How to use PowerShell Reference Variable
- The Tech Platform
- Jul 6, 2023
- 3 min read
In PowerShell, when we pass parameters to functions, we often make use of PowerShell reference variables to enhance flexibility and control over the data being passed.
There are two primary methods for passing variables to functions:
by value
by reference.
When we pass a variable by value, a copy of the data is made and passed to the function. This means that any modifications made to the variable within the function do not affect the original variable outside the function's scope. In other words, the value of the variable remains unchanged once the function execution is completed.
This behavior is planned and ensures that the original value of the variable is preserved, preventing unintended side effects and maintaining data consistency. By passing variables by value, we can control how and when modifications are applied to the original data, providing a level of isolation between the function and the calling code.
By utilizing the concept of passing variables by value, we can confidently work with functions without worrying about unintended changes to our data.
PowerShell Reference Variable
1. Passing Variable to functions by Value
Here's an example of a PowerShell function that takes a parameter as a value type:
Function Calculate($num)
{
$num = 50
}
$var = 100
Calculate -num $var
Write-Host $var
In this case, we have a function called "Calculate" that takes a parameter, $num, as a value type. Inside the function, we assign the value 50 to the $num variable.
When we run the code and check the output, we see that the value of $var remains as 100. This is because passing the variable by value creates a copy of the data, and any modifications made within the function do not affect the original variable outside of the function's scope.
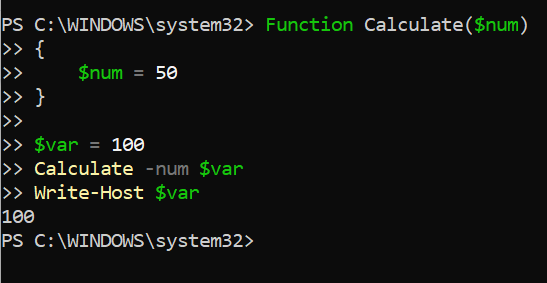
However, if we pass the parameter as a reference type, the value of the variable will be changed.
2. Passing a variable to function by Reference
If we want to change the value of the variable outside the function's scope, we can pass the parameter as a reference type. We can achieve this by using the [ref] keyword. Here's an example:
Function Calculate([ref]$number)
{
$number.Value = 50
}
$var = 100
Calculate -number ([ref]$var)
Write-Host $var
In this case, we are passing $var as a reference type to the "Calculate" function. Within the function, we modify the $number.Value property to 30. When we check the value of $var after running the code, we observe that it has been changed to 30.
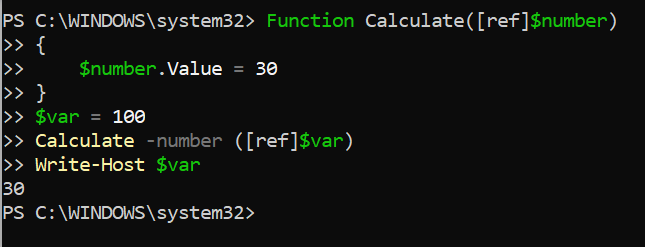
By passing the parameter as a reference type, we allow the function to directly modify the original variable, impacting its value outside the function's scope.
PowerShell Pass by reference multiple parameters
In PowerShell, it is possible to pass multiple parameters by reference, allowing for the modification of multiple variables within a function.
Consider the following example, where we pass two reference type variables to a PowerShell function:
Function GetFullName([ref]$fname, [ref]$lname)
{
$fname.Value = "Bhawana"
$lname.Value = "Rathore"
}
$firstname = "Bijay"
$lastname = "Sahoo"
GetFullName -fname ([ref]$firstname) -lname ([ref]$lastname)
Write-Host $firstname" "$lastname
In the above code, we define the function "GetFullName" that accepts two parameters, $fname, and $lname, both passed as reference types using the [ref] keyword. Within the function, we assign new values to $fname.Value and $lname.Value, changing their content.
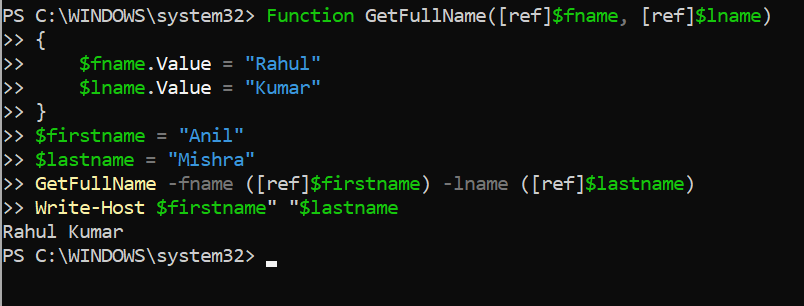
When we execute the script and examine the output, we notice that the values of $firstname and $lastname have been modified according to the function's logic.
A PowerShell reference type is expected in the argument
While working with PowerShell reference variables, you may encounter an error that states: "Cannot process argument transformation on parameter 'number'. Reference type is expected in argument." Let's explore the issue and the solution.
Consider the following code snippet where you encountered the error:
Function Calculate([ref]$number)
{
$number.Value = 50
}
$var = 100
Calculate -number [ref]$var
Write-Host $var
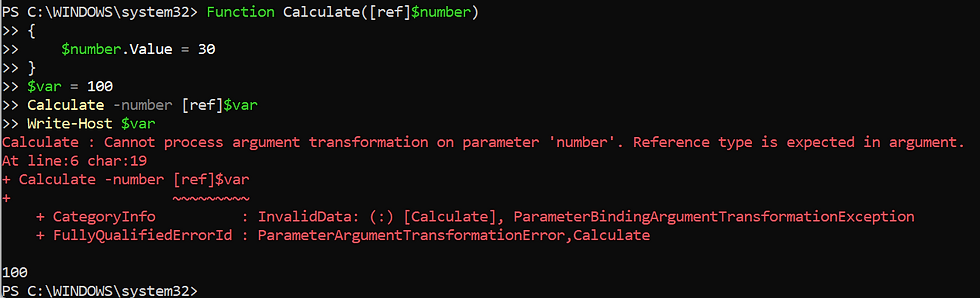
The error occurs because of the way the reference parameter is passed to the function. To resolve this, you need to add the reference type variable name within parentheses (), and within the function, refer to the value using $variable.Value. The correct way to call the function would be:
Calculate -number ([ref]$var)
By making this adjustment, you ensure that the reference variable is properly recognized as a reference-type argument.
Conclusion
This tutorial discussed the concept of PowerShell reference variables, including their creation and usage. It also addressed the issue of passing multiple parameters by reference in PowerShell. Furthermore, we explored the error that occurs when a reference type is expected in an argument and provided the appropriate solution. By understanding these aspects, you can effectively work with reference variables in PowerShell and handle any related errors that may arise.
댓글