Model Component in ASP.NET MVC Architecture
- The Tech Platform
- Feb 28, 2023
- 5 min read
ASP.NET MVC (Model-View-Controller) is a powerful framework for building web applications. One of its key components is the Model, which represents the data and business logic in the application. In this article, we will take a closer look at the Model and its role in the ASP.NET MVC architecture.
What is Model Component?
The Model is a component in the ASP.NET MVC framework that represents the data and business logic of an application. It is responsible for managing the application's data and business logic by encapsulating its state and behavior.
The Model provides an abstraction layer between the presentation layer and the data access layer of the application. It handles the business logic of the application, including validation, calculations, and data processing. The Model component ensures that the data is consistent and accurate and that it is stored securely.
The following are the components of MVC:
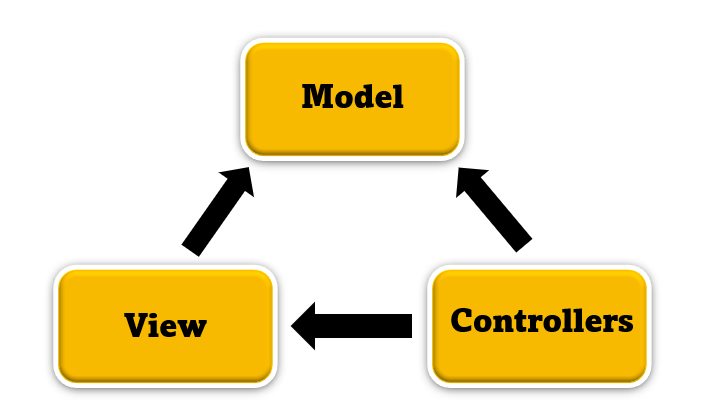
1. Model: The Model component represents the application's data and business logic. It is responsible for retrieving, updating, and deleting data from a database or other data sources. The Model component also defines the rules and validations to be applied to the data.
2. View: The View component represents the user interface of the application. It is responsible for rendering the data provided by the Model component and displaying it to the user. Views are usually implemented using HTML, CSS, and JavaScript.
3. Controller: The Controller component is responsible for receiving user input, processing it, and making the appropriate calls to the Model component to retrieve or update data. Once the data has been retrieved or updated, the Controller selects the appropriate View to display the data to the user.
In ASP.NET MVC, the Model is implemented using a set of classes that define the application's data and its behavior. It provides a clean and consistent interface for other components in the system to interact with. The Model component is designed to be reusable and maintainable, making it easy to manage and update as the application evolves.
Model Flow Diagram
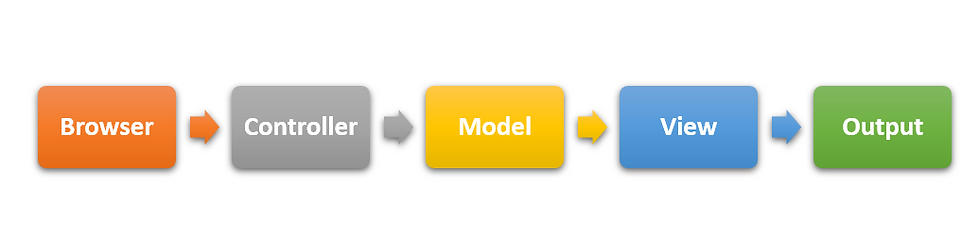
Step 1 − The client browser sends a request to the MVC Application.
Step 2 − Global.ascx receives this request and performs routing based on the URL of the incoming request using the RouteTable, RouteData, UrlRoutingModule, and MvcRouteHandler objects.
Step 3 − This routing operation calls the appropriate controller and executes it using the IControllerFactory object and MvcHandler object's Execute method.
Step 4 − The Controller processes the data using Model and invokes the appropriate method using ControllerActionInvoker object
Step 5 − The processed Model is then passed to the View, which in turn renders the final output.
Implementation of the Model
In ASP.NET MVC, the Model is typically implemented using classes that define the application's data and behavior. These classes can be created using various programming languages, including C# and Visual Basic.
For example, let's say we have an application that stores information about customers. We can create a Customer class that defines the data associated with a customer:
public class Customer
{
public int Id { get; set; }
public string Name { get; set; }
public string Email { get; set; }
public DateTime DateOfBirth { get; set; }
}
This class defines four properties: Id, Name, Email, and DateOfBirth. These properties represent the data associated with a customer.
Examples of data operations performed by the Model
The Model is responsible for performing various data operations, including CRUD operations (create, read, update, and delete), data retrieval, and data processing.
For example, let's say we want to retrieve a list of all customers from our database. We can create a method in our Customer class that retrieves this data:
public List<Customer> GetAllCustomers()
{
List<Customer> customers = new List<Customer>();
// code to retrieve all customers from the database// and add them to the customers listreturn customers;
}
This method retrieves all customers from the database and returns them as a list of Customer objects.
We can also create methods to perform CRUD operations on our data. For example, to create a new customer, we can create a method like this:
public void CreateCustomer(Customer customer)
{
// code to add the customer to the database
}
This method takes a Customer object as a parameter and adds it to the database.
Importance of ensuring data consistency and security
The Model is responsible for ensuring that the application's data is consistent and accurate and that it is stored securely. This is critical for the overall reliability and security of the application.
To ensure data consistency, the Model should implement validation rules that enforce data integrity. For example, if we want to ensure that the email address for a customer is unique, we can add a validation rule to our Customer class:
public class Customer
{
// ...
[Required]
[EmailAddress]
[UniqueEmail]
public string Email { get; set; }
}
public class UniqueEmailAttribute : ValidationAttribute
{
protected override ValidationResult IsValid(object value, ValidationContext validationContext)
{
// code to check if the email address is uniquereturn ValidationResult.Success;
}
}
This code adds a validation rule that ensures the email address for a customer is unique.
To ensure data security, the Model should implement measures to protect sensitive data, such as encrypting passwords and implementing access controls. The Model should also follow best practices for data storage, such as using secure database connections and avoiding storing sensitive data in plain text.
Benefits of Model in ASP.NET MVC
The Model component in ASP.NET MVC offers several benefits that make it an essential part of modern web application development. Some of the benefits of using the Model in ASP.NET MVC include:
Separation of concerns: One of the most significant benefits of using the Model in ASP.NET MVC is the separation of concerns it provides. By separating the data and business logic of the application from the user interface, the Model enables developers to work on each component independently. This separation of concerns makes it easier to maintain and update the application over time.
Code reusability: Another benefit of using the Model in ASP.NET MVC is the code reusability it offers. By encapsulating the application's data and business logic in a single component, the Model makes it easy to reuse code across different parts of the application. This reusability can help reduce development time and improve the overall quality of the code.
Testability: The Model component in ASP.NET MVC is designed to be easily testable. Because the Model encapsulates the application's data and business logic, developers can test this code in isolation, without worrying about the user interface. This testability makes it easier to identify and fix bugs in the code, improving the overall quality and reliability of the application.
Data consistency and security: The Model component is responsible for ensuring the consistency and security of the application's data. By implementing validation rules and security measures, the Model helps ensure that the data is accurate, consistent, and secure. This can help prevent data corruption, data loss, and data breaches, improving the overall reliability and security of the application.
Conclusion
The Model is a crucial component of the ASP.NET MVC architecture. It provides a clean and consistent interface for the application's data and business logic and allows developers to write code that is reusable, maintainable, and testable. By separating the data and business logic from the presentation layer, the Model enables the creation of flexible, scalable, and robust web applications.
Commentaires