How to avoid fat controllers in ASP.NET Web API
- The Tech Platform
- Mar 25, 2021
- 4 min read
Updated: Feb 10, 2023
In this article, you will learn about the Fat controllers in ASP.NET web API and how to avoid it.
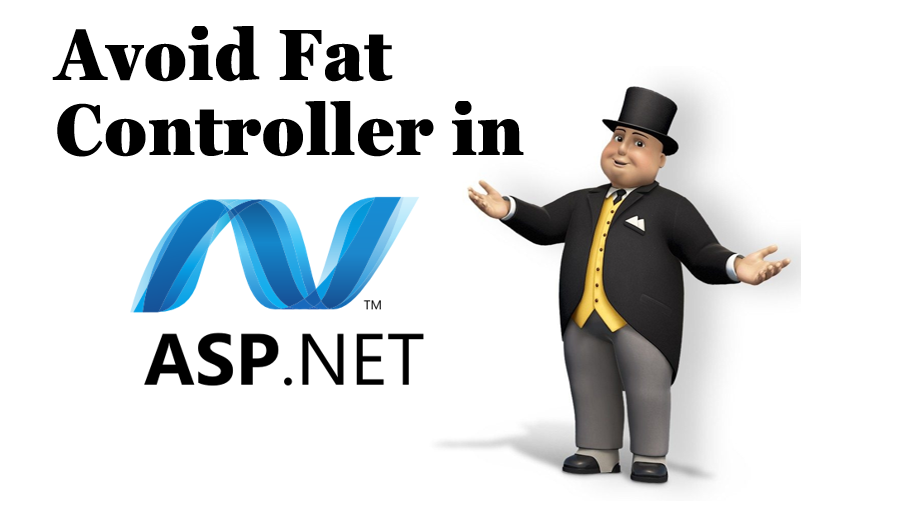
What is Fat controller?
A fat controller is a term used to describe a controller in ASP.NET that has become too complex and contains too much logic. Fat controllers can be identified by having multiple responsibilities, such as handling both data retrieval and validation, and can make code more difficult to maintain and test.
Some common causes of fat controllers include:
Business Logic: Incorporating business logic into the controller rather than abstracting it into a separate layer.
Data Access: Performing data access operations within the controller, such as querying a database or calling a web service, rather than using a repository pattern to abstract away the data access layer.
Validation: Implementing validation logic within the controller rather than using model validation.
Multiple Responsibilities: Handling multiple types of requests within a single controller, making the code difficult to maintain and test.
Fat controllers can lead to increased code complexity, reduced maintainability, and decreased performance. To avoid fat controllers, it is important to follow best practices such as separating business logic, using dependency injection, and utilizing model validation.
Steps to avoid fat controllers in ASP.NET Web API
STEP 1: Separate Business Logic
Use a separate business logic layer to encapsulate complex business logic. For example:
public interface IEmployeeService
{
Employee GetEmployeeById(int id);
IEnumerable<Employee> GetAllEmployees();
void UpdateEmployee(Employee employee);
void AddEmployee(Employee employee);
void DeleteEmployee(int id);
}
STEP 2: Use Dependency Injection
Use dependency injection to pass objects into the controller, rather than creating them within the controller. For example:
public class EmployeeController : ApiController
{
private readonly IEmployeeService _employeeService;
public EmployeeController(IEmployeeService employeeService)
{
_employeeService = employeeService;
}
// Controller methods
}
STEP 3: Implement Model Validation
Use model validation to validate incoming data, rather than relying on validation logic in the controller. For example:
public class Employee
{
[Required]
public string FirstName { get; set; }
[Required]
public string LastName { get; set; }
[Range(0, 999999.99)]
public decimal Salary { get; set; }
}
STEP 4: Refactor Long Methods
If a controller method becomes too long, consider breaking it down into smaller, more focused methods. This makes the code easier to read and maintain.
STEP 5: Use Action Filters
Use action filters to encapsulate common logic, such as logging or authentication, so that it doesn't need to be repeated in each controller method.
public class LogAttribute : ActionFilterAttribute
{
public override void OnActionExecuting(HttpActionContext actionContext){
// Logging logic
}
}
[Log]
public class EmployeeController : ApiController
{
// Controller methods
}
STEP 6: Use Repository Pattern
Use the repository pattern to abstract away the data access layer. This allows the controller to focus on business logic and helps to keep the data access logic out of the controller.
public interface IEmployeeRepository
{
Employee GetEmployeeById(int id);
IEnumerable<Employee> GetAllEmployees();
void UpdateEmployee(Employee employee);
void AddEmployee(Employee employee);
void DeleteEmployee(int id);
}
By following these best practices, you can reduce the size of your controllers, improve code maintainability, and make your ASP.NET Web API application more scalable and reliable.
Conclusion:
Fat controllers are a common issue that can arise when controllers become too complex and take on too many responsibilities. To avoid fat controllers, it is important to follow best practices such as separating business logic, using dependency injection, implementing model validation, refactoring long methods, using action filters, and utilizing the repository pattern to abstract away the data access layer. By following these best practices, you can reduce the size of your controllers, improve code maintainability, and make your ASP.NET Web API application more scalable and reliable.
Frequently Asked Questions
Question 1: What are the consequences of having a fat controller in ASP.NET Web API?
Answer: Fat controllers can lead to increased code complexity, reduced maintainability, and decreased performance. They can also make it more difficult to test the application, since the controller is responsible for multiple types of functionality.
Question 2: How can I avoid having a fat controller in ASP.NET Web API?
Answer: To avoid fat controllers, it is important to follow best practices such as separating business logic, using dependency injection, implementing model validation, refactoring long methods, using action filters, and utilizing the repository pattern to abstract away the data access layer.
Question 3: What is the repository pattern and why is it important for avoiding fat controllers?
Answer: The repository pattern is a design pattern that provides a unified interface to access data in a persistence store, such as a database. By using the repository pattern, you can abstract away the data access layer and keep it out of the controller, reducing the size and complexity of the controller.
Question 4: What is the role of dependency injection in avoiding fat controllers?
Answer: Dependency injection is a design pattern that allows objects to be passed into a class, rather than creating them within the class. By using dependency injection, you can separate the concerns of the controller and make it easier to test and maintain.
Question 5: What are action filters and how can they be used to avoid fat controllers?
Answer: Action filters are attributes that can be applied to a controller or an individual action method to encapsulate common logic, such as logging or authentication. By using action filters, you can reduce the size of your controllers and make the code easier to maintain.
Comentarios