PHP Array: Create, Access, and Manipulate.
- The Tech Platform
- Aug 12, 2023
- 9 min read
Arrays, those essential tools in the programming world, empower developers to streamline data management. These adaptable containers let you neatly arrange a collection of values under a single name. Across programming languages, arrays are the bedrock, for efficiently managing data. In PHP, an array plays a pivotal role, giving developers potent ways to wield data.
From basic lists to intricate data setups, a PHP array opens up a treasure trove of options for data handling and manipulation. This article delves into PHP arrays, exploring their structure, types, features, and real-world applications. Whether you're starting out or a seasoned pro, this guide equips you with a deep understanding of PHP arrays, enabling you to make the most of their power. So, let's embark on this journey and unveil the enchanting world of PHP arrays together.
Table of Content
Using [] Operator
Using key() and value() Function
Using each() Function
Adding an element in an array
Removing an element from an array
Sort an array
Search an array
6. Conclusion
What is PHP Array?
A PHP Array is a data structure that can store multiple values of the same or different data types. Arrays are indexed, which means that each element in the array has a unique identifier called the index. The index can be a number or a string.
There are three types of arrays in PHP:
1. Indexed arrays: Indexed arrays are the most common type of array in PHP. The elements in an indexed array are stored in consecutive memory locations, and the index is a number that starts from 0.
Here is an example of an indexed PHP Array:
$cars = array("Volvo", "BMW", "Toyota");
This array has 3 elements, and the elements are "Volvo", "BMW", and "Toyota". The indexes of the elements are 0, 1, and 2.
2. Associative arrays: Associative arrays are arrays that use keys to store the elements. The keys can be strings or numbers.
Here is an example of an associative PHP array
$fruits = array("apple" => "red", "banana" => "yellow", "orange" => "orange");
This array has 3 elements, and the keys are "apple", "banana", and "orange". The values of the elements are "red", "yellow", and "orange".
3. Multidimensional arrays: Multidimensional arrays are arrays that can contain other arrays. This allows you to store data in a hierarchical fashion.
Here is an example of a multidimensional PHP Array:
$cars = array(
array("Volvo", 2019),
array("BMW", 2020),
array("Toyota", 2021)
);
This array has 3 elements, and each element is an array. The first element is an array with the elements "Volvo" and 2019. The second element is an array with the elements "BMW" and 2020. The third element is an array with the elements "Toyota" and 2021.
Creating arrays in PHP
In PHP, there are a few different ways to create an array. Here are a couple of common methods:
Using the Array() Function: You can create an array using the array() function. Here's the basic syntax:
$array_name = array(element1, element2, ..., elementN);
For example:
$fruits = array("apple", "banana", "orange");
Using Square Brackets (Short Array Syntax, PHP 5.4+): Starting from PHP 5.4, you can also use square brackets to create an array:
$array_name = [element1, element2, ..., elementN];
For example:
$fruits = ["apple", "banana", "orange"];
Create an Indexed PHP Array
<?php
// Creating an indexed array
$fruits = array("apple", "banana", "orange", "grape");
// Accessing array elements
echo "I like " . $fruits[0] . " and " . $fruits[1] . ".";
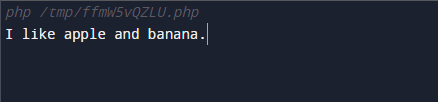
Create an associate PHP Array
<?php
// Creating an associative array
$person = array(
"name" => "John",
"age" => 30,
"city" => "New York"
);
// Accessing associative array elements
echo "My name is " . $person["name"] . ", I am " . $person["age"] . " years old.";

Create Multidimensional Arrays
>?php
// Creating a multidimensional array
$matrix = array(
array(1, 2, 3),
array(4, 5, 6),
array(7, 8, 9)
);
// Accessing multidimensional array elements
echo "The value at matrix[1][2] is " . $matrix[1][2] . ".";
?>
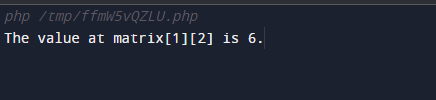
Accessing Array elements in PHP
There are three ways to access elements of the PHP array:
1. Using the [] operator:
The [] operator is the most common way to access array elements. It takes the index of the element as its argument, and it returns the value of the element. For example, the following code gets the value of the first element in the $cars array:
$firstCar = $cars[0];
Consider the below example, we have created an array named $colors with five elements. We use the [] operator to access elements by their index within square brackets.
<?php
// Creating an array
$colors = array("red", "green", "blue", "yellow", "purple");
// Accessing array elements using []
echo"Color at index 0: " . $colors[0] . "<br>";
echo"Color at index 2: " . $colors[2] . "<br>";
echo"Color at index 4: " . $colors[4] . "<br>";
?>
2. Using the key() and value() functions:
The key() and current() functions in PHP are used to retrieve the key and value of the current element in an array, respectively.
Here's an example of how you can use these functions to access array elements:
<?php
// Creating an associative array
$student_scores = array(
"Alice" => 85,
"Bob" => 92,
"Charlie" => 78,
"David" => 95,
"Eve" => 88
);
// Resetting the internal pointer of the array to the first element
reset($student_scores);
// Accessing array elements using key() and current() functions
while ($key = key($student_scores)) {
$value = current($student_scores);
echo "$key has scored $value marks.";
// Move the internal pointer to the next element
next($student_scores);
}
?>
In this example, we've created an associative array $student_scores with students' names as keys and their scores as values. We then reset the internal pointer of the array using reset() to ensure we start iterating from the beginning.
Inside the while loop, we use the key() function to retrieve the current key (student's name) and the current() function to retrieve the current value (student's score). We then print out a message displaying the student's name and their score. After each iteration, we use the next() function to move the internal pointer to the next element.
3. Using the each() function:
The each() function in PHP is used to retrieve the current key and value pair from an array and then advance the internal array pointer. However, it's important to note that each() function is considered deprecated as of PHP 7.2.0 and has been removed in PHP 8.0.0. It's recommended to use alternatives like foreach loops for iterating through arrays.
Here, I will provide an example of how you could use each() function:
<?php
// Creating an array
$colors = array("red", "green", "blue", "yellow");
// Using the each() function to access array elements
$element = each($colors);
echo "Key: " . $element['key'] . ", Value: " . $element['value'] . "\n";
$element = each($colors);
echo "Key: " . $element['key'] . ", Value: " . $element['value'] . "\n";
?>
In this example, we've used each() function to access array elements. The function returns an array containing the 'key' and 'value' of the current element and then advances the array's internal pointer to the next element. However, as mentioned earlier, it's recommended to use foreach loops for iterating through arrays, especially since each() function is deprecated.
Manipulating arrays in PHP
There are many ways to manipulate arrays in PHP. Here are a few common examples:
Adding elements to an array:
You can use the array_push() function to add elements to the end of an array. The array_push() function takes an array as its first argument, and it takes a comma-separated list of elements as its second argument. The function adds the elements to the end of the array and returns the number of elements in the array.
For example, the following code adds the element "grape" to the end of the $fruits array:
<?php
// Creating an initial fruits array
$fruits = array("apple", "banana", "orange");
// Adding a new element to the array using array_push()
array_push($fruits, "grape");
// Printing the updated array
print_r($fruits);
?>
Removing elements from an array:
You can use the unset() function to remove elements from an array. The unset() function takes the index of the element as its argument.
For example, the following code removes the first element from the $fruits array:
<?php
// Creating an initial array
$fruits = array("apple", "banana", "orange", "grape");
// Removing an element using unset()
unset($fruits[2]);
// This removes the "orange" element// Printing the updated array
print_r($fruits);
?>
Sorting arrays:
You can use the sort() function to sort an array in ascending order. The sort() function takes an array as its argument and returns the sorted array.
For example, the following code sorts the $fruits array in ascending order:
<?php
// Creating an array of fruits
$fruits = array("banana", "apple", "orange", "grape");
// Sorting the array in alphabetical order
sort($fruits);
// Printing the sorted array
print_r($fruits);
?>
For example, the following code sorts the $numbers array in ascending order:
<?php
// Creating an array
$numbers = array(10, 5, 8, 2, 7);
// Sorting the array using sort()
sort($numbers);
// Printing the sorted array
print_r($numbers);
?>
Searching arrays:
You can use the array_search() function to search for an element in an array. The array_search() function takes the element to search for as its first argument, and it takes the array to search as its second argument. The function returns the index of the element if it is found in the array, or it returns -1 if it is not found.
For example, we have an array of fruits. We use the array_search() function to search for the value "orange" within the array. If the value is found, the function returns the corresponding index (key). If the value is not found, it returns false.
<?php
// Creating an array
$fruits = array("apple", "banana", "orange", "grape");
// Searching for a value using array_search()
$searchValue = "orange";
$result = array_search($searchValue, $fruits);
if ($result !== false)
{
echo "$searchValue found at index: $result";
} else {
echo "$searchValue not found in the array";
}
?>
When you run this script, it will output:
orange found at index: 2
If you were to search for a value that's not in the array, like "pear", the output would be:
pear not found in the array
Common Mistakes People Make when Working with Array in PHP
Here are some common mistakes that people make when working with arrays in PHP:
Assuming that an array key is always set. This is a common mistake, especially when working with associative arrays. If you try to access an element in an associative array with a key that does not exist, PHP will return NULL. To avoid this, you should always check if the key exists before you try to access it.
Assuming that the array's order is defined by the key. This is not always the case, especially when you are using a multidimensional array. The order of the elements in a multidimensional array is not guaranteed, and it can change depending on how the array is created. To avoid this, you should not rely on the order of the elements in a multidimensional array.
Not using strict comparison with current() and next(). The current() and next() functions are used to iterate through an array. By default, they use loose comparison, which means that they will return the next element in the array even if the key is not strictly equal. To avoid this, you should use the strict comparison operator (===) with current() and next().
Using numeric strings, floats, or Booleans for array keys. Numeric strings, floats, and Booleans are not valid array keys in PHP. If you try to use one of these values as an array key, PHP will convert it to an integer. This can lead to unexpected results, so it is best to avoid using these values as array keys.
Using strict comparison on numeric string keys in foreach loops. When using a foreach loop to iterate through an array with numeric string keys, you should use the loose comparison operator (==) instead of the strict comparison operator (===). This is because the keys will be converted to integers by PHP, and the strict comparison operator will only return true if the keys are equal after they have been converted to integers.
Using arrays instead of iterables for ordered lists. Arrays are not always the best way to store ordered lists. If you are only going to be using the list to iterate through it, you can use an iterable object such as a SplFixedArray or a SplDoublyLinkedList. This will be more efficient than using an array because it will not have to create a copy of the list each time it is iterated through.
Assuming that keys are rearranged when an array element is removed. This is not always the case. When you remove an element from an array, the keys of the remaining elements are not automatically rearranged. This can lead to unexpected results, so it is best to rearrange the keys yourself if you need to.
Using references in foreach loops to change the array's values. This can lead to unexpected results because the changes will be reflected in the original array. If you need to change the values of an array in a foreach loop, you should use a copy of the array.
Using isset() instead of array_key_exists(). The isset() function can be used to check if a variable is set. However, it cannot be used to check if a key exists in an array. To check if a key exists in an array, you should use the array_key_exists() function.
Conclusion
In summary, PHP arrays are invaluable for managing data efficiently. We've covered how to create, access, and manipulate arrays, empowering you to build dynamic applications. But, beware of common mistakes like overusing keywords and mishandling indices. By mastering these techniques and avoiding pitfalls, you're primed to create effective, error-free code that capitalizes on the power of PHP arrays.
תגובות