Arrays in C/C++
- The Tech Platform
- Nov 22, 2021
- 2 min read
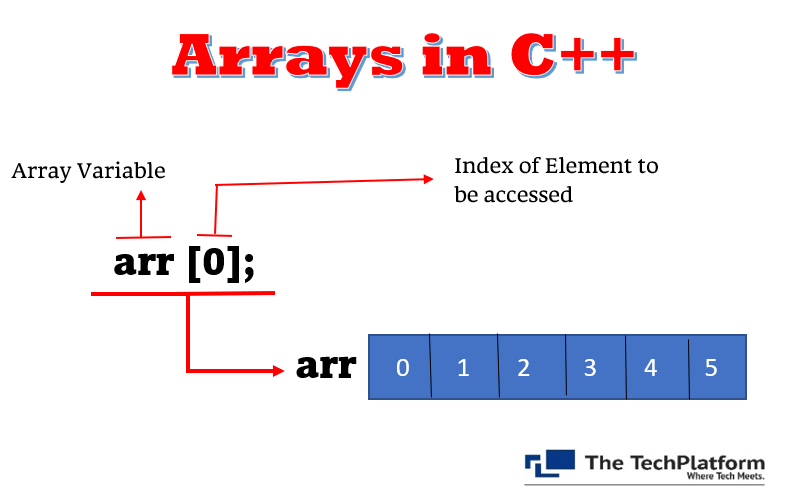
Arrays
An array is a collection of similar data items stored at contiguous memory locations and elements can be accessed randomly using indices of an array. They can be used to store collection of primitive data types such as int, float, double, char, etc., of any particular type. To add to it, an array in C/C++ can store derived data types such as the structures, pointers etc.
Given below is the picture representation of an array.
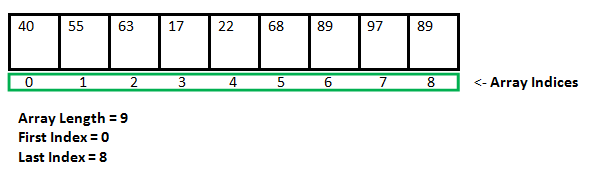
Syntax:
Single -Dimensional Array: The ArraySize must be integer constant greater than Zero and type can be any valid C Data Type.
type ArrayName [ ArraySize ];
double balance [10];
Example:
#include <iostream>
#include <string>
using namespace std;
int main() {
string cars[4] = {"Volvo", "BMW", "Ford", "Ferrari"};
cout <<"Output of Cars[2] array is " <<" " << cars[2];
return 0;
}
Output:

Loop through Array
You can loop through the array elements with the for loop.
Example:
#include <iostream>
#include <string>
using namespace std;
int main() {
string Fruits[4] = {"Apple", "Mango", "Banana", "Orange"};
for(int i = 0; i < 4; i++)
{
cout << Fruits[i] << "\n";
}
return 0;
}
Output:

Advantages of an Array:
Random access of elements using array index.
Use of less line of code as it creates a single array of multiple elements.
Easy access to all the elements.
Traversal through the array becomes easy using a single loop.
Sorting becomes easy as it can be accomplished by writing less line of code.
Disadvantages of an Array:
Allows a fixed number of elements to be entered which is decided at the time of declaration. Unlike a linked list, an array in C is not dynamic.
Insertion and deletion of elements can be costly since the elements are needed to be managed in accordance with the new memory allocation.
The Tech Platform
Comments