Building GraphQL API With .Net 5 — EF Core And Hot Chocolate
- The Tech Platform
- Feb 24, 2022
- 6 min read
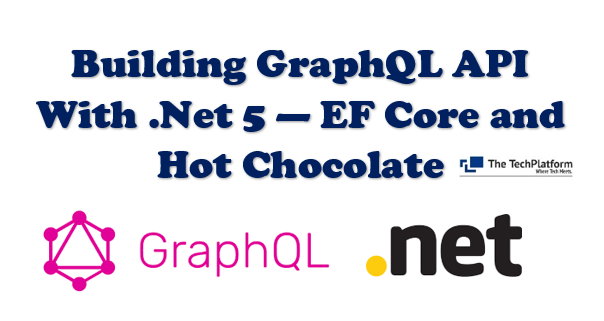
Introduction
GraphQL is an open-source query language, originally developed by Facebook. It was in the year 2012 that Facebook chose to modify its applications to improve execution and productivity. It was the point at which Facebook’s portable methodology wasn’t working on account of high organization use. Enhancement techniques utilizing reserving may have improved the exhibition, yet since the application was excessively unpredictable, it was believed that the information bringing system itself ought to be changed. Here’s the place where GraphQL came in, and today it has gotten amazingly mainstream among the advancement local area around the world. GraphQL, created by Facebook in 2012 and publicly released in 2015, is presently kept up by the GraphQL Foundation. This article examines the highlights and advantages of GraphQL and afterward shows how one can function with GraphQL in .Net 5.0.
Prerequisites
Visual Studio 2019
.Net 5.0 SDK
Why do we need GraphQL?
GraphQL is a JSON-like query language for APIs as well as a server-side runtime for executing your queries. Unlike REST where the request and response are defined by the API, in GraphQL, the client has complete control over what data the API should return. You can integrate GraphQL with ASP.NET, ASP.NET Core, Java, Python,Node.js, etc.
In the event that you are dealing with an application that uses RESTful design, the endpoints may develop over the long haul, and keeping up with them may turn into a bad dream. Despite what might be expected, with GraphQL, you simply need one endpoint programming api/graphql, and there’s nothing more to it. This is another critical distinction between REST and GraphQL.
In utilizing GraphQL you need fewer roundtrips to the worker, i.e., you need fewer to and fro calls to the worker to get all the information you need. With REST, you will have a few endpoints like programming api/understudies, api/educators, api/groups, and so on
In contrast to REST, when utilizing GraphQL, you won’t ever have nearly nothing or a lot of information — you can characterize your questions and all information you need.
When utilizing GraphQL, you need not stress over forming. The truth of the matter is that GraphQL doesn’t require forming and as long as you don’t erase fields from the sorts, the customers or shoppers of the API wouldn’t break.
Like Swagger creates documentation for the REST endpoints, GraphQL can likewise produce documentation for the GraphQL endpoint.
What is HotChocolate?
HotChocolate is a .Net GraphQL Platform that can help you build a GraphQL layer over your existing and new application. hot chocolate is very easy to set up and takes the clutter away from writing the GraphQL Schemas.
In-depth understanding of GraphQL
Schema
A GraphQL schema is a description of the data clients can request from a GraphQL API. It also defines the queries and mutation functions that the client can use to read and write data from the GraphQL server. In other words, you specify your client or application UI data requirements in your GraphQL schema.
The schema contains a Query and Mutation
Query fetch data — like a GET in the rest
Mutation changes data — like DELETE or a POST in rest.
Some people use the word query to refer to both GraphQL queries and mutations.
Configuring the GraphQL Middleware
Setup the Project — Choose the Empty Asp.Net core project.
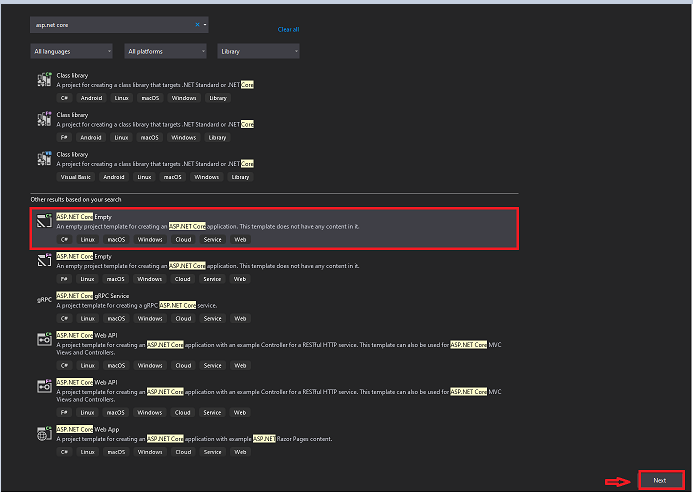
Fig-1
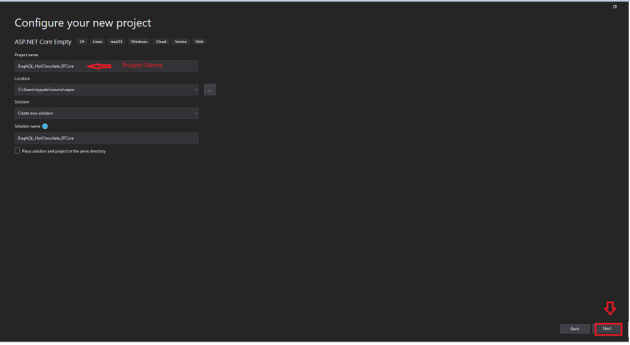
Fig-2
Packages used to configure the EF Core and GraphQL
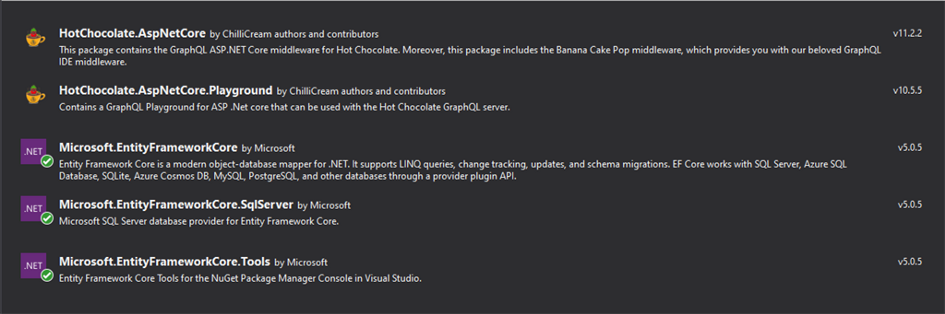
Fig-3
Create an empty folder Model and inside that will add the respective Model and Data context.

Fig-4
Create a Model class to perform the Database operation using Entity Framework Core using Code first approach
Employee.cs
using System;
using System.Collections.Generic;
using System.ComponentModel.DataAnnotations;
using System.Linq;
using System.Threading.Tasks;
namespace GraphQL_HotChoclate_EFCore.Models
{
public class Employee
{
[Key]
public int Id { get; set; }
public string Name { get; set; }
public string Designation { get; set; }
}
}
Define all the classes inside this DatabaseContext class and add the static data by using the seed data mechanism.
DatabaseContext.cs
using Microsoft.EntityFrameworkCore;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
namespace GraphQL_HotChoclate_EFCore.Models
{
public class DatabaseContext : DbContext
{
public DatabaseContext(DbContextOptions<DatabaseContext> options) : base(options)
{
}
public DbSet<Employee> Employees { get; set; }
#region Seed Data
protected override void OnModelCreating(ModelBuildermodelBuilder)
{
modelBuilder.Entity<Employee>().HasData(
new Employee
{
Id=1,
Name="Jay krishna Reddy",
Designation="Full Stack Developer"
},
new Employee
{
Id=2,
Name="JK",
Designation="SSE"
},
new Employee
{
Id=3,
Name="Jay",
Designation="Software Engineer"
},
new Employee
{
Id=4,
Name="krishna Reddy",
Designation="Database Developer"
},
new Employee
{
Id=5,
Name="Reddy",
Designation="Cloud Engineer"
}
);
}
#endregion
}
}
Add the connection string in the appsettings.json file
appsettings.json
{
"Logging":
{
"LogLevel":
{
"Default": "Information",
"Microsoft": "Warning",
"Microsoft.Hosting.Lifetime": "Information"
}
},
"AllowedHosts": "*",
"ConnectionStrings":
{
"myconn": "server="Your server name";
database=GraphQL;Trusted_Connection=True;"
}
add the SQL server configuration setup in a Startup.cs file under the Configure services method.
Startup.cs
using GraphQL_HotChoclate_EFCore.GraphQL;
using GraphQL_HotChoclate_EFCore.Models;
using GraphQL_HotChoclate_EFCore.Services;
using HotChocolate;
using HotChocolate.AspNetCore;
using HotChocolate.AspNetCore.Playground;
using Microsoft.AspNetCore.Builder;
using Microsoft.AspNetCore.Hosting;
using Microsoft.AspNetCore.Http;
using Microsoft.EntityFrameworkCore;
using Microsoft.Extensions.Configuration;
using Microsoft.Extensions.DependencyInjection;
using Microsoft.Extensions.Hosting;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
namespace GraphQL_HotChoclate_EFCore
{
public class Startup
{
public IConfigurationConfiguration { get; }
// This method gets called by the runtime. Use this method to
add services to the container.
// For more information on how to configure your application,
visit https://go.microsoft.com/fwlink/?LinkID=398940
public Startup(IConfigurationconfiguration)
{
Configuration=configuration;
}
public void ConfigureServices(IServiceCollectionservices)
{
#region Connection String
services.AddDbContext<DatabaseContext>(item=>item
.UseSqlServer(Configuration.GetConnectionString("myconn")));
#endregion
}
// This method gets called by the runtime. Use this method to
configure the HTTP request pipeline.
public void Configure(IApplicationBuilderapp,
IWebHostEnvironmentenv)
{
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
}
app.UseRouting();
app.UseEndpoints(endpoints=>
{
endpoints.MapGet("/", async context=>
{
await context.Response.WriteAsync("Hello World!");
});
});
}
}
Create the database and tables in the SQL server by using the below commands.
Add-Migration ‘Migration Name’ — To create the Script inside the solution project
update-database — To execute the generated script in the SQL server
Execute the Table in SQL Server to check the data
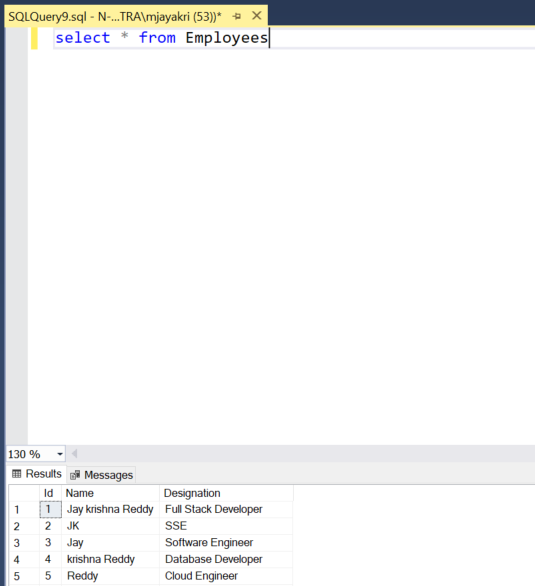
Fig-5
Now, will add the services to have all our business logic in them.
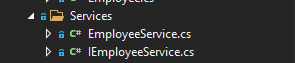
Fig-6
EmployeeService.cs
using GraphQL_HotChoclate_EFCore.Models;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
using Microsoft.EntityFrameworkCore;
namespace GraphQL_HotChoclate_EFCore.Services
{
public class EmployeeService : IEmployeeService
{
#region Property
private readonly DatabaseContext_dbContext;
#endregion
#region Constructor
public EmployeeService(DatabaseContextdatabaseContext)
{
_dbContext=databaseContext;
}
#endregion
public async Task<Employee> Create(Employeeemployee)
{
var data = new Employee
{
Name=employee.Name,
Designation=employee.Designation
};
await _dbContext.Employees.AddAsync(data);
await _dbContext.SaveChangesAsync();
return data;
}
public async Task<bool> Delete(DeleteVMdeleteVM)
{
var employee = await _dbContext.Employees
.FirstOrDefaultAsync(c=>c.Id==deleteVM.Id);
if(employee is notnull)
{
_dbContext.Employees.Remove(employee);
await _dbContext.SaveChangesAsync();
return true;
}
return false;
}
public IQueryable<Employee> GetAll()
{
return _dbContext.Employees.AsQueryable();
}
}
public class DeleteVM
{
public int Id { get; set; }
}
IEmployeeService.cs
using GraphQL_HotChoclate_EFCore.Models;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
namespace GraphQL_HotChoclate_EFCore.Services
{
public interface IEmployeeService
{
Task<Employee> Create(Employeeemployee);
Task<bool> Delete(DeleteVMdeleteVM);
IQueryable<Employee> GetAll();
}
}
add this Employeeservice dependency in the startup. cs under the ConfigureServices Method.
services.AddScoped<IEmployeeService,EmployeeService>();
After completion of the table creation, Now let’s integrate the GraphQL in step by step process.
create an empty folder named GraphQL where will add the Query class and Mutation class to perform the GraphQL operations with respect to its GraphQLTypes.
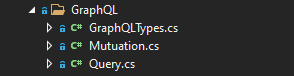
Fig-7
Define the Employee services in the Query and Mutation class
Query.cs
using GraphQL_HotChoclate_EFCore.Models;
using GraphQL_HotChoclate_EFCore.Services;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
namespace GraphQL_HotChoclate_EFCore.GraphQL
{
public class Query
{
#region Property
private readonly IEmployeeService_employeeService;
#endregion
#region Constructor
public Query(IEmployeeServiceemployeeService)
{
_employeeService=employeeService;
}
#endregion
public IQueryable<Employee> Employees=>_employeeService.GetAll();
}
}
Mutation.cs
using GraphQL_HotChoclate_EFCore.Models;
using GraphQL_HotChoclate_EFCore.Services;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
namespace GraphQL_HotChoclate_EFCore.GraphQL
{
public class Mutuation
{
#region Property
private readonly IEmployeeService_employeeService;
#endregion
#region Constructor
public Mutuation(IEmployeeServiceemployeeService)
{
_employeeService=employeeService;
}
#endregion
public async Task<Employee> Create(Employeeemployee)
=>await _employeeService.Create(employee);
public async Task<bool> Delete(DeleteVMdeleteVM)
=>await _employeeService.Delete(deleteVM);
}
}
GraphQLTypes.cs
Invoke the Employee class inside the object type by using the Hot chocolate library.
using GraphQL_HotChoclate_EFCore.Models;
using HotChocolate.Types;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
namespace GraphQL_HotChoclate_EFCore.GraphQL
{
public class GraphQLTypes :ObjectType<Employee>
{
}
}
Configure the GraphQL Middleware in the Startup.cs file by adding the GraphQL Model class and hot chocolate playground is the tool that will help to query the data (GraphQL).
Startup.cs — — final version
using GraphQL_HotChoclate_EFCore.GraphQL;
using GraphQL_HotChoclate_EFCore.Models;
using GraphQL_HotChoclate_EFCore.Services;
using HotChocolate;
using HotChocolate.AspNetCore;
using HotChocolate.AspNetCore.Playground;
using Microsoft.AspNetCore.Builder;
using Microsoft.AspNetCore.Hosting;
using Microsoft.AspNetCore.Http;
using Microsoft.EntityFrameworkCore;
using Microsoft.Extensions.Configuration;
using Microsoft.Extensions.DependencyInjection;
using Microsoft.Extensions.Hosting;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
namespace GraphQL_HotChoclate_EFCore
{
public class Startup
{
public IConfigurationConfiguration { get; }
// This method gets called by the runtime. Use this method to add services to the container.
// For more information on how to configure your application, visit https://go.microsoft.com/fwlink/?LinkID=398940
public Startup(IConfigurationconfiguration)
{
Configuration=configuration;
}
public void ConfigureServices(IServiceCollectionservices)
{
#region Connection String
services.AddDbContext<DatabaseContext>(item=>item.UseSqlServer(Configuration.GetConnectionString("myconn")));
# endregion
services.AddScoped<Query>();
services.AddScoped<Mutuation>();
services.AddScoped<IEmployeeService,EmployeeService>();
services.AddGraphQL(c=>SchemaBuilder.New()
.AddServices(c).AddType<GraphQLTypes>()
.AddQueryType<Query>()
.AddMutationType<Mutuation>()
.Create());
}
// This method gets called by the runtime. Use this method to configure the HTTP request pipeline.
public void Configure(IApplicationBuilderapp, IWebHostEnvironmentenv)
{
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
app.UsePlayground(new PlaygroundOptions
{
QueryPath="/api",
Path="/playground"
});
}
app.UseGraphQL("/api");
app.UseRouting(); app.UseEndpoints(endpoints=>
{
endpoints.MapGet("/", async context=>
{
await context.Response.WriteAsync("Hello World!");
});
});
}
}
Run the project
Add the playground tag to the URL — https://localhost:44330/playground/ this playground will actually take us to the hot-chocolate tool in which we can do our querying (GraphQL).
Overview of the HotChocolate Platform
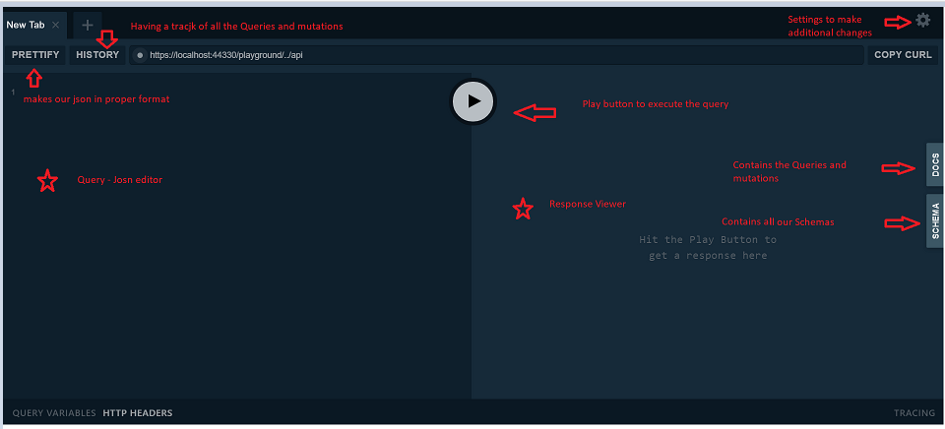
Fig-8
Fetch all the list of employees by using the query
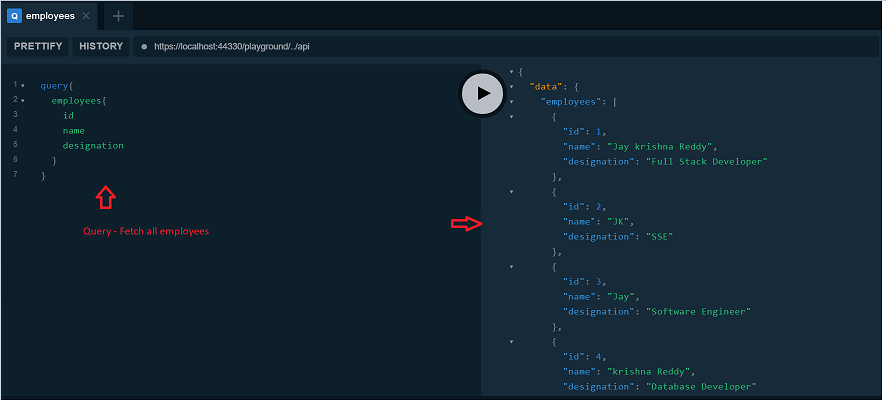
Fig-9
Create the user by using the mutation
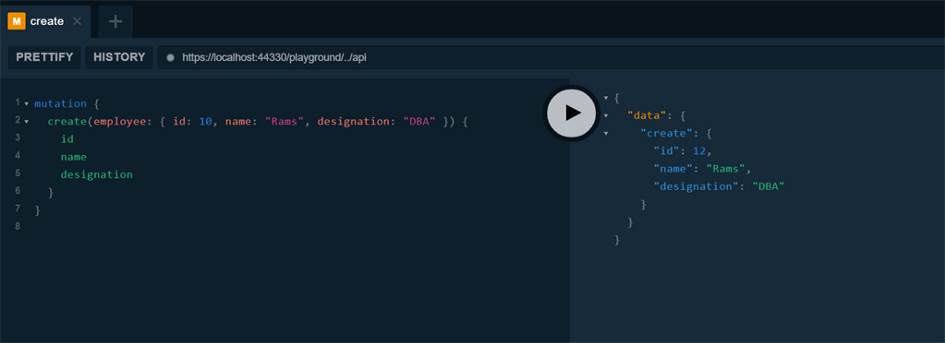
Fig-10
Delete the user
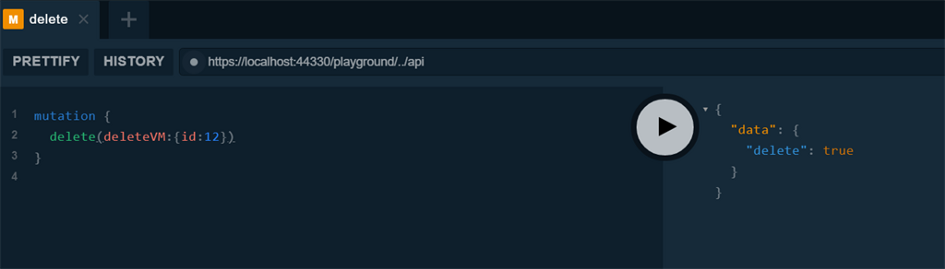
Fig-11
Conclusion
In this article, we have covered all the topics related to GraphQl and its usage and the integration with .Net 5.0, EF Core with performing the CRUD Operations. Hope this gives you a clear idea in understanding and as well as in implementation.
Source: Medium - Jay Krishna Reddy
The Tech Platform
"Edulete is a competitive exam preparation product created with the intention of improving learning outcomes by tapping into key factors of human psychology which can augment learning. Our app is designed to help you participate in gamified tests / quizzes and compete against India’s best students We curate & host quizzes across educational (IIT JEE, CAT, UPSC, CLAT, CBSE, GMAT etc.) as well as non-educational (IQ, Sports Trivia, GK, Movies etc.) topics in a variety of formats" https://www.edulete.in/