"this" Parameter in JavaScript
- The Tech Platform
- Jun 3, 2022
- 4 min read
The "this" parameter in JavaScript is a fundamental concept in JavaScript that plays an important role in defining the context of a function and accessing object properties. It allows functions to interact with the object they belong to and provides a mechanism for dynamic binding. Understanding the usage and purpose of "this" in JavaScript is important for writing clean, maintainable code and leveraging the full power of JavaScript's object-oriented capabilities.
In this article, we will delve into the definition, purpose, and usage of "this" in JavaScript. We will explore how it grants access to other properties on the same object, how it depends on the invocation context rather than the function's definition, and how it behaves differently when used with arrow functions.
What is 'this' in JavaScript?
The "this" parameter in JavaScript refers to an object that is associated with the function invocation. It is an implicit parameter that is automatically passed to a function when it is called. The value of the "this" parameter determines the context in which the function is executed.
The behavior of the "this" parameter is influenced by two factors: where the function is defined and how the function is invoked.
Where the function is defined:
If a function is defined as a method of an object, "this" refers to the object itself. Inside the function, you can access the object's properties and methods using "this".
If a function is defined globally (not as a method of any object), "this" refers to the global object. In a web browser, the global object is usually the window object.
How the function is invoked:
If a function is called as a method of an object using the dot notation (e.g., obj.method()), "this" refers to the object that owns the method. It allows the function to access the object's properties and methods.
If a function is called using the "new" keyword to create an instance of an object (e.g., new Constructor()), "this" refers to the newly created object. It allows the constructor function to initialize the properties of the new object.
If a function is called using the call(), apply(), or bind() methods, "this" is explicitly set to the value provided as the first argument to these methods. It allows you to control the value of "this" within the function.
Understanding the value of the "this" parameter is crucial for working with object-oriented programming in JavaScript and for accessing the correct object context within a function. It allows functions to be reused in different contexts and enhances the flexibility and reusability of code.
By utilizing the "this" parameter effectively, you can access and manipulate the properties and methods of objects dynamically, based on the context in which the function is invoked.
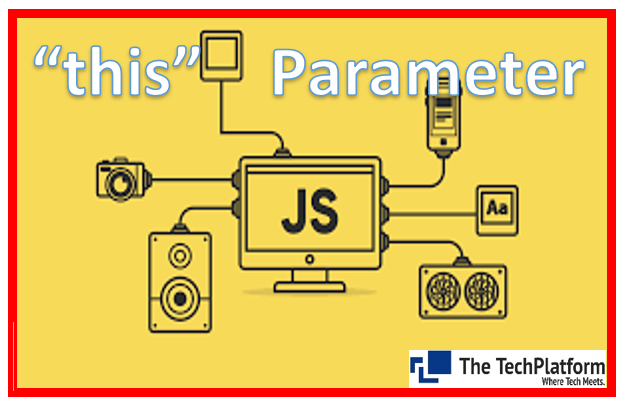
Usage and Purpose of "this" in JavaScript
The "this" parameter in JavaScript plays a crucial role in accessing and manipulating object properties and defining the context of a function. Let's explore its usage and purpose in detail:
It gives access to other properties on the same object:
When a function is defined as a method on an object, the "this" keyword inside that function refers to the object itself. It allows the function to access other members (properties or methods) on the same object. This behavior enables better code organization and encapsulation. For example:
const obj = {
msg: 'Hi',
logMessage: function() {
console.log(this.msg);
}
};
obj.logMessage(); // Output: 'Hi'
It depends on how the function is invoked, not where it is defined:
The value of the "this" parameter is determined by how a function is invoked, regardless of where the function is defined. When a method is called on an object, "this" refers to that object. However, when the same function is invoked with different objects, "this" points to the respective object. For example:
const obj = {
msg: 'Hi',
logMessage: function() {
console.log(this.msg);
}
};
const newObj = {
msg: 'Hello',
logMessage: obj.logMessage
};
obj.logMessage(); // Output: 'Hi'
newObj.logMessage(); // Output: 'Hello'
It points to something else when using the function form:
Functions that are not part of an object can be invoked independently. In such cases, the value of "this" inside the function depends on how the function is invoked. If the function is invoked as a standalone function, "this" may point to the global object (e.g., window in a web browser) or be undefined in strict mode. This behavior can lead to unexpected results. For example:
const obj = {
msg: 'Hi',
logMessage: function() {
console.log(this.msg);
}
};
const logMessage = obj.logMessage;
logMessage(); // Output: undefined
Arrow functions don't have their own "this":
Arrow functions in JavaScript don't have their own "this" binding. Instead, they inherit the "this" value from the surrounding lexical scope. This behavior is beneficial when dealing with nested functions and avoids the need for additional workarounds. For example:
const obj = {
logMessage: function() {
const msg = 'Hi';
const logSomething = () => {
console.log(msg);
};
logSomething();
}
};
obj.logMessage(); // Output: 'Hi'
Applications can be written without using "this":
While "this" is a fundamental concept in JavaScript, it is not mandatory to use it in application development. Modern UI frameworks like React Hooks, Vue.js Composition API, and Svelte allow writing components without using "this". Encapsulated objects can be achieved using closures, ensuring access to variables even after the outer function has returned. This approach provides more control and flexibility over the application structure. For example:
const obj = (function() {
let msg = '';
function logMessage() {
msg = 'Hi';
const logSomething = () => {
console.log(msg);
};
logSomething();
}
return {
logMessage
};
})();
obj.logMessage(); // Output: 'Hi'
Understanding and mastering the usage of the "this" parameter in JavaScript is essential for effectively working with objects, defining proper function contexts, and writing maintainable code.
That's It!
Comentarios