
In C#, arguments can be passed to parameters either by value or by reference. Passing by reference enables function members, methods, properties, indexers, operators, and constructors to change the value of the parameters and have that change persist in the calling environment.
To pass a parameter by reference with the intent of changing the value, use the ref, or out keyword. To pass by reference with the intent of avoiding copying but not changing the value, use the in modifier. For simplicity, only the ref keyword is used in the examples in this topic.
Below is the difference between Pass by Value and Pass by Reference
Pass by Value Pass by Reference
Passes and argument by Value | Passes an argument by reference |
Callee does not have any access to the underlying element in the calling code | Callee gives a direct reference to the programming element in the calling code |
A copy of the data is sent to the callee | The memory address of the stored data is passed |
Changes made to the passed variable do not affect the actual value | Changes to the value have an effect on original data. |
If we are building multi-threaded application, then we don’t have to worry of objects getting modified by other threads. In distributed application pass by value can save the over network overhead to keep the objects in sync. | In pass by reference, no new copy of the variable is made, so overhead of copying is saved. This makes programs efficient especially when passing objects of large structs or classes. |
Required more Memory | Required less Memory |
Requires more time as it involves copying values | Requires less amount of time as there is no copying. |
Pass by Value
In c#, Passing a Value-Type parameter to a method by value means passing a copy of the variable to the method. So the changes made to the parameter inside the called method will not affect the original data stored in the argument variable.
Passing Parameters By Value Example
Following is the example of passing a value type parameter to a method by value in the c# programming language.
using System;
namespace Tutlane
{
class Program
{
static void Main(string[] args)
{
int x = 10;
Console.WriteLine("Variable Value Before Calling the Method: {0}", x);
Multiplication(x);
Console.WriteLine("Variable Value After Calling the Method: {0}", x);
Console.WriteLine("Press Enter Key to Exit..");
Console.ReadLine();
}
public static void Multiplication(int a)
{
a *= a;
Console.WriteLine("Variable Value Inside the Method: {0}", a);
}
}
}
If you observe the above example, the variable x is a value type, and it is passed to the Multiplication method. The content of variable x copied to the parameter a and made required modifications in the Multiplication method. Still, the changes made inside of the method do not affect the original value of the variable.
Output
When we execute the above c# program, we will get the result as shown below.
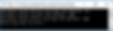
If you observe the above result, the variable value not changed even after we made the modifications in our method.
This is how we can pass parameters to the method by value in the c# programming language based on our requirements.
Pass by Reference
Following is a simple example of passing parameters by reference in the c# programming language.
int x = 10; // Variable need to be initialized
Multiplication(ref x);
If you observe the above declaration, we declared and assigned a value to the variable x before passing it as an argument to the method by using reference (ref).
To use the ref parameter in the c# application, both the method definition and the calling method must explicitly use the ref keyword.
Passing Parameters By Reference Example
Following is the example of passing a value type parameter to a method by reference in the c# programming language.
using System;
namespace Tutlane
{
class Program
{
static void Main(string[] args)
{
int x = 10;
Console.WriteLine("Variable Value Before Calling the Method: {0}", x);
Multiplication(ref x);
Console.WriteLine("Variable Value After Calling the Method: {0}", x);
Console.WriteLine("Press Enter Key to Exit..");
Console.ReadLine();
}
public static void Multiplication(ref int a)
{
a *= a;
Console.WriteLine("Variable Value Inside the Method: {0}", a);
}
}
}
If you observe the above example, we are passing the reference of variable x to variable a in the Multiplication method by using the ref keyword. In this case, the variable contains the reference of variable x, so the changes made to variable a will affect variable x.
Output
When we execute the above c# program, we will get the result as shown below.
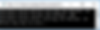
If you observe the above result, the changes we did for the variable in the called method have also reflected the calling method.
This is how we can pass parameters to the method by reference using the ref keyword in c# programming language based on our requirements.
Source Code: Tutlane.com
The Tech Platform