX(0);
opacity: 1
}
Awesome! Here’s how our full-screen slider looks:
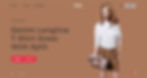
Looks great, right? But let’s make it responsive.
/* Responsive */
@media only screen and (max-width: 768px) {
.slider h2 {
font-size: 20px;
}
.slider h4 {
font-size: 16px;
}
.slider-content {
padding: 0 2%
}
.navigation {
padding: 0 2%;
}
.navigation-left {
display: none;
}
.number-pagination {
right: 2%;
}
.slider-pagination {
left: 2%;
}
.slider .buy-now-btn {
padding: 0 15px;
width: 175px;
height: 42px;
}
.slider .buy-now-btn:after {
top: 15px;
}
.slider > img {
right: 2%;
}
.slide-1 > img {
right: -110px;
}
}
Javascript (jQuery)
We have one more thing to do. Our slider only changes slides when we click on pagination bullets. We’ll use some Javascript to make the slider to change automatically. Make sure to include jQuery in your project first and then add this line to your javascript file.
var TIMEOUT = 6000;
var interval = setInterval(handleNext, TIMEOUT);
function handleNext() {
var $radios = $('input[class*="slide-radio"]');
var $activeRadio = $('input[class*="slide-radio"]:checked');
var currentIndex = $activeRadio.index();
var radiosLength = $radios.length;
$radios
.attr('checked', false);
if (currentIndex >= radiosLength - 1) {
$radios
.first()
.attr('checked', true);
} else {
$activeRadio
.next('input[class*="slide-radio"]')
.attr('checked', true);
}
}
Source: designmodo
The Tech Platform