Checked and Unchecked Exception in Java
- The Tech Platform
- Feb 9, 2022
- 2 min read
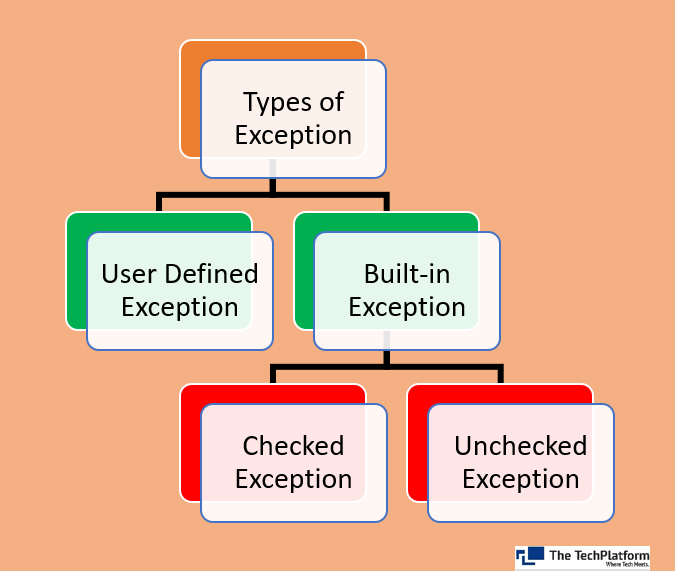
Read - Exception Handling in Java
Checked Exception
These are the exceptions that are checked at compile time. If some code within a method throws a checked exception, then the method must either handle the exception or it must specify the exception using the throws keyword.
Example:
using System;
namespace CSharpProgram
{
class Program
{
static void Main(string[] args)
{
int val = int.MaxValue;
Console.WriteLine(val + 2);
}
}
}
output:

Example using Checked :
This program throws an exception and stops program execution.
using System;
namespace CSharpProgram
{
class Program
{
static void Main(string[] args)
{
checked
{
int val = int.MaxValue;
Console.WriteLine(val + 2);
}
}
}
}
output:

Advantages:
Fail-Fast Development: Exceptional situations, such as possible IO errors, surface at compile time. Ignoring an unchecked exception becomes a concious decision as opposed to, for example, custom return values indicating errors, or unchecked exceptions.
Contract: The fact that a method throws a certain exception can be an essential part of the contract. The throws declaration is therefore as important as the types of the return value and parameters. Apart from the static analysis it enables, it also serves a documentational purpose for the programmer.
Disadvantages:
Extensibility: You can't add throws SomeCheckedException to a method signature without breaking client code, wihch restricts the evolution of APIs. You're obliged to either throw an unchecked exception (even it otherwise has the characteristics of a checked exception) or create a new method and deprecate the original one.
Misuse: Many checked exceptions, including some in the the Java API, should have been implemented as unchecked exceptions. Such misuse leads to frustration and in the end catch blocks that either ignores them or rethrows them as RuntimeExceptions which in turn are never caught.
Propagation: One key feature of exceptions is that they can be thrown at a low level, bubbled up and dealt with at a high level---"throw early, catch late". The issue with checked exceptions is that every intermediate level needs to declare the exception to be thrown. This is particularly problematic when the intermediate levels include interfaces that doesn't allow checked exceptions to be thrown.
Unchecked Exception
These are the exceptions that are not checked at compile time. In C++, all exceptions are unchecked, so it is not forced by the compiler to either handle or specify the exception. It is up to the programmers to be civilized, and specify or catch the exceptions. In Java exceptions under Error and RuntimeException classes are unchecked exceptions, everything else under throwable is checked.
Example:
using System;
namespace CSharpProgram
{
class Program
{
static void Main(string[] args)
{
unchecked
{
int val = int.MaxValue;
Console.WriteLine(val + 2);
}
}
}
}
Output:

Difference Between Checked and Unchecked Exception
Checked Unchecked
Checked Exception are the sub-class of exception class | Unchecked Exception are Runtime Exception |
In this, Java Virtual Machine require the exception to be caught or handled | In this, Java Virtual Machine does not require the exception to be caught or handled |
They are checked at runtime of the program | They are checked at compile time of program |
Checked Exception occurs when the chances of failure are too high | Unchecked Exception occurs mostly due to programming mistakes |
The Program gives a compilation error if method throws a checked exception and the compile is not able to handle the exception on its own | The Program compiles fine because the exception escape the notice of compiler: Exceptions occurs due to error in programming logic. |
They can be ignored | They cannot be ignored. |
Common Checked Exception are: IOException, DataSccessException, IllegalAccessException, InterruptedException etc. | Common Unchecked Exception include ArithmeticException, InvalidClassException, NullPointerException etc. |
They can be handed using try, catch and finally | They can also be handled using try, catch and finally |
The Tech Platform
Comments