Array Destructuring in JavaScript
- The Tech Platform
- Jul 27, 2021
- 6 min read
Updated: Jun 9, 2023
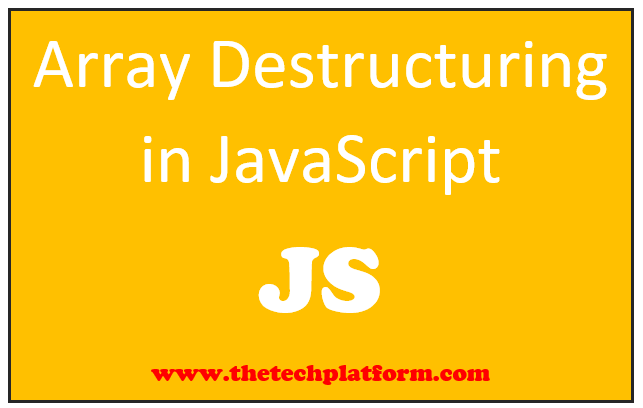
Destructuring is an ECMAScript 6 (ES6/ECMAScript 2015) feature. When it comes to arrays, destructuring is basically a way to unpack values from an array into separate variables. In other words, destructuring is all about breaking a complex data structure (an array in this case) down into a smaller (and therefore simpler) data structure, like a variable.
For arrays, the primary benefit of destructuring is that we can retrieve elements from the array and store them into variables in a very easy way. Let’s start with a very simple array and use destructuring to extract elements from it.
Extracting Elements
Let's explore how destructuring works with arrays using the following example:
let arr = ['Soumadri', 'Sunil', 'John'];
// Traditional way of retrieving elements from an arraylet name1 = arr[0];
let name2 = arr[1];
let name3 = arr[2];
console.log(name1, name2, name3);
In the above code, we extract each element from the arr array using traditional indexing and assign them to separate variables (name1, name2, and name3).
Now, let's achieve the same result using restructuring:
let [name1, name2, name3] = arr;
console.log(name1, name2, name3);
By using destructuring, we declare all three variables (name1, name2, and name3) within square brackets [ ] on the left-hand side of the assignment. JavaScript recognizes this syntax as destructuring and assigns the corresponding elements from the arr array to the variables.
The output of the above code will be the same as before, as it retrieves the elements in the same order.
Furthermore, when we check the original arr array, we can see that it remains unaffected:
console.log(arr);
The output will display the original arr array as it was defined initially.
Destructuring simplifies the process of extracting values from an array, reducing the amount of code required and improving readability. It is a powerful feature in JavaScript that can also be used with objects to extract properties.
Extracting Selected Elements
We also do not have to take all of the elements out of the array. Let’s check out an example where we perform destructuring to retrieve selected elements from an array. We’ll be using the arr array once again.
let arr = ['Soumadri', 'Sunil', 'John'];
let [name1, , name2] = arr;
console.log(name1, name2);
In the above code, we perform destructuring to retrieve selected elements from the arr array. By using empty commas (,,) between name1 and name2, we indicate that the element at the corresponding index position should be skipped.
In this example, name1 will retrieve the element at the 0th index ("Soumadri"), and name2 will retrieve the element at the 2nd index ("John"), while the element in between at the 1st index position ("Sunil") is skipped.
When we run the code and check the result using console.log(name1, name2), we will indeed see "Soumadri" and "John" as expected.
Swapping Variables
Let’s see how we can swap variables using destructuring. Let’s declare two variables name1 and name2, and assign them some values.
let name1 = 'Sunil';
let name2 = 'Soumadri';
// Traditional way of swapping variables
let tmp = name1;
name1 = name2;
name2 = tmp;
console.log(name1, name2);
// Swapping variables using destructuring
let name3 = 'Sunil';
let name4 = 'Soumadri';
[name4, name3] = [name3, name4];
console.log(name3, name4);
In the above code, we demonstrate how to swap variables using destructuring. Initially, we have two variables name1 and name2 with values 'Sunil' and 'Soumadri' respectively. The traditional way of swapping involves using a temporary variable tmp.
After that, we showcase how to swap variables using destructuring. We create two additional variables name3 and name4 with the same values. By assigning [name3, name4] to [name4, name3], the values are swapped in a single line.
When we run the code and check the result using console.log(name1, name2) and console.log(name3, name4), we will observe that the values of name1 and name2 are swapped using the traditional method, while the values of name3 and name4 are swapped using destructuring.
Destructuring Array Values from an Object
First things first, let us create our library object.
const library = {
name: "Soumadri’s Fiction Book Shelf",
location: '1234 Fiction Palace, Calcutta, India',
genres: [
'Mystery',
'Horror',
'Fantasy',
'Sci-Fi',
'Literary Fiction',
'Historical Fiction',
],
titles: [
'The Inugami Clan',
'The Three Coffins',
'In Search of Lost Time',
'Sandman',
'The Left Hand of Darkness',
'The Complete Works of H.P. Lovecraft',
'Do Androids Dream of Electric Sheep?',
'In the Woods',
'In a Glass Darkly',
'Fingersmith',
],
authors: [
'Neil Gaiman',
'Ursula Le Guin',
'Sheridan Le Fanu',
'H.P. Lovecraft',
'Marcel Proust',
'Tana French',
'John Dickson Carr',
'Seishi Yokomizo',
'Philip K. Dick',
'Sarah Waters',
],
};
let [firstBook, secondBook] = library.titles; console.log(firstBook, secondBook);
let [, , secondPickedBook] = library.titles; console.log(secondPickedBook);
[firstBook, , secondBook] = library.titles; console.log(firstBook, secondBook);
[firstBook, secondBook] = [secondBook, firstBook]; console.log(firstBook, secondBook);
In the above code, we demonstrate various examples of destructuring array values from the library object.
First, we extract the first and second elements (book titles) from the library.titles array using destructuring and store them in the variables firstBook and secondBook. We then log the result to the console.
Next, we skip the first two elements of the library.titles array using empty slots in the destructuring pattern, and extract the third element (book title) into the variable secondPickedBook. We log the result to the console.
Then, we extract the first and third elements from the library.titles array using destructuring and skip the second element. The first title is stored in firstBook and the third title is stored in secondBook. We log the result to the console.
Finally, we demonstrate swapping the values of firstBook and secondBook using destructuring. We first extract the values into the variables firstBook and secondBook. Then, by assigning [secondBook, firstBook] to [firstBook, secondBook], the values are swapped. We log the result to the console.
When you run the code, you will see the output for each step of the destructuring operations.
Extracting Elements from an Array Returned by a Function
const library = {
name: "Soumadri’s Fiction Book Shelf",
location: '1234 Fiction Palace, Calcutta, India',
genres: [
'Mystery',
'Horror',
'Fantasy',
'Sci-Fi',
'Literary Fiction',
'Historical Fiction',
],
titles: [
'The Inugami Clan',
'The Three Coffins',
'In Search of Lost Time',
'Sandman',
'The Left Hand of Darkness',
'The Complete Works of H.P. Lovecraft',
'Do Androids Dream of Electric Sheep?',
'In the Woods',
'In a Glass Darkly',
'Fingersmith',
],
authors: [
'Neil Gaiman',
'Ursula Le Guin',
'Sheridan Le Fanu',
'H.P. Lovecraft',
'Marcel Proust',
'Tana French',
'John Dickson Carr',
'Seishi Yokomizo',
'Philip K. Dick',
'Sarah Waters',
],
lend: function(firstBookIndex, secondBookIndex) {
return [this.titles[firstBookIndex], this.titles[secondBookIndex]];
},
};
console.log(library.lend(2, 6));
let [firstBook, secondBook] = library.lend(2, 6);
console.log(firstBook, secondBook);
In the code above, we added the lend function to the library object. This function accepts two parameters: firstBookIndex and secondBookIndex, which represent the index positions of the books to be borrowed from the library.titles array. The function returns an array containing the titles at the specified positions.
To borrow the books, we call the lend function and pass the desired index positions as arguments. The result, which is an array of the selected book titles, is logged to the console.
Next, we use array destructuring to assign the elements of the returned array to the variables firstBook and secondBook. Finally, we log the values of these variables to the console.
When you run the code, you will see the borrowed book titles logged as output.
Nested Destructuring
const nested = [1, 3, [2, 4]];
const [i, , j] = nested;
console.log(i, j);
const [i, , [j, k]] = nested;
console.log(i, j, k);
In the code above, we have a nested array called nested containing elements [1, 3, [2, 4]].
To extract specific values from the nested array, we use array destructuring. In the first example, we extract the values at the 0th index and the 2nd index, skipping the value at the 1st index. This is achieved by using an empty slot (,) to represent the value we want to skip.
In the second example, we further destructure the nested array [2, 4] inside nested. We extract the value at the 0th index of nested into i, skip the value at the 1st index, and then destructure the nested array to extract its values at the 0th and 1st indices into j and k, respectively.
When you run the code, you will see the extracted values logged to the console.
Setting Default Values
const [p, q, r] = [8, 9];
console.log(p, q, r);
const [p = 1, q = 1, r = 1] = [8, 9];
console.log(p, q, r);
In the code above, we have an array [8, 9] assigned to the variables [p, q, r] using array restructuring.
When we log the values of p, q, and r without setting default values, we get 8, 9, and undefined, respectively. This is because the array only contains two elements, but we are trying to assign three variables.
To set default values, we modify the destructuring assignment by using the syntax variable = defaultValue. In the second example, we set the default values for p, q, and r to 1. Now, even though the array [8, 9] has only two elements, the variables p and q will take the values 8 and 9, respectively, while the variable r will take the default value of 1.
When you run the code, you will see the values of p, q, and r logged to the console.
댓글