Aphorisms from Zen of Python
Guido van Rossum (creator of python’s language) created a well known Easter Egg in Python called import this that when added to your code will output:
Beautiful is better than ugly.
Explicit is better than implicit.
Simple is better than complex.
Complex is better than complicated.
Flat is better than nested.
Sparse is better than dense.
Readability counts.
Special cases aren't special enough to break the rules.
Although practicality beats purity.
Errors should never pass silently.
Unless explicitly silenced.
In the face of ambiguity, refuse the temptation to guess.
There should be one-- and preferably only one --obvious way to do it.
Although that way may not be obvious at first unless you're Dutch.
Now is better than never.
Although never is often better than *right* now.
If the implementation is hard to explain, it's a bad idea.
If the implementation is easy to explain, it may be a good idea.
Namespaces are one honking great idea -- let's do more of those!
1. Beautiful is better than ugly
We have to talk about this, you don’t have to show anyone else these perfect matches of codes.
You can make a simple program but, even then, the code you write will almost never be exactly like someone else’s. You don’t have to be fancy. C’mon guys, we have to make simple codes easy to understand.
Write for humans and be consistent
Use simple expression syntax
See also: DRY - Don't repeat yourself
https://pt.wikipedia.org/wiki/Don%27t_repeat_yourselfAnd KISS: Keep it simple stupid
https://pt.wikipedia.org/wiki/Princ%C3%ADpio_KISS
2. Explicit is better than implicit
Programming with more explicitness helps us make sense of abstract ideas. It means not to hide the behavior of a function. Specifically, implicit or explicit methods are defined by the contexts in which they are meant to be used. Implicit code can be a very nice experience if things are well named as it keeps things simple.
Specifically the file, the arguments, the variables:
#Implicit
def read(filename):
# code for reading a csv or json
# depending on the file extension
#Explicit
def read_csv(filename):
# code for reading a csv
def read_json(filename):
# code for reading a json
3. Simple is better than complex
Simplifying the code is an art, because we programmers like to see the code running, and at just at the final tests we are trying to simplify, but we can start the project thinking things like: ‘What’s the easiest way to accomplish this action?’
Simplify everything:
x , i = [], 0
while 1:
if i>10:
break
else:
i = i + 1
x.append(i)
4. Complex is better than complicated
Complex things ins’t for just those Nerds, Masters in Math’s. In Python we can do complex things in a ‘Pythonic Way’:




As we can see above, we don’t need to do these full books of calculating, we have to understand their concepts and know how to use it.
5. Flat is better than nested
Flatting the code, new name for a simple action: no need to use long names, long terms, complex functions…
Your code is unique and have to use the same style always:
#dict to organize the code
x = {"name": ('person','full name'),
"contact":{"phones":('12345','67890'),
"email":'belex@pet.com'}}
to
x = {"name": 'person', "fullname":'full name',
"phones":'12345', "cel":'67890',
"email":'belex@pet.com'}
6. Sparse is better than dense
Here you need to use the Unix philosophy that emphasizes building simple, short, clear, modular, and extensible code that can be easily maintained and repurposed by developers other than its creators.
We will talk about this next:
if i > 0:
return sqrt(i)
elif i == 0:
return 0
else:
return 1j * sqrt(-i)
versus
if i>0: return sqrt(i)
elif i==0: return 0
else: return 1j * sqrt(-i)
7. Readability counts
Here I need to emphasize: code for humans, you need to keep these thinks in mind:
Whitespaces for block structures
Minimal Ponctuation
Built in documentation
#complex to understand
[i**2 for i in [j for j in range(10) if j%2==0]]
versus
sequence = range(10)
pairs = [j for j in sequence if j%2==0]
[i**2 for i in pairs]
del sequence, pairs
# cleaning the house!
8. Special cases aren’t special enough to break the rules
Now your code has a style. A style guide is about consistency. Consistency with this style guide is important.
However, know when to be inconsistent — sometimes style guide recommendations just aren’t applicable. Knows when to differ methods and functions by scope.
Everything is an object:
x = [ 4, 8, 15, 16, 23, "42" ]
r = 0
for i in x:
if isinstance(i, basestring):
i = int(i)
r += i
versus
for i in x:
try:
r += i
except TypeError:
r += int(i)
9. Errors should never pass silently
So, you don’t need to be perfect.
If is necessary:
x = [ 4, 8, 15, 16, 23, "42" ]
r = sum(int(i) for i in x)
r
10. Errors should neverpass silently
When you start to do complex programs, you need to start thinking what the user can do to break your application…
Start using the simplest methods like Try, Except.
Exception-based
Error handling
Tracebacks aid
Debugging
try:
# do something
except:
pass
versus
try:
# do something
except:
"Error"
11. Unless explicitly silenced
Your homework is simple: if the user put the wrong command you need to Catch exceptions. Use tracebacks, logging, etc.
After this, follow this pipeline:
Process
Convert
Ignore
Catching Exceptiondef f(n):
if not isinstance(n, int):
raise TypeError(n should be an int)
if n > 0:
return n * f(n-1)
return 1
try:
print f(5)
except TypeError, err:
print 'Had an error:', err
else:
print 'No error'
finally:
print 'Always run'
-----------------
$ python catching.py
Had an error: n should be an int
Always run
12. In the face of ambiguity, refuse the temptation to guess
Your user is so dumb, as we know… coerce types only when not surprising, you cannot add strings and numbers.
Can multiply them!
Type Coercion
print 1 + '1'
print 5 * 1.0
print 2 ** 100
print 2 * abc
print 2 + abc
Traceback (most recent call last):
File "<stdin>", line 1, in ?
TypeError: unsupported operand type(s) for +: 'int' and 'str'
13. There should be one — and preferably only one — way to do it
Don’t need to reinvent the wheel.
Use existing libraries. (pandas is awesome)
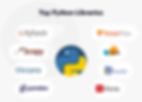
14. Although that way may not be obvious at first unless you’re Dutch
By the way, it is just a joke with his friend: Tim Peters, because Guido van Rossum is Dutch
15. Now is better than never
Here we can see within a various aspects, in the vision of the now is the best moment because in reality there’s no tomorrow, we are always in the now.
But without this coach’s mindset we can see in programming the conversion between another languages like: Jython (Is an implementation of the Python programming language designed to run on the Java platform.) which means that you don’t need to be the Java’s expert to use Java in your own applications.
15. If the implementation is hard to explain, it’s a bad idea
If you can’t explain it simply, you don’t understand it well like Einstein’s said. Enough’ If the implementation is easy to explain, it may be a good idea.
16. Namespaces are one honking great idea — let’s do more of those!
And finally, he is referencing the modules in python, a simple implementation is: Import.
A module can contain executable statements as well as function definitions.
The basic import statement (no from clause) is executed in two steps:
find a module, loading and initializing it if necessary.
define a name or names in the local namespace for the scope where the import statement occurs.from foo import bar’ instead of ‘from foo import *’
Source: Medium - Pedro Felix
The Tech Platform